Modifying Data in SQL: An Overview
When working with databases, you often need to add, update or remove data. In SQL (Structured Query Language), modifying data is a common and essential task, ensuring that the information remains accurate and up-to-date. In this article, you will learn about the fundamental concepts for modifying data in SQL, including data types, operations, and the most used SQL statements for data manipulation.
Fundamental Concepts for Modifying Data in SQL
To effectively modify data in SQL, you need to understand some basic concepts such as tables, columns, and rows. A database table consists of columns, which represent attributes, and rows, representing individual data records. Here, we'll discuss the core SQL statements used for modifying data and offer guidance on their applications.
SQL statements used for data modification are collectively known as Data Manipulation Language (DML). They include INSERT, UPDATE, DELETE and SELECT statements, allowing you to insert new rows, modify existing rows, or remove rows from a table, as well as retrieving data based on certain criteria.
Understanding SQL Data Types and Operations
Different data types and operations are available in SQL that affect how you work with and modify data. Recognising these data types and associated operations is crucial to efficient data manipulation.
Let's examine the most common SQL data types:
- CHAR(n): A fixed-length character string with a maximum length of n characters
- VARCHAR(n): A variable-length character string with a maximum length of n characters
- INT: An integer (whole number) value
- DECIMAL(p, s): A signed decimal number with a total of p digits and s digits after the decimal point
- DATE: A date value represented in the format 'YYYY-MM-DD'
- TIMESTAMP: A combination of date and time values
Understanding how operations work with these data types is essential for modifying data in SQL:
Arithmetic operations, such as addition (+), subtraction (-), multiplication (*) and division (/), can be applied to numeric data types like INT and DECIMAL. Similarly, string operations, such as concatenation (joining two strings together) or substring extraction (retrieving a portion of a string), work with character data types like CHAR and VARCHAR. Meanwhile, date and time functions can be used to manipulate DATE and TIMESTAMP data types, offering greater versatility in how you work with and modify data.
Now let's discuss an example of modifying data by changing the price of a product in a table named 'Products', which has three columns: 'ID', 'ProductName' and 'Price'. First, you'll need to understand the data type of the 'Price' column. To modify the data, you can use an UPDATE statement and the appropriate arithmetic operation, for instance, increasing the product's price by 10%:
UPDATE Products
SET Price = Price * 1.10
WHERE ID = 1;
Learning how SQL data types and operations work together allows you to efficiently modify data and ensures you make the most of your database activities.
How to Modify Data in SQL: Step-by-Step Guide
Modifying data in SQL involves using specific SQL commands to insert, update, and delete data from tables. This step-by-step guide covers the essentials of modifying data in SQL, ensuring you can effectively manage your database records.
SQL Command to Modify Data in a Table
There are three primary SQL commands to modify data in a table - INSERT, UPDATE, and DELETE. Each command has its specific syntax and usage, allowing you to insert new rows, modify existing rows, and remove rows from the table. These commands belong to the Data Manipulation Language (DML) subset of SQL, enabling the alteration of data within database tables.
INSERT: The INSERT command adds new rows of data to a table.
UPDATE: The UPDATE command modifies existing rows in a table based on specified conditions.
DELETE: The DELETE command removes rows from a table, depending on the given criteria.
Inserting Data into a Table
The INSERT command allows you to add new rows of data to a table. There are two primary syntaxes for the INSERT command: INSERT INTO with column names and values, and INSERT INTO with SELECT.
The first method specifies the column names and corresponding values within the INSERT INTO statement. Its general form is as follows:
INSERT INTO table_name (column1, column2, column3, ... )
VALUES (value1, value2, value3, ... );
For example, to insert a new product into the 'Products' table with columns 'ID', 'ProductName', and 'Price', you can use the following statement:
INSERT INTO Products (ID, ProductName, Price)
VALUES (1, 'Example Product', 19.99);
The second method, INSERT INTO with SELECT, inserts data from another table or query result into the target table. This method is useful for copying data between tables or inserting data based on specific criteria. Its general form is:
INSERT INTO target_table (column1, column2, column3, ... )
SELECT column1, column2, column3, ...
FROM source_table
WHERE condition;
For example, to insert a list of products with a price lower than £10 from a 'SaleProducts' table, use the following statement:
INSERT INTO Products (ID, ProductName, Price)
SELECT ID, ProductName, Price
FROM SaleProducts
WHERE Price < 10;
Updating Data in a Table
The UPDATE command allows you to modify existing rows in a table based on specified conditions. Its usage consists of specifying the columns to be updated, the new values for each column, and the conditions the record must meet to be updated.
