Looping in SQL Explained: The Basics
Looping is a fundamental concept in most programming languages, allowing tasks to be repeated multiple times based on certain conditions. Similarly, looping in SQL is the process of repeating a set of SQL statements until a specific condition is met. It enables developers to automate repetitive tasks such as updating data or iterating through a result set. There are different types of loops available in SQL, including:
- WHILE loops
- FOR loops (specific to certain databases)
- Cursor-based loops
A WHILE loop, as the name suggests, repeats a block of code while a given condition remains true. This type of loop is commonly used in SQL scripting when the number of iterations is unknown or depends on a certain query result.
FOR loops, on the other hand, iterate through a predefined range of values or a specified number of times. However, it should be noted that FOR loops are not universally available in all SQL dialects and might be specific to certain databases such as PostgreSQL or Oracle.
Lastly, cursor-based loops are designed to iterate through rows in a result set fetched by a query. They are especially useful when working with large datasets, as they offer row-by-row processing, avoiding the need to load the entire dataset into memory.
The Importance of Looping in SQL
Looping in SQL plays an essential role in many database operations, enabling developers to perform a variety of tasks more efficiently and automate certain processes. Some examples include:
- Updating records
- Data validation
- Aggregation and summation
- Complex data manipulation
- Procedural code execution
By using loops in SQL, developers gain additional flexibility and control over their database operations, which can lead to improved performance and reduced code complexity.
Looping in SQL Example: Step-by-Step Guide
In this section, we will go through a step-by-step example of using a WHILE loop in SQL to demonstrate how looping can be utilised in practice. The following example assumes that you have a SQL table named 'employees' with the following columns: 'employee_id', 'salary', and 'bonus'. You want to give a 5% bonus to all employees who earned less than £50,000 in the past year.
Step 1: Write a SQL query to calculate the bonus amount for each eligible employee:
UPDATE employees
SET bonus = salary * 0.05
WHERE salary < 50000;
Step 2: In order to use a WHILE loop, we will first need a starting point. To get the minimum employee_id in the table, use the following query:
SELECT MIN(employee_id) AS min_id
FROM employees;
Step 3: Next, we will create a stored procedure that utilises the WHILE loop for this specific task:
CREATE PROCEDURE UpdateEmployeeBonus()
AS
BEGIN
DECLARE @current_id INT;
SELECT @current_id = MIN(employee_id) FROM employees;
WHILE @current_id IS NOT NULL
BEGIN
UPDATE employees
SET bonus = salary * 0.05
WHERE employee_id = @current_id
AND salary < 50000;
SELECT @current_id = MIN(employee_id)
FROM employees
WHERE employee_id > @current_id;
END;
END;
Step 4: Lastly, execute the stored procedure to update bonuses:
EXEC UpdateEmployeeBonus;
This example demonstrates how you can efficiently use looping in SQL to update records based on certain conditions. It is important to note that this is just one example, and looping can be applied in various other contexts to automate tasks and make your SQL code more efficient and manageable.
SQL Looping Techniques
FOR loops in SQL are used to execute a specific block of code a predetermined number of times or through a given range of values. It is worth noting that not all SQL dialects support FOR loops, with their syntax and usage varying between different database systems such as PostgreSQL and Oracle.
In PostgreSQL, the syntax for using FOR loops is as follows:
DO $$
DECLARE
counter INTEGER;
BEGIN
FOR counter IN 1..10
LOOP
-- Your SQL code here
END LOOP;
END;
$$;
In this example, the loop iterates from 1 to 10, and you can place your desired SQL code within the loop. Each iteration will execute the code specified inside the loop.
Meanwhile, in Oracle, the FOR loop syntax looks like this:
BEGIN
FOR counter IN 1..10
LOOP
-- Your SQL code here
END LOOP;
END;
/
The Oracle syntax is similar to PostgreSQL's, with the key difference being that the loop declaration is directly within the BEGIN and END statements, and the delimiter at the end of the block is '/'.
Pros and Cons of Using For Loops in SQL Statements
For loops in SQL can prove beneficial in various situations, but their applicability and usefulness also come with some limitations. Here's a rundown of the main pros and cons:
Pros:
- For loops provide a straightforward way to iterate through a specified range of values, allowing you to better control the number of iterations.
- They can simplify complex queries by breaking them down into smaller, more manageable tasks.
- For loops enable better optimisation of certain calculations, such as aggregate functions over a large dataset.
Cons:
- Not all SQL dialects support FOR loops, limiting their use across different database systems.
