Understanding SQL SELECT: SQL Select Explained
SQL (Structured Query Language) is a popular language used in managing databases and performing operations like adding, modifying and retrieving data. SQL SELECT is a fundamental component of SQL that allows you to query and retrieve data from a database table. It enables you to define the specific columns and rows you want to select and the conditions that must be met for the data to be returned.
Core Components of SQL SELECT Statements
An SQL SELECT statement consists of various parts, each serving a specific purpose in extracting data from the database. These parts are:
- SELECT clause: This is the main component that specifies the columns you want to retrieve from the table. You can use an asterisk (*) to select all columns, or list the individual column names separated by commas.
- FROM clause: The FROM clause indicates the table(s) from which the data should be retrieved. In case of multiple tables, SQL uses JOIN operations to combine them based on a specified relation.
- WHERE clause: WHERE is an optional component that filters the rows of data based on specified conditions - data must meet these conditions to be returned in the output.
- GROUP BY clause: This is another optional component that groups rows with similar values in specified columns. Often used with aggregate functions like COUNT, SUM or AVG to perform calculations on each group.
- ORDER BY clause: Allows you to sort the result set by one or more columns. You can also specify the sorting order - ASC (ascending) or DESC (descending).
- Limit clause: This optional part limits the number rows returned from the query, which can be useful to handle large datasets. OFFSET can also be used in combination to specify the starting point of the data retrieval.
Basic Syntax and Structure of SQL SELECT
The SQL SELECT statement follows a certain syntax and structure that dictates how the various components should be arranged. A typical SELECT statement can be broken down into its essential parts as shown below:
SELECT column1, column2, ... FROM table_name WHERE condition GROUP BY column(s) ORDER BY column(s) [ASC | DESC] LIMIT number OFFSET number;
The SELECT and FROM clauses are mandatory while others are optional, depending on your specific requirements. Use of brackets to highlight optional components as shown above in the syntax structure is not required but highlights the different components and their importance.
Here's a practical example of a simple SQL SELECT statement:
SELECT first_name, last_name, age FROM customers WHERE age > 18 ORDER BY last_name ASC;
In this example, we are selecting the first_name, last_name, and age columns from the customers table. We filter the data to only display customers with an age greater than 18 and sort the result set by the last_name column in ascending order.
Understanding SQL SELECT statements is crucial for any individual working with databases as it forms the foundation for database operations. By learning the core components, syntax and structure, you can start writing queries to access and manipulate data efficiently within a database.
SQL SELECT Statement Examples
Throughout this article, we will delve into several examples of SQL SELECT statements, focusing on different components and their usage. By understanding these components and their practical applications, you will be able to construct SQL SELECT statements based on your specific requirements.
Selecting All Columns and Rows
When you want to retrieve all columns and rows from a table, you can use the SQL SELECT statement with an asterisk (*) symbol – which represents 'all columns'. Let's take a look at an example:
SELECT * FROM employees;
In this query, we select all columns and rows from the employees table. The result set will display every row with all the columns available in the table. While this can be useful in certain situations, selecting all columns and rows may not always be the most efficient approach, especially when dealing with large databases. To avoid potential performance issues, try to select only the columns and rows you need.
Selecting Specific Columns
In most cases, you only need to retrieve specific columns from a table. You can achieve this by listing the required column names in the SQL SELECT statement, separated by commas. This allows more control over the data you want to retrieve and can lead to improved performance and readability. Let's examine an example:
SELECT first_name, last_name, job_title FROM employees;
In this query, we select the first_name, last_name, and job_title columns from the employees table. The result set will display only the required columns and all rows in the table.
Selecting specific columns is particularly important when you want to:
- Improve query performance by reducing the amount of data to be processed
- Enhance readability by focusing on relevant columns
- Limit the disclosure of sensitive information by selecting only public or non-sensitive columns
Using WHERE Clause in SQL SELECT
The WHERE clause is a powerful tool that allows you to filter rows in your result set based on one or more conditions. By using the WHERE clause, you can select rows that meet specific criteria, making your queries more precise and efficient. Let's take a look at a basic example:
SELECT first_name, last_name, salary FROM employees WHERE salary >= 50000;
In this query, we are selecting the first_name, last_name, and salary columns from the employees table, but we only want to display rows where the salary is greater than or equal to 50,000. The result set will only include rows that meet the specified condition.
