SQL Expressions Basics
SQL expressions are used to combine, manipulate, and compare values in SQL statements to produce a meaningful result. An SQL expression is a combination of one or more values, operators, and SQL functions that result in a single value. They play a crucial role in crafting complex SQL queries and designing database structures.
Definition and Purpose of SQL Expressions
An SQL Expression is a formula that involves various elements, including field names, values, and operators, which results in a single value. They can manipulate data, perform calculations, compare values, and even concatenate data from multiple sources. The primary purpose of SQL expressions is to filter, sort, and combine data so that you can retrieve specific information from a database.
In other words, SQL expressions are the fundamental building blocks that help you create and perform various operations on your datasets in a convenient and efficient manner. They enable you to extract, manipulate, and evaluate data across different tables or columns in a database.
Building Blocks of SQL Expressions
SQL expressions are formed using these key building blocks:
1. Values: These can be constants (like a specific number or string), variables, or column references. You can use these values in your SQL expression to perform operations or make comparisons.
2. Operators: Operators are used in conjunction with values to perform the desired operations, such as arithmetic, comparison, or logical operations. Examples of operators include '+', '-', '*', '/', 'AND', 'OR', and '='.
SQL Expressions Explained with Examples
Arithmetic SQL expressions allow you to perform basic mathematical operations such as addition, subtraction, multiplication, and division on numeric values and columns within an SQL query. This can be helpful in calculating derived values from the data stored in the database, such as the total cost of items or the average salary of employees.
Addition and Subtraction in SQL Expressions
Addition and subtraction operations in SQL expressions are performed using the '+' and '-' symbols, respectively. Here's an example of using addition and subtraction operators in an SQL expression: Consider the following table 'orders':
| order_id | item_price | shipping_cost | |----------|------------|---------------| | 1 | 35 | 5 | | 2 | 25 | 8 | | 3 | 45 | 6 |
To calculate the total cost, including the item price and shipping cost, you could use the addition operator in a SELECT statement:
SELECT order_id, item_price + shipping_cost
AS total_cost
FROM orders;
The result of the addition will be shown in a new column 'total_cost':
| order_id | total_cost | |----------|------------| | 1 | 40 | | 2 | 33 | | 3 | 51 |
Similarly, if you want to calculate the difference between the item price and a discount value, you could use the subtraction operator. For example, if the discount for each order is £10:
SELECT order_id, item_price - 10 AS discounted_price FROM orders;The 'discounted_price' column will show the result of the subtraction:
|order_id | discounted_price | |----------|------------------| | 1 | 25 | | 2 | 15 | | 3 | 35 |Multiplication and Division in SQL Expressions
Multiplication and division operations in SQL expressions are performed using the '*' and '/' symbols, respectively. You can use these operators to perform calculations that involve multiplying or dividing numeric values or columns. Here's an example of using the multiplication and division operators within an SQL expression:
Consider the following table 'sales':
| sale_id | sale_price | sale_quantity | |---------|------------|---------------| | 1 | 10 | 2 | | 2 | 20 | 5 | | 3 | 15 | 3 |
To calculate the total value of each sale, you can multiply 'sale_price' by 'sale_quantity':
SELECT sale_id, sale_price * sale_quantity AS total_value FROM sales;
The result will be displayed in the 'total_value' column:
| sale_id | total_value | |---------|-------------| | 1 | 20 | | 2 | 100 | | 3 | 45 |
If you want to calculate the price per unit for each sale, you can divide 'total_value' by 'sale_quantity':
SELECT sale_id, total_value / sale_quantity AS price_per_unit FROM sales;
The 'price_per_unit' column shows the result of the division:
| sale_id | price_per_unit | |---------|----------------| | 1 | 10 | | 2 | 20 | | 3 | 15 |
Implementing Comparison SQL Expressions
Comparison SQL expressions are used to compare the values of columns or constants and return a Boolean value (TRUE or FALSE) or UNKNOWN, depending on whether the comparison is satisfied or not. This is particularly useful for filtering data based on specific conditions. Common comparison operators include '=', '>', '=', and '<>'.