The general syntax for the UPDATE command is:
UPDATE table_name
SET column1 = new_value1, column2 = new_value2, ...
WHERE condition;
To update the price of a product in the 'Products' table, you would use the following statement:
UPDATE Products
SET Price = 24.99
WHERE ProductName = 'Example Product';
Deleting Data from a Table
The DELETE command allows you to remove rows from a table based on specified conditions. The syntax is relatively straightforward, requiring only a reference to the table and the conditions for deletion.
The general syntax of the DELETE command is:
DELETE FROM table_name
WHERE condition;
For instance, if you need to delete a product from the table 'Products', you can use the following statement:
DELETE FROM Products
WHERE ProductName = 'Example Product';
By understanding and applying these SQL commands, you can efficiently manipulate data in your database tables, ensuring records stay accurate and up-to-date.
Modifying Data Types in SQL: Techniques and Best Practices
Altering data types in SQL tables is sometimes necessary as your database evolves to meet changing requirements. A number of techniques and best practices exist to ensure that you modify data types safely and efficiently, while maintaining data integrity and database performance.
Altering Data Types in SQL Tables
Modifying data types mainly involves changing the structure of a table without losing its existing data. The SQL command used for this purpose is the ALTER TABLE statement. It allows you to change the data type of a column, add or drop columns, and rename columns, among other operations.
ALTER TABLE: The ALTER TABLE command is used to modify a table's structure, such as adding or dropping columns, changing data types, and setting default values for columns.
The general syntax for modifying data types is as follows:
ALTER TABLE table_name
ALTER COLUMN column_name
[new_data_type] [constraints];
There are a few important considerations when altering data types in SQL tables:
- Ensure compatibility between the old and new data types to prevent data loss.
- Test your changes in a development or staging environment before applying them to the production database.
- Perform a backup of your database before making significant changes, so you can easily revert if needed.
- Consider any potential performance implications of altering data types, particularly for large tables.
Changing Data Types Safely and Efficiently
To change data types without losing data and with minimal impact on performance, follow these recommendations:
- Perform a compatibility check between the existing and new data types. If the conversion is not straightforward, you may need to create a temporary column with the desired data type. Once the data is successfully converted, you can drop the original column and rename the new column to the original name.
- Create a copy of the table with the new column data types and constraints. Then, use the INSERT INTO SELECT statement to copy data from the original table to the new one. Finally, rename or replace the old table with the new one. This method can be more efficient for large tables, as the table can be accessed while data is being copied.
- Consider database performance and indexing strategies when altering data types, as the change could affect query performance and storage requirements. Modify your indexes and query optimization techniques as needed to maintain optimal performance.
- Perform proper testing and review before applying changes to the production database. Always create backups and test your modifications in a development or staging environment before applying them in production.
For example, to change the data type of the 'Price' column in the 'Products' table from 'INT' to 'DECIMAL(10, 2)', you can use the following SQL statement:
ALTER TABLE Products
ALTER COLUMN Price DECIMAL(10, 2);
Following these best practices will ensure smooth transitions when modifying data types, allowing your database to adapt to evolving business needs while maintaining performance and integrity.
Modifying Data in SQL Explained: Common Scenarios and Solutions
As you work with databases, you'll come across various scenarios requiring data modification. In these situations, understanding best practices, solutions, and potential challenges can help you manage your SQL databases effectively and efficiently.
Dealing with Data Modification Challenges
There are numerous challenges that can arise when modifying data in SQL, such as dealing with locked records, managing data integrity, and safely performing modifications on related tables. Addressing these challenges requires understanding the underlying principles, techniques, and possible solutions.
Joining Tables for Data Modification
When working with related tables, you often need to associate information from multiple tables to update records or complete an action. To do this, you must join tables using specific techniques. Joining tables can be performed using various methods, such as INNER JOIN, OUTER JOIN, and CROSS JOIN. Choosing the appropriate method depends on the specific requirements of your operation.
Consider the following tips when joining tables for data modification:
- Select the appropriate join type based on the desired result and relationship between the tables. For example, use INNER JOIN when updating records that have matching entries in both tables, or LEFT JOIN when updating records in one table even if there are no matching entries in the other table.
- Use aliases when working with multiple tables with similar column names to improve readability.
- Ensure proper indexing on join conditions to improve query performance.
- Whenever possible, use subqueries and CTE (Common Table Expressions) to break complex queries into smaller, more manageable parts.