The WHERE clause supports various types of conditions, including:
- Comparison operators: =, <>, !=, , <=, and >=
- Logical operators: AND, OR, and NOT
- IN, BETWEEN, and LIKE operators: for working with lists, ranges, and patterns respectively
Multiple conditions can be combined using AND, OR, and NOT operators to further refine your query. Consider the following example:
SELECT first_name, last_name, salary, job_title FROM employees WHERE salary >= 50000 AND job_title NOT LIKE '%Manager%';
In this query, we are selecting the first_name, last_name, salary, and job_title columns from the employees table with two conditions: the salary must be greater than or equal to 50,000, and the job_title must not contain the word 'Manager'. The result set will only include rows that meet both conditions.
Mastering the use of WHERE clause can significantly enhance the power and flexibility of your SQL SELECT statements, allowing you to perform complex data filtering and manipulation tasks with ease.
Advanced SQL SELECT Techniques
Beyond the basic SQL SELECT operations, there are a number of advanced techniques that provide even greater flexibility when querying and manipulating data in a database. These advanced SQL SELECT techniques include querying data from multiple tables, selecting distinct values, and using INSERT SELECT for combining data. Mastering these advanced techniques will significantly enhance your ability to retrieve and manipulate complex datasets within a database.
SQL SELECT From Multiple Tables
Quite often, the required data resides in multiple tables, and there is a need to combine or relate these tables in a specific way to retrieve the desired information. Using SQL SELECT from multiple tables is one such technique that enables you to retrieve data from more than one table in a single query. There are several types of table relationships that can be used:
- INNER JOIN
- LEFT JOIN (or LEFT OUTER JOIN)
- RIGHT JOIN (or RIGHT OUTER JOIN)
- FULL JOIN (or FULL OUTER JOIN)
- CROSS JOIN
INNER JOIN is the most commonly used table relationship, as it returns only matching rows from both tables. For instance, consider two tables: employees and departments. If we want to retrieve the employee's first name, last name and their department name, we can use the following INNER JOIN query:
SELECT employees.first_name, employees.last_name, departments.department_name FROM employees INNER JOIN departments ON employees.department_id = departments.department_id;
In this example, we specifying a common column (department_id) shared between the two tables as the joining condition. The result set will display only those rows where there is a match in both departments and employees tables based on the specified condition.
LEFT JOIN, RIGHT JOIN, and FULL JOIN are the types of OUTER JOINs, which allow you to retrieve data even if there is no match in one or both tables. LEFT JOIN returns all rows from the left table and matching rows from the right table. If no match is found, NULL values are returned for columns from the right table. RIGHT JOIN is similar, but it returns all rows from the right table and matching rows or NULLs from the left table. FULL JOIN combines both - it returns all rows from both tables, displaying NULLs when no match is found for either table.
CROSS JOIN, while less frequently used, can be useful in certain scenarios. It returns a Cartesian product between two tables, meaning that it combines each row from the first table with every row from the second table. This type of join can result in a large number of rows and should be used with caution, especially when dealing with large datasets.
SQL SELECT DISTINCT for Unique Values
When working with datasets containing duplicate rows, you may want to retrieve only distinct (unique) values for a particular column. The SQL SELECT DISTINCT keyword allows you to achieve this by eliminating duplicates and returning only unique rows for the specified column(s). The basic syntax for SELECT DISTINCT is:
SELECT DISTINCT column1, column2, ... FROM table_name;
For example, if we want to retrieve the unique job titles from the employees table, we can use the following SELECT DISTINCT query:
SELECT DISTINCT job_title FROM employees;
The result set will display all the distinct job titles found in the table, without presenting any duplicate values.
It is essential to note that SELECT DISTINCT works by comparing the specified columns' values and removing duplicates within those columns. If you want to retrieve unique rows based on multiple columns, you must list all the relevant columns within the SELECT DISTINCT statement. Keep in mind that the more columns you include, the more unique combinations become possible, which might affect the result set's size.
INSERT SELECT in SQL for Combining Data
There are times when you may want to insert data from one table into another, either to create a new table or to update an existing one. The INSERT SELECT statement in SQL allows you to achieve this by retrieving data using a SELECT query and then inserting the result set into another table. There are two main use cases for using INSERT SELECT:
- Inserting data into a new table
- Inserting data into an existing table
To insert data into a new table, you can use the following INSERT SELECT syntax:
CREATE TABLE target_table AS SELECT column1, column2, ... FROM source_table WHERE condition;
For instance, we can create a new table called 'manager_employees' containing the first_name, last_name, and job_title columns from the employees table where the job_title contains the word 'Manager':
CREATE TABLE manager_employees AS SELECT first_name, last_name, job_title FROM employees WHERE job_title LIKE '%Manager%';
If you want to insert data into an existing table, you should use the following syntax:
INSERT INTO target_table (column1, column2, ...) SELECT column1, column2, ... FROM source_table WHERE condition;
For example, let's say we want to copy the names of employees with a salary greater than or equal to 50,000 from the employees table into an existing table called 'high_salary_employees':
INSERT INTO high_salary_employees (first_name, last_name) SELECT first_name, last_name FROM employees WHERE salary >= 50000;
The result is that all the desired data from the employees table will be inserted into the 'high_salary_employees' table, meeting the specified condition.