Equivalence and Inequality SQL Expressions Example
To demonstrate equivalence and inequality in SQL expressions, consider the following table 'employees':
| employee_id | department | salary | |-------------|------------|--------| | 1 | HR | 35000 | | 2 | IT | 45000 | | 3 | IT | 38000 | |x
Advanced SQL Expressions Techniques
SQL expressions can be significantly enhanced by incorporating various built-in functions available in SQL. These functions help perform complex calculations, aggregate data, and manipulate date and time-related components within SQL queries.
Aggregate Functions in SQL Expressions
Aggregate functions let you perform calculations on a group of values, such as the sum, average, minimum, maximum, or count of a particular column. These functions prove extremely useful in generating summary statistics, assessing trends, and analysing aggregated data. Some common aggregate functions include:
- SUM(column_name): Calculates the sum of all values in the specified column.
- AVG(column_name): Calculates the average of all values in the specified column.
- MIN(column_name): Returns the minimum value found in the specified column.
- MAX(column_name): Returns the maximum value found in the specified column.
- COUNT(column_name): Returns the total number of rows in the specified column.
- To demonstrate the use of aggregate functions with SQL expressions, consider the following table 'sales_data':
| sale_id | product_id | product_price | quantity | |---------|------------|---------------|----------| | 1 | 1001 | 20 | 5 | | 2 | 1002 | 30 | 10 | | 3 | 1003 | 25 | 8 | | 4 | 1001 | 20 | 15 |
To find the total revenue, you can use the SUM function in conjunction with the multiplication operator on 'product_price' and 'quantity':
SELECT SUM(product_price * quantity) as total_revenue FROM sales_data;
The result will display the total revenue:
| total_revenue | |---------------| | 825 |
Built-in SQL Functions with Expressions
SQL has several built-in functions that can be used to perform complex calculations and manipulate data within SQL expressions. These functions can be particularly useful when dealing with numerical, string, or datetime data types or for programming conditional logic-based tasks. Some common built-in functions include:
- ABS: Returns the absolute value of a number.
- LENGTH: Returns the character length of a string.
- UPPER: Converts all characters in a string to uppercase.
- LOWER: Converts all characters in a string to lowercase.
- SUBSTRING: Extracts a specific portion of a string based on the start and length parameters.
- SQRT: Computes the square root of a given number.
- ROUND: Rounds a numeric value to the specified decimal places.
- IFNULL: Returns the first non-null value from the provided argument list.
- For example, the following query can be used to calculate the square root of the 'total_revenue' computed earlier:
SELECT SQRT(SUM(product_price * quantity)) as revenue_sqrt FROM sales_data;
The result will display the square root of the total revenue: | revenue_sqrt | |-----------------| | 28.722813232686 |
Managing Date and Time with SQL Expressions
Date and time management is an essential aspect of SQL and involves a wide range of functions to manipulate, format, and perform calculations on date and time data types. These functions can be used in SQL expressions to filter and sort data based on specific date and time conditions, as well as to calculate time intervals and perform various datetime-related operations.
Current Date and Time Functions in Expressions
To work with the current date and time in SQL expressions, various database systems offer dedicated functions such as:
- GETDATE(): Returns the current date and time in SQL Server.
- NOW(): Returns the current date and time in MySQL and PostgreSQL.
- SYSDATE: Returns the current date and time in Oracle DB.
- CURRENT_DATE: Returns the current date in databases like PostgreSQL and MySQL.
- CURRENT_TIME: Returns the current time in databases like PostgreSQL and MySQL.
- For example, you can use the NOW() function in MySQL to display the current date and time:
SELECT NOW() as current_datetime;
The result will display the current date and time: | current_datetime | |------------------------| | 2022-12-10 12:34:56 |
Adding and Subtracting Time Values in SQL Expressions
SQL expressions can be used to add or subtract time intervals from specific date and time values. Several databases offer functions, such as DATE_ADD, DATE_SUB, or INTERVAL, to perform calculations on date and time data types. These functions enable you to compute the difference between two time values or add a specific time interval to a given date.
For instance, consider the following table in MySQL 'events':
| event_id | event_name | event_date | |----------|------------|------------| | 1 | Party | 2022-12-20 | | 2 | Meeting | 2022-12-25 | | 3 | Conference | 2022-12-30 |
To find out the date after adding five days to each event date, you could use the DATE_ADD and INTERVAL functions in MySQL:
SELECT event_name, DATE_ADD(event_date, INTERVAL 5 DAY) as new_date FROM events;
The result will display the new dates for each event: | event_name | new_date | |------------|------------| | Party | 2022-12-25 | | Meeting | 2022-12-30 | | Conference | 2023-01-04 |
In a similar manner, you can subtract time intervals from date values using the DATE_SUB function.
