Introduction to Control Statements in SQL
As you learn about computer science and programming, you will inevitably encounter databases and SQL, the Structured Query Language. SQL is a widely used database technology that allows you to store, manage, and retrieve data from a database. One important aspect that makes SQL even more powerful is the use of control statements. Control statements in SQL enable you to execute or skip specific code sections based on certain conditions and to modify the order in which statements are executed.
Control Statements in SQL Definition
Control statements in SQL are a set of commands that provide a way to control the flow of a program. They allow you to make decisions and perform specific actions depending on different conditions. This functionality is critical when managing data, as it enables you to create more dynamic and flexible queries.
Types of Control Statements in SQL
There are several types of control statements in SQL, which play a significant role in handling the flow of code execution. These statements are used to handle different cases within your SQL code, allowing for more powerful and versatile database management. Some of the most commonly used control statements in SQL include:
- IF...THEN...ELSE
- WHILE
- FOR
- CASE
- LOOP
For example:
DECLARE @age INT = 25;
IF @age >= 18
BEGIN
PRINT 'You are an adult.'
END
ELSE
BEGIN
PRINT 'You are a minor.'
END
In this example, an IF...THEN...ELSE control statement is used to check if a person is an adult based on their age. If the age is greater than or equal to 18, a message stating "You are an adult" will be displayed. Otherwise, the message "You are a minor" will be shown.
Take a deeper look at some of these control statements:
- IF...THEN...ELSE: This statement allows you to execute different code blocks based on a condition. If the condition is true, then the code within the BEGIN block following THEN is executed. If the condition is false, the code within the BEGIN block after ELSE is executed.
- WHILE: WHILE is a loop control statement that continually executes a block of code as long as a specific condition is true. Once the condition becomes false, the loop will stop, and program execution will continue after the loop.
- FOR: The FOR command is used to loop through a specified range of values in a SELECT statement with a cursor. Like the WHILE statement, FOR also executes a set of statements repeatedly based on a condition.
- CASE: CASE is a versatile statement that allows you to perform conditional logic in SQL queries. It can be used to execute different expressions or code blocks depending on the value of an expression or a set of conditions.
- LOOP: LOOP is a control statement that is used to execute a block of code repeatedly until a specific condition is met. It is often used in conjunction with other control statements, such as IF and WHILE, to create more complex control structures.
By mastering the use of control statements in SQL, you can create more complex and flexible solutions for your data management needs. Understanding how to control the flow of your code execution enables you to harness the full potential of SQL and make the most of your database systems.
Understanding Control Flow Statements in SQL
Control flow statements in SQL are essential tools for managing and working with data in a rational and organised manner. By understanding how these statements function and learning how to implement them effectively, you will be better equipped to harness the power of SQL in your database management tasks.
Conditional Control Statements in SQL Explained
Conditional control statements in SQL provide a way to perform different actions or execute different parts of code based on specific conditions. They bring flexibility to your SQL code, allowing you to handle different scenarios dynamically. Some commonly used conditional control statements in SQL include:
- IF...THEN...ELSE
- CASE
IF...THEN...ELSE: The IF...THEN...ELSE statement evaluates a condition and, based on whether the condition is true or false, subsequently executes one of the two code blocks enclosed within the BEGIN and END statements.
There are two types of IF statements in SQL:
- IF...THEN
- IF...THEN...ELSE
The first type, IF...THEN, is used when you only need to execute a block of code if a certain condition is met. The second type, IF...THEN...ELSE, allows you to define an alternate block of code to be executed if the condition is not met.
Consider the following example:
DECLARE @score INT = 75;
IF @score >= 60
BEGIN
PRINT 'Passed';
END
ELSE
BEGIN
PRINT 'Failed';
END
Here, the IF...THEN...ELSE statement checks whether the @score variable is greater than or equal to 60. If the condition is true, it will print 'Passed', otherwise, it will print 'Failed'.
CASE: The CASE statement is a more versatile conditional control statement that allows you to perform different actions or execute different expressions depending on the value of an expression or a set of conditions. It can be used both in SELECT statements and within stored procedures or functions.
Two forms of the CASE statement in SQL are:
- Simple CASE expression
- Searched CASE expression
Here's an example of a simple CASE expression:
SELECT OrderID,
CASE ShipRegion
WHEN 'North America' THEN 'NA'
WHEN 'South America' THEN 'SA'
WHEN 'Europe' THEN 'EU'
ELSE 'Other'
END as Region
FROM Orders;
In this example, the CASE statement assigns a short code to the ShipRegion column of each row in the Orders table based on the region's name.