Understanding and mastering advanced SQL SELECT techniques will significantly increase your ability to manipulate, retrieve and combine datasets within a database effortlessly. By learning and applying these advanced techniques, you can take your SQL skills to the next level and become more efficient at handling complex data retrieval tasks.
SQL SELECT - Key takeaways
SQL SELECT statements are used to query and retrieve data from database tables.
Core components of SQL SELECT statements include SELECT, FROM, WHERE, GROUP BY, ORDER BY, and LIMIT clauses.
SQL SELECT statement examples include selecting specific columns, using WHERE clause, and querying data from multiple tables.
Advanced techniques include SQL SELECT DISTINCT for unique values and INSERT SELECT in SQL for combining data.
Mastering SQL SELECT is vital for effectively managing, retrieving, and presenting information stored in relational databases.
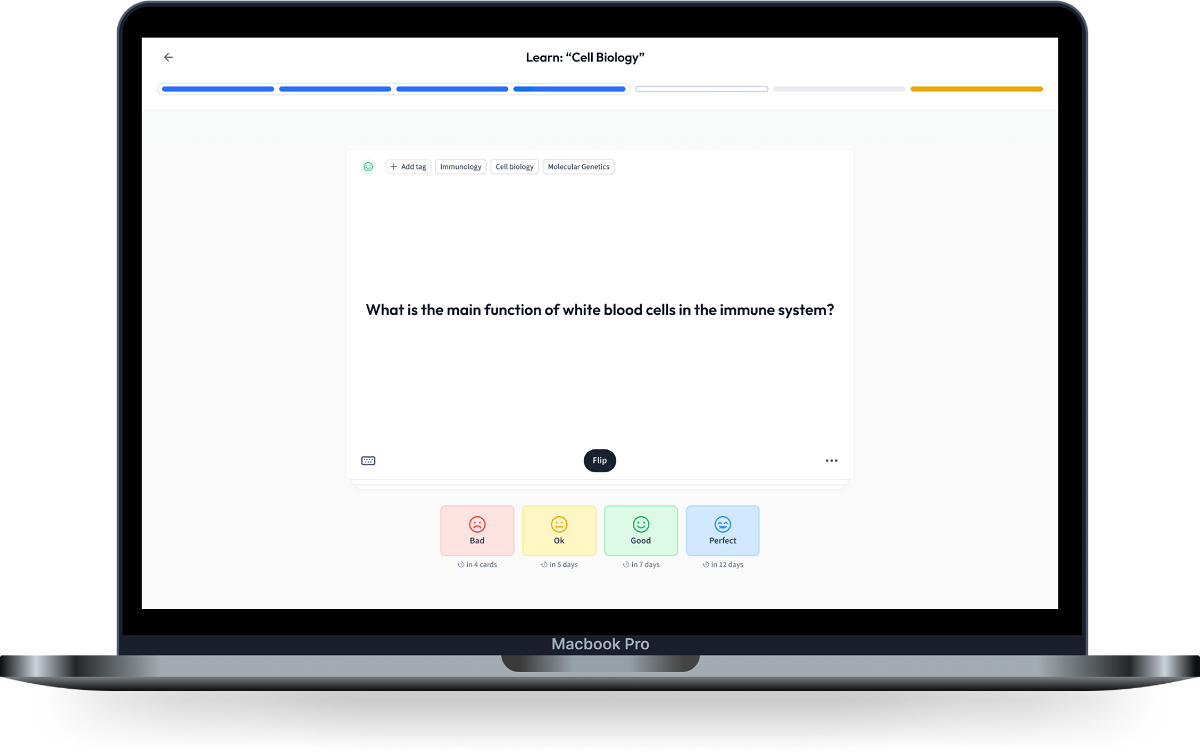
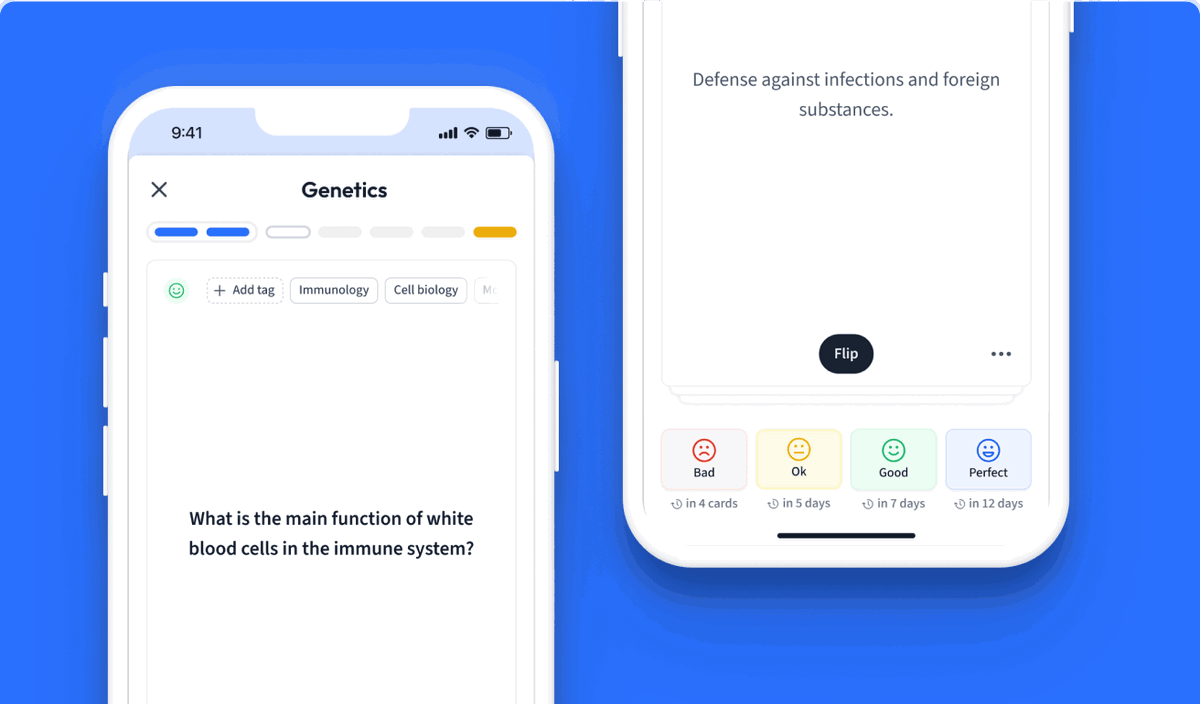
Learn with 15 SQL SELECT flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about SQL SELECT
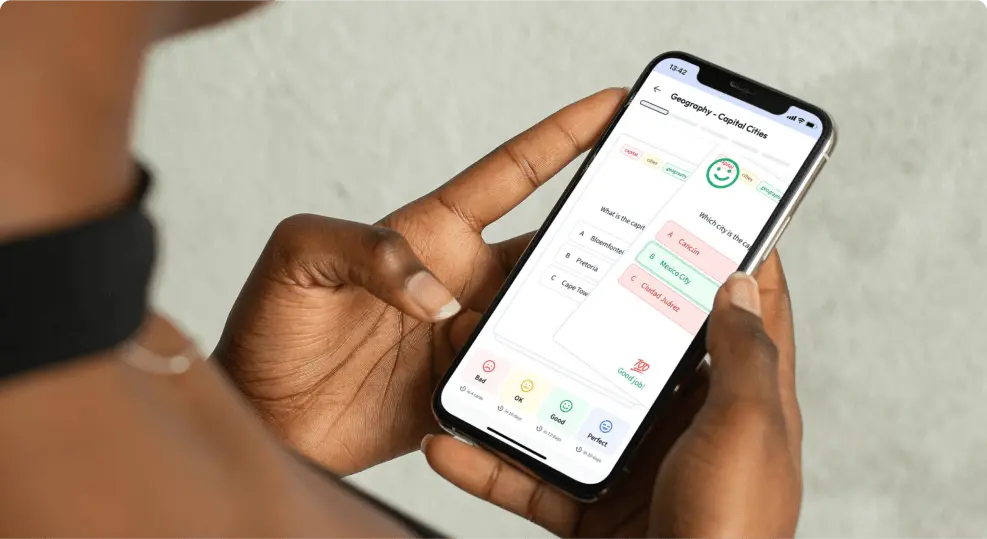
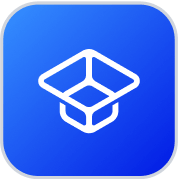
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more