For instance, suppose you want to update the price of all 'Products' based on a specific discount applied in a 'Discounts' table. The following query demonstrates an INNER JOIN for this purpose:
UPDATE Products
SET Products.Price = Products.Price * (1 - Discounts.DiscountPercentage / 100)
FROM Products
INNER JOIN Discounts ON Products.DiscountID = Discounts.DiscountID;
Handling Locked Records during Data Modification
Locked records occur when two or more concurrent transactions try to modify the same data. These locks are designed to maintain data consistency and integrity. However, they can occasionally lead to challenges when performing updates or deletions during data modification. To handle locked records effectively, it is essential to understand the following concepts:
- Transaction Isolation Levels: Determine the level of concurrency control applied during transactions. Higher isolation levels provide more consistency but can result in increased locking, whereas lower levels allow for better concurrency at the cost of increased risk of data inconsistency.
- Deadlocks: Situations where two or more transactions are waiting for each other to complete, causing both to be blocked indefinitely. SQL Server automatically detects and resolves deadlocks by choosing one transaction as the victim and aborting it, allowing the other transaction(s) to proceed.
- Locking Hints: Provide explicit control over locking behaviour for a given operation. You can specify hints such as ROWLOCK, PAGELOCK, TABLOCK, and NOWAIT to manipulate locks at the row, page, or table level, or to force the operation to fail immediately if a lock cannot be acquired.
Here are some techniques for handling locked records during data modification:
- Avoid long-running transactions, as they can increase the likelihood of record locking. Try to modify data as quickly as possible and commit the transaction.
- Break large modification operations into smaller, manageable chunks. This technique reduces the likelihood of locked records and eases database contention.
- Use lock timeouts or apply locking hints to limit the impact of record locking, reducing the chances of blocking other transactions.
- Optimise your queries and indexes to minimise the time that records remain locked and prevent deadlocks.
Implementing these techniques can help you prevent and handle locked records during data modification, ensuring smooth, efficient performance in your SQL databases.
Modifying Data in SQL Example: Real-World Applications
Modifying data in SQL is widely used across various industries, from e-commerce and finance to healthcare and logistics. Knowledge of data manipulation techniques is vital for maintaining, updating, and analysing information in your SQL databases. In this section, we will discuss practical uses of SQL data modification and delve into case studies that demonstrate efficient data modification in real-world applications.
Practical Uses of SQL Data Modification
SQL data modification plays a crucial role in numerous sectors, ensuring that information stored in databases is accurate, up-to-date, and reliable. Some practical applications of SQL data modification include:
- Inventory management: Updating stock levels, adding new products, and removing discontinued items in e-commerce and retail databases
- Financial transactions: Recording, updating, and deleting transactions in banking and finance systems, such as deposits, withdrawals, and transfers
- Customer relationship management (CRM): Adding, updating, and deleting contact information, preferences, and history for customer records
- Logistics: Tracking and updating shipments, deliveries, and routing information in transportation and logistics databases
- Healthcare: Managing patient records, including personal information, medical history, prescriptions, and appointments
Understanding how to modify data effectively in SQL is essential for addressing these diverse industry requirements and maintaining a reliable, efficient data management system.
Case Studies on Efficiently Modifying Data in SQL
In this section, we examine case studies that highlight the importance of efficiently modifying data in SQL and discuss the techniques and best practices used in each scenario.
Case Study 1: An e-commerce platform wants to apply a time-limited promotion across all products in their database:
- Create a new table 'Promotions' to store information such as promotion name, start date, end date, and discount percentage.
- Use the INSERT statement to add new promotions to the 'Promotions' table.
- Implement an UPDATE statement with an INNER JOIN, linking the 'Products' and 'Promotions' tables, to apply the promotion pricing to the relevant products within the valid date range.
- After the promotion ends, use another UPDATE statement with an INNER JOIN to revert product prices back to their original values.
Case Study 2: A healthcare provider needs to update patient records after a series of laboratory tests:
- Create a new table 'LabResults' with columns for patient ID, test date, test type, and result.
- Perform an INSERT INTO statement to incorporate the new laboratory test results into the 'LabResults' table.
- Use an UPDATE statement with an INNER JOIN between the 'Patients' and 'LabResults' tables to update patient records with the latest laboratory test results.
- Employ appropriate data validation and integrity checks to ensure accuracy when modifying patient records.