Iterative Control Statements in SQL with Example
Iterative control statements in SQL are used to execute a block of code repeatedly, based on a specific condition or set of conditions. These statements provide a way to loop through certain operations, enabling more complex and dynamic data management tasks. Two of the most commonly used iterative control statements in SQL are:
- WHILE
- FOR
WHILE: The WHILE statement continually executes a specified block of code as long as a particular condition is true. Once the condition becomes false, the loop terminates, and the program execution continues after the loop.
Here's an example of a WHILE loop:
DECLARE @counter INT = 1;
WHILE @counter <= 10
BEGIN
PRINT CONCAT('Number: ', @counter);
SET @counter = @counter + 1;
END
In this example, the WHILE loop prints the numbers 1 through 10. The loop continues to iterate as long as the value of @counter is less than or equal to 10.
FOR: The FOR loop in SQL is used to iterate through a specified range of values in a SELECT statement using a cursor. It is a powerful tool for managing data when combined with other SQL commands and control statements. However, FOR loops are less common in SQL than in other programming languages, as SQL primarily deals with set-based operations.
Here's an example of using a FOR loop with a cursor:
DECLARE @ProductName NVARCHAR(50);
DECLARE product_cursor CURSOR FOR
SELECT ProductName
FROM Products
WHERE CategoryID = 2;
OPEN product_cursor;
FETCH NEXT FROM product_cursor INTO @ProductName;
WHILE @@FETCH_STATUS = 0
BEGIN
PRINT @ProductName;
FETCH NEXT FROM product_cursor INTO @ProductName;
END
CLOSE product_cursor;
DEALLOCATE product_cursor;
In this example, the FOR loop iterates through the ProductName column of the Products table for all rows with a CategoryID of 2. The loop prints out each ProductName, using the cursor to manage the looping process.
Taking the time to understand and practice the usage of conditional and iterative control statements in SQL will drastically improve your ability to manage and manipulate data more effectively. Familiarising yourself with these essential tools is key to harnessing SQL's full potential and maximising the efficiency of your database management tasks.
Essential Examples of Control Statements in SQL
Having a strong grasp of real-life examples of control statements in SQL can significantly enhance your ability to work with and manage databases effectively. In this section, we will dive deeper into the practical usage of some of the most important control statements: IF, CASE, WHILE, and LOOP.
Control Statements in SQL: IF Statement
In SQL, the IF statement plays a crucial role in conditionally executing different parts of code based on specific circumstances. Let's explore a few examples that illustrate the practical use of the IF statement and uncover some of its versatile applications.
Example 1: Calculate bonuses for employees based on their performance rating:
DECLARE @PerformanceRating INT = 5;
DECLARE @BonusAmount DECIMAL(7,2);
IF @PerformanceRating >= 4
BEGIN
SET @BonusAmount = 1000.00;
PRINT 'Bonus amount: ' + CAST(@BonusAmount AS NVARCHAR) + ' for excellent performance.';
END
ELSE
BEGIN
SET @BonusAmount = 500.00;
PRINT 'Bonus amount: ' + CAST(@BonusAmount AS NVARCHAR) + ' for satisfactory performance.';
END
In this example, the IF statement checks an employee's performance rating and calculates their bonus amount accordingly. With excellent performance (a rating of 4 or higher), the system will assign a bonus of 1000.00. Otherwise, the bonus will be 500.00.
Control Statements in SQL: CASE Statement
The CASE statement is another highly versatile control statement in SQL that allows you to perform conditional verifications on data and outputs different results based on specific evaluation criteria. Here are a few examples of how the CASE statement can be used effectively:
Example 1: Calculate discounts for customers based on their membership status:
SELECT CustomerID,
MembershipStatus,
TotalSpent,
CASE MembershipStatus
WHEN 'Gold' THEN TotalSpent * 0.10
WHEN 'Silver' THEN TotalSpent * 0.05
ELSE TotalSpent * 0.02
END AS DiscountedAmount
FROM Customers;
This example demonstrates the use of a simple CASE expression. The membership status column from the Customers table is evaluated, and a discount percentage is applied based on the membership tier (Gold - 10%, Silver - 5%, others - 2%). The result is stored in the DiscountedAmount column.
Control Statements in SQL: WHILE and LOOP
While both WHILE and LOOP are iterative control statements used to execute code repeatedly based on certain conditions, there are subtle differences between the two. Let’s delve into their practical applications through a few examples:
Example 1: Create a table with a WHILE loop:
DECLARE @Sales TABLE (Month INT, Amount DECIMAL(7,2));
DECLARE @MonthCounter INT = 1;
WHILE @MonthCounter <= 12
BEGIN
INSERT INTO @Sales (Month, Amount) VALUES (@MonthCounter, RAND() * 10000);
SET @MonthCounter = @MonthCounter + 1;
END
In this example, a temporary table named @Sales with columns for Months and Amounts is created. A WHILE loop populates the table with dummy sales data for each month using the RAND() function. This is achieved by incrementally increasing the value of @MonthCounter until it reaches 12.