- Heavy use of loops can lead to performance issues, as SQL is primarily designed for set-based operations instead of procedural programming.
- It might be more challenging to work with row-by-row processing in FOR loops compared to other means, such as using a cursor.
While Loop in SQL: Creating Iterative Procedures
While loops in SQL provide you with the ability to execute a block of code repeatedly as long as a specific condition remains true. Contrary to FOR loops, WHILE loops are available across most SQL dialects, such as SQL Server, MySQL, and PostgreSQL, with minor differences in syntax. The condition is checked before each iteration, and if the condition is met, the loop continues.
Here's an example of a WHILE loop in SQL Server:
DECLARE @counter INT = 1;
WHILE @counter <= 10
BEGIN
-- Your SQL code here
SET @counter = @counter + 1;
END;
This example shows a WHILE loop that would iterate 10 times, executing the specified SQL code within the BEGIN and END statements for each iteration.
Similarly, a WHILE loop in PostgreSQL can be implemented using the following syntax:
DO $$
DECLARE
counter INTEGER := 1;
BEGIN
WHILE counter <= 10
LOOP
-- Your SQL code here
counter := counter + 1;
END LOOP;
END;
$$;
Again, the loop iterates 10 times, executing the specified SQL code within the LOOP and END LOOP statements for each iteration.
When to Choose While Loop in SQL
While loops are particularly useful in cases where the number of iterations depends on a condition and is unknown in advance. However, it is essential to be aware of the circumstances in which WHILE loops are most effective and appropriate. Here are some scenarios in which using a WHILE loop in SQL can be advantageous:
- When the number of iterations depends on the result of a query or a dynamic condition.
- When updating or modifying records based on certain criteria, such as applying discounts or tax rates.
- If you need to carry out row-by-row processing, especially when handling a large result set with significant memory constraints.
- When performing data validation or data cleansing on a record-by-record basis.
In summary, WHILE loops offer a valuable tool for handling tasks in SQL that require iterative procedural execution. However, it is crucial to remember that SQL is designed for set-based operations, and relying excessively on loops can hinder performance. Always evaluate the trade-offs and consider alternative approaches, such as using set-based operations or window functions, whenever possible.
Advanced Looping in SQL
As your SQL skills grow, so too does your ability to perform complex looping operations. It's essential to keep improving these techniques to maximise efficiency and performance in large-scale tasks. This section will cover advanced looping methods and scenarios that demand more than just simple WHILE or FOR loops.
Efficient Looping Techniques for Large Databases
Dealing with large databases often requires the usage of efficient looping techniques that maintain optimal performance. Here are some key strategies to improve your looping capabilities when working with substantial amounts of data:
- Batch processing: Updating millions of rows all at once could lead to a performance slowdown. Instead, you can process data in smaller batches, which significantly reduces the impact on the database's overall performance. Use a loop to go through the dataset in defined intervals and update the data jTable by jTable. This gives you more control over the resources consumed by the operation.
- Optimising cursors: While cursors are considered an expensive option for row-by-row processing, they can be advantageous in certain situations with large datasets. You can optimise cursors by reducing the number of fetches and filtering the dataset fetched by the cursor to only those rows that truly require processing.
- Parallelism: If your database system allows for parallel execution, you can leverage it to speed up looping through large datasets. It's essential to manage server resources effectively and ensure parallelism doesn't lead to contention issues.
- Indexing and query optimisation: When looping through large datasets, consider improving index usage and optimising queries for enhanced performance. Proper indexing can significantly reduce the number of loops, and a well-executed query will minimise the overhead throughout the loop iterations.
Batch processing is an optimisation technique that allows you to divide large tasks into smaller units (batches) and process them sequentially or concurrently. By doing so, you can better manage server resources, improve throughput, and reduce the impact on overall database performance.
Best Practices for Looping in SQL Database Management
To get the most out of your looping operations in SQL, it's crucial to follow best practices. Here are some recommendations to help you effectively manage looping in SQL:
- Avoid unnecessary loops: SQL is primarily designed for set-based operations. Whenever possible, opt for set-based solutions instead of relying on loops, especially for large datasets.
- Choose the right loop type: As previously mentioned, WHILE loops are suitable for cases where the number of iterations is unknown or relies on a certain condition. FOR loops are used when the number of iterations is predetermined. Choose the appropriate loop type based on your requirements.