Case Study 3: A logistics company needs to update shipment statuses and reroute deliveries affected by unexpected weather-related disruptions:
- Use a SELECT statement with a WHERE clause to identify and retrieve shipments affected by the weather disruptions.
- Update the shipment status for the affected records usingthe UPDATE command.
- Prepare an alternative routing plan for the disrupted shipments.
- Apply the new routing plan to the 'Shipments' table with an UPDATE statement, ensuring changes comply with all required business rules and constraints.
These case studies emphasize the significance of effective data modification in SQL, as well as the importance of understanding and implementing best practices for complex real-world applications.
Modifying Data in SQL - Key takeaways
Modifying Data in SQL: Essential skill for managing databases, including inserting, updating, and deleting data from tables.
SQL Data Types and Operations: Crucial to efficient data manipulation, such as CHAR, VARCHAR, INT, DECIMAL, DATE, and TIMESTAMP.
SQL Commands for Data Modification: Using INSERT, UPDATE, and DELETE to efficiently manage data in database tables.
Modifying Data Types in SQL: Techniques and practices for altering data types, ensuring data integrity and database performance.
Practical Uses and Case Studies: Real-world applications showcasing efficient data modification in sectors like e-commerce, finance, healthcare, and logistics.
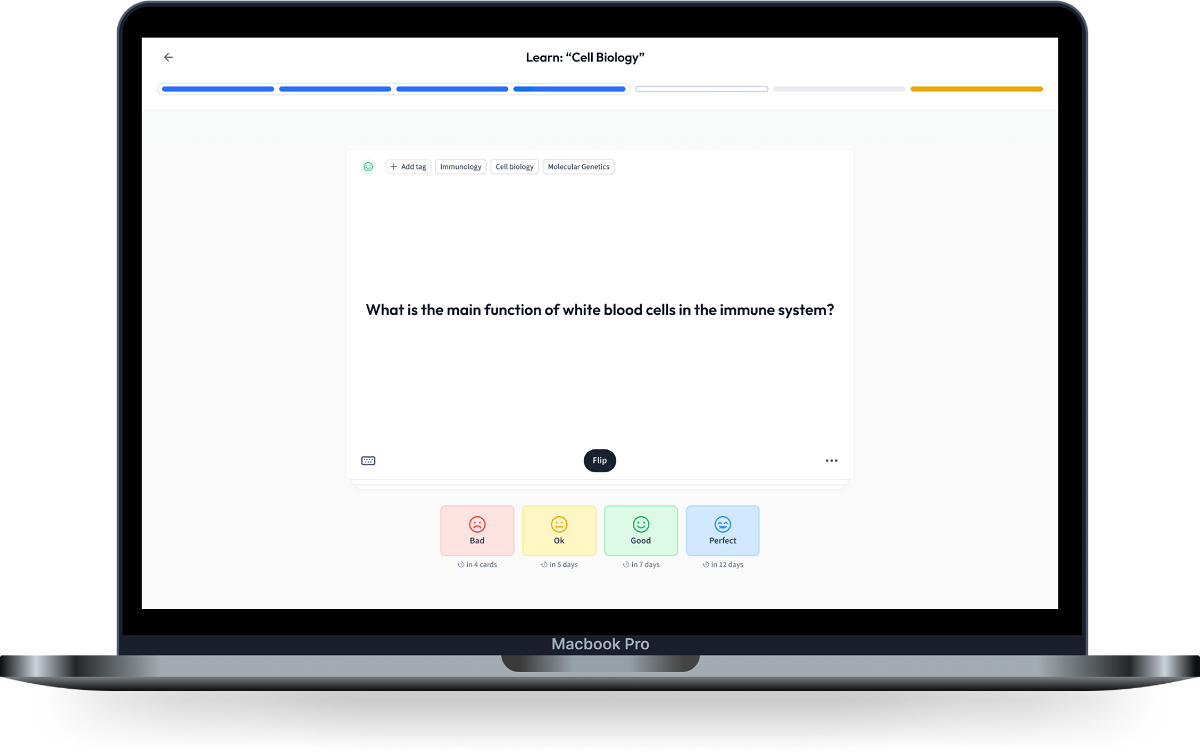
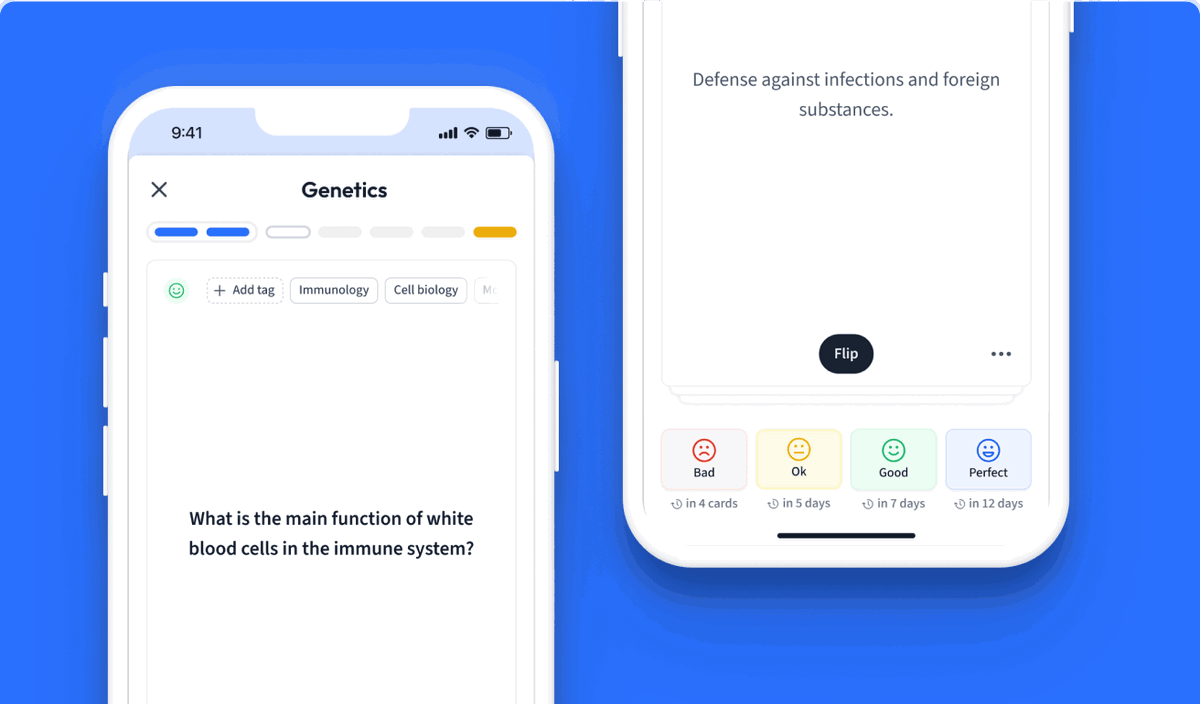
Learn with 15 Modifying Data in SQL flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Modifying Data in SQL
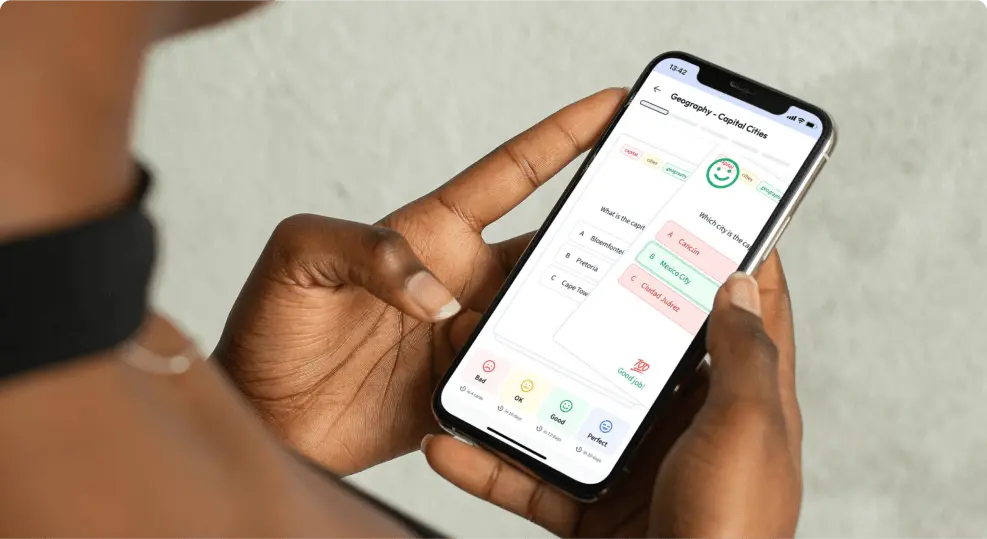
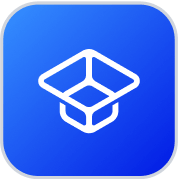
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more