Example 2: Process records with a LOOP using a cursor:
DECLARE @ProductID INT;
DECLARE product_cursor CURSOR FOR
SELECT ProductID
FROM Products
WHERE Discontinued = 0;
OPEN product_cursor;
FETCH NEXT FROM product_cursor INTO @ProductID;
WHILE @@FETCH_STATUS = 0
BEGIN
-- Perform operations on the @ProductID here (e.g., update, delete, etc.)
FETCH NEXT FROM product_cursor INTO @ProductID;
END
CLOSE product_cursor;
DEALLOCATE product_cursor;
In this example, a LOOP is created using a cursor named product_cursor to process all non-discontinued products in the Products table. The WHILE loop evaluates the @@FETCH_STATUS system variable, continuing to execute as long as there are more records to process. The loop terminates once all records have been processed.
By thoroughly understanding and applying these examples of control statements in SQL, you can improve your ability to manage and manipulate databases with precision and effectiveness. This newfound expertise will empower you to create sophisticated database solutions and streamline your data management processes.
Control Statements in SQL - Key takeaways
Control Statements in SQL Definition: Set of commands that control the flow of a program, enabling decisions and actions depending on different conditions.
Types of Control Statements in SQL: IF...THEN...ELSE, WHILE, FOR, CASE, and LOOP statements.
Control flow Statements in SQL: Essential for managing and working with data, such as conditional control statements (IF, CASE) and iterative control statements (WHILE, FOR).
Examples of SQL Control Statements: IF statements for condition-based execution, CASE statements for versatile conditions, WHILE and LOOP for iterative processes.
Control Statements in SQL with Example: IF...THEN...ELSE, simple and searched CASE expressions, WHILE loops, FOR loops with cursors.
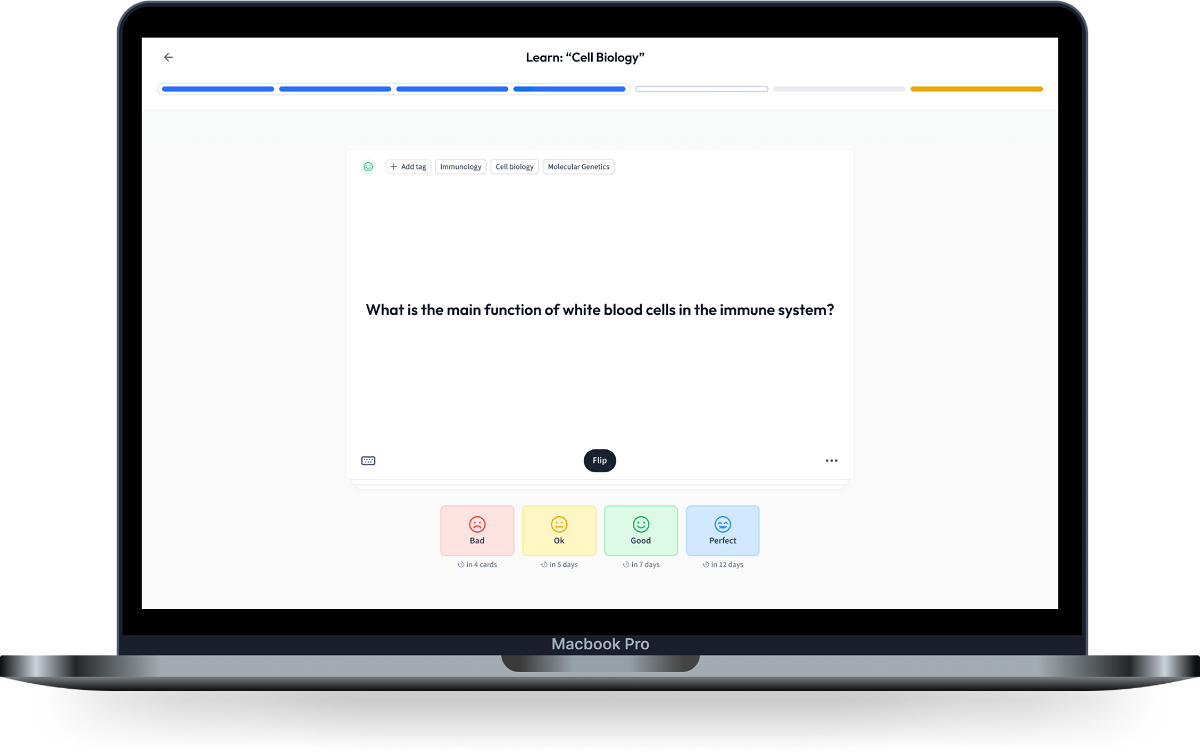
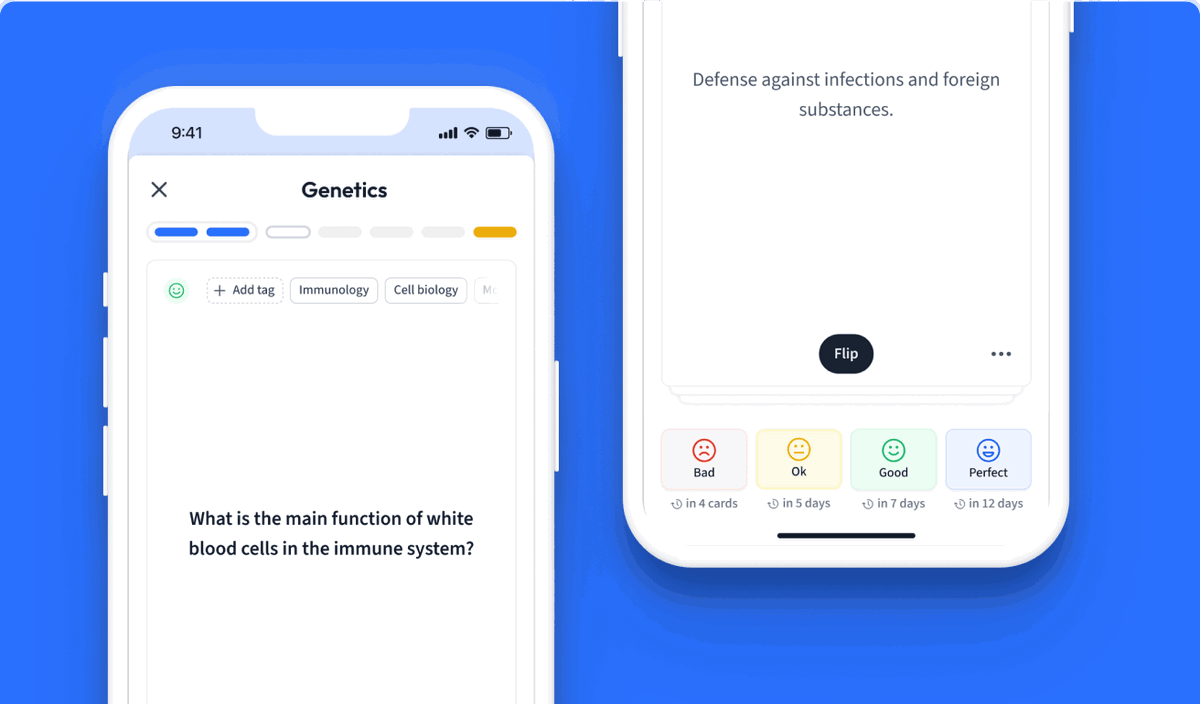
Learn with 59 Control Statements in SQL flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Control Statements in SQL
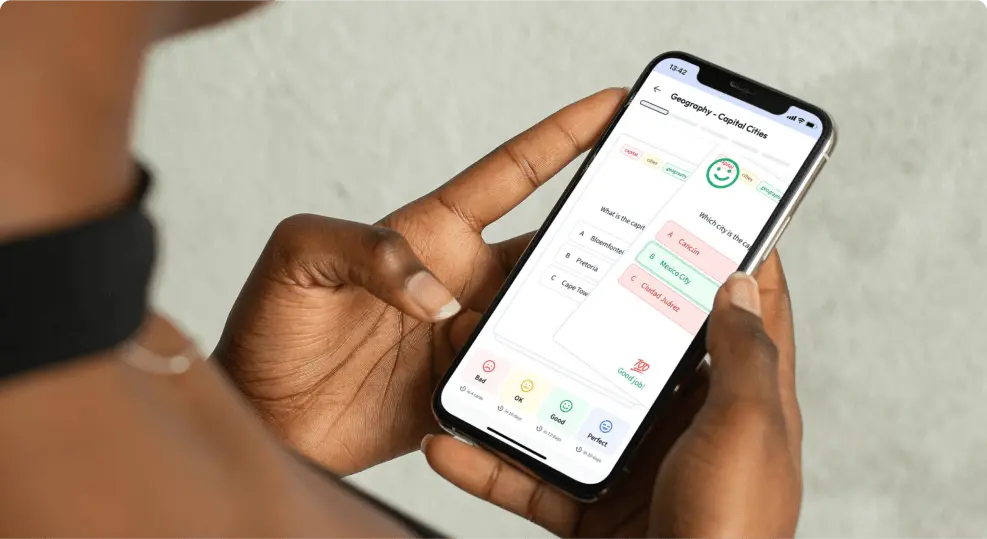
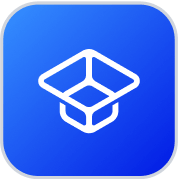
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more