- Test and optimise: Continuously review your looping code, performing tests to ensure it remains efficient and checking for potential bottlenecks. Be sure to test your code thoroughly, particularly when updating or deleting data, as error recovery may prove challenging.
- Minimise lock contention: When performing looping operations, ensure that lock contention is reduced to avoid related performance issues. You can do so by implementing efficient database transaction strategies and leveraging row-versioning techniques.
- Use transactions wisely: When dealing with looping constructs that perform data manipulation, implement transactions to ensure data consistency and integrity. Be cautious when using transactions, as they can also lead to lock contention or blocking problems if not managed correctly.
- Error handling and recovery: Implement robust error handling within your loops to gracefully handle unexpected errors and prevent data corruption. Ensure your looping code can recover from errors or rollback to a consistent state without jeopardising database integrity.
Looping in SQL - Key takeaways
Looping in SQL is the process of repeating a set of SQL statements until a specific condition is met.
Types of loops in SQL include WHILE loops, FOR loops, and cursor-based loops.
While loops execute code repeatedly as long as a specific condition remains true.
FOR loops iterate through a predefined range of values or a specified number of times (not universally available in all SQL dialects).
Advanced looping techniques include batch processing, optimising cursors, parallelism, and indexing and query optimisation.
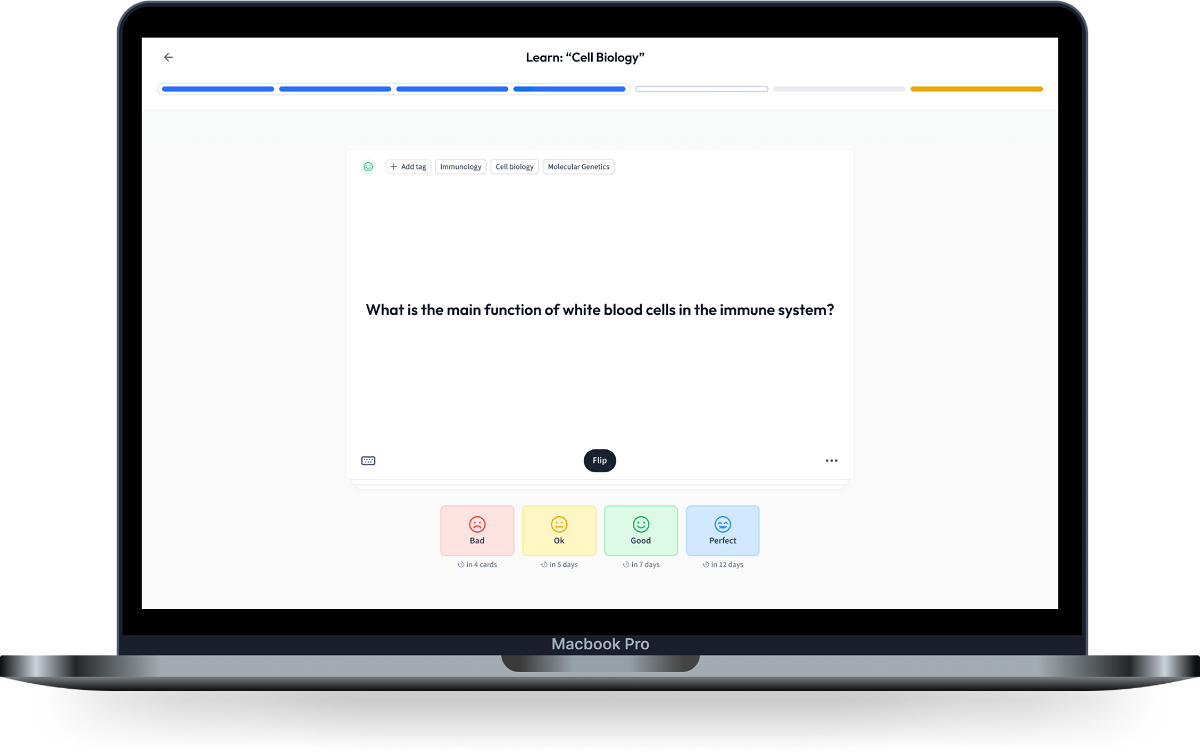
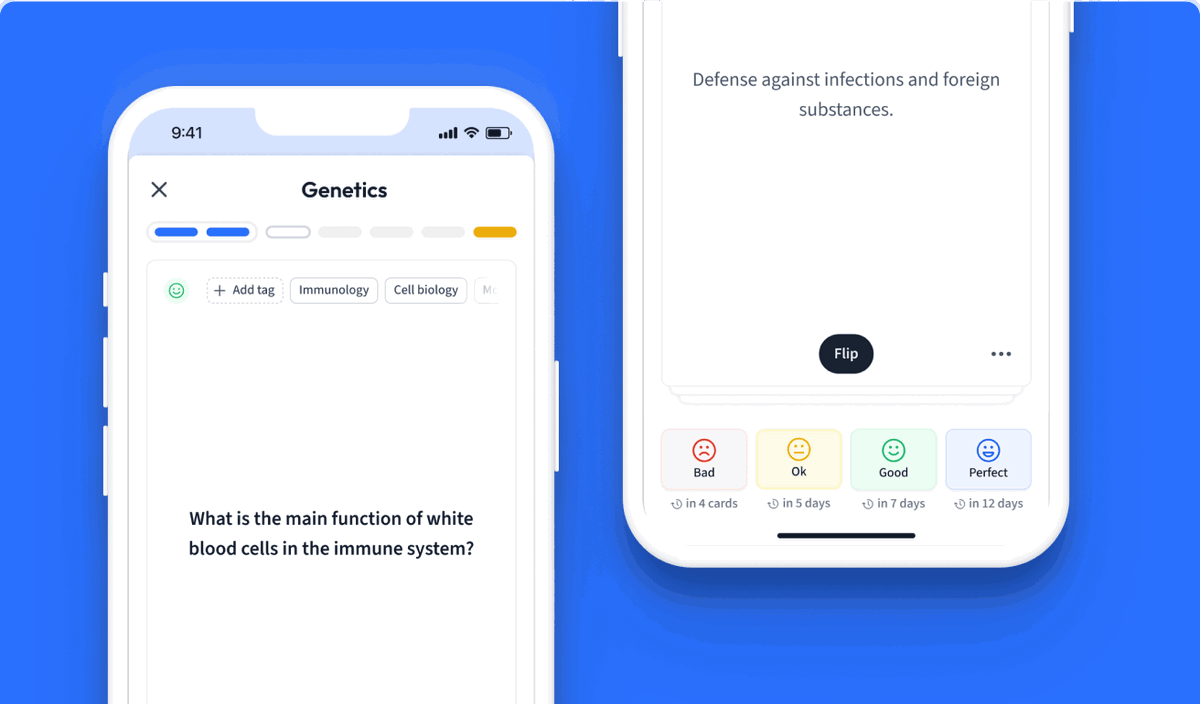
Learn with 14 Looping in SQL flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Looping in SQL
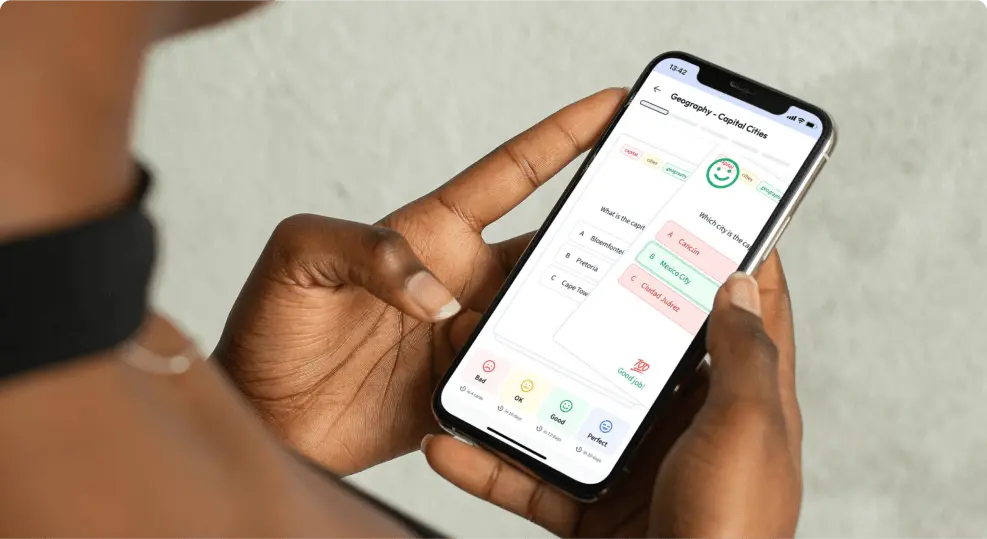
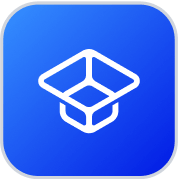
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more