SQL Expressions - Key takeaways
SQL Expressions are formulas consisting of values, operators, and functions that produce a single value, used for database querying and data manipulation.
Types of SQL Expressions include arithmetic, comparison, logical, and concatenation expressions.
Implementing SQL Expressions involves using various operators, such as '+', '-', '*', and '/', for arithmetic operations or '=' and '<>' for comparisons.
Advanced SQL Expressions techniques include using built-in functions to perform calculations, aggregate data, and manipulate date and time components.
SQL Expressions can be further enhanced with functions like SUM, AVG, MIN, MAX, COUNT, and various date and time functions to address complex querying needs.
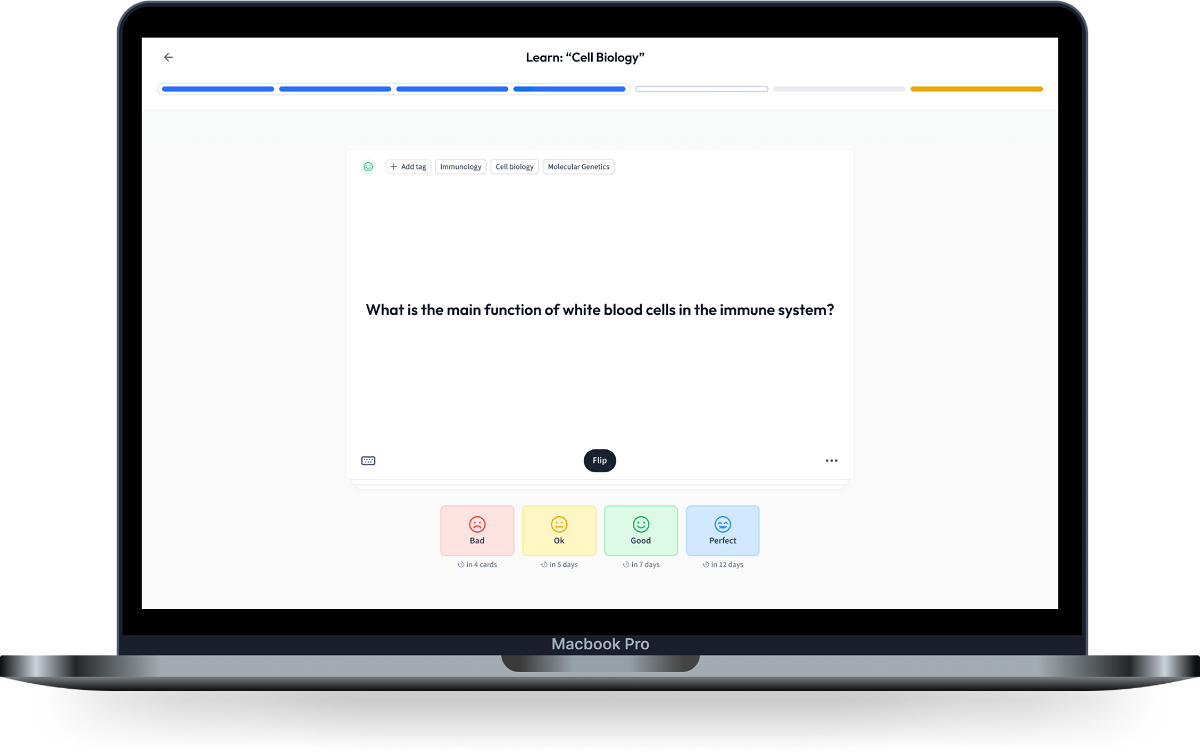
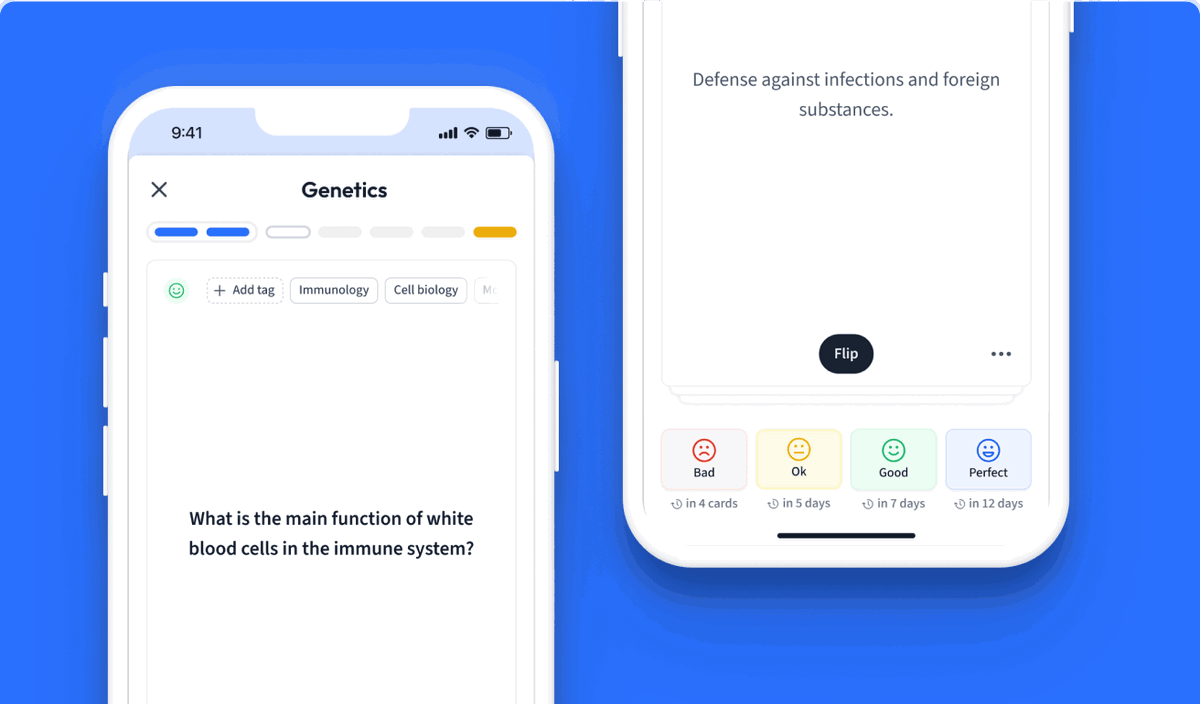
Learn with 11 SQL Expressions flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about SQL Expressions
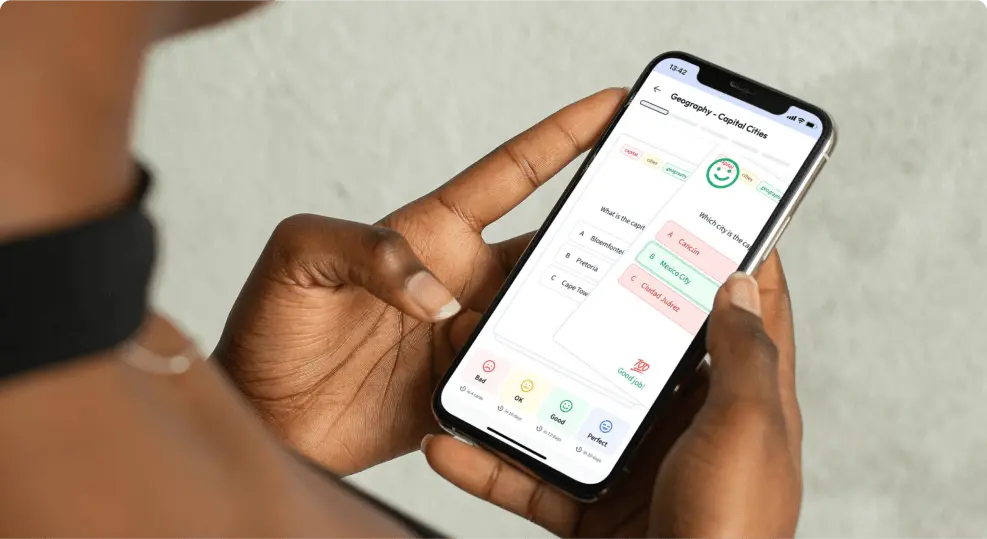
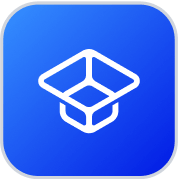
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more