Understanding SQL Subquery
To work efficiently with databases, it is essential to understand SQL Subquery. It is a powerful technique in the SQL language, which allows you to retrieve and manipulate data more effectively. In this article, you will get a comprehensive understanding of the SQL Subquery, its applications, and its functionalities. You will also learn about the advantages of using SQL Subquery in database management.
SQL Subquery Explained: Basics and Applications
A SQL Subquery is a query that is embedded within another query, often referred to as the main query or outer query. A subquery is enclosed in parentheses and can be used in various parts of a SQL statement, such as the SELECT, FROM, WHERE, and HAVING clauses, to produce intermediate results for the main query.
Subqueries can be classified into different types, which mainly differ based on their position and the number of rows and columns returned. These types include:
- Scalar Subquery: returns a single value (one row and one column)
- Row Subquery: returns a single row with multiple columns
- Column Subquery: returns multiple rows with a single column
- Table Subquery: returns multiple rows and columns
Subqueries can be used in various real-world applications, such as:
- Data filtering: by using subqueries in the WHERE or HAVING clause
- Data manipulation: with INSERT, UPDATE, and DELETE statements containing subqueries
- Data aggregation and calculation: by employing subqueries in the SELECT or FROM clause
- Joining tables: by using subqueries in place of regular table names in the FROM clause
SQL Subquery Functions: Aggregating and Filtering Data
Subqueries are extremely useful when it comes to aggregating and filtering data in SQL. Here are some ways subqueries can be employed for these purposes:
- Calculating average, sum, or count based on a specific condition
- Filtering out rows that do not meet specific criteria
- Selectively importing data from other tables
- Finding the maximum or minimum value in a specific situation
For example, imagine you have a table of students with their scores in different subjects. You want to find the average score of students who scored more than 80 points in Mathematics. You can use a subquery in the WHERE clause to filter out the students:
SELECT AVG(score) FROM students WHERE student_id IN ( SELECT student_id FROM scores WHERE subject = 'Mathematics' AND score > 80 );
Advantages of Using SQL Subquery in Database Management
Incorporating SQL subqueries into your database management processes provides several benefits:
- Enhanced Readability: Subqueries can make your SQL code more readable and easier to understand by breaking down complex queries into smaller, more manageable parts.
- Increased Flexibility: Subqueries allow you to use the results of one query as input for another, which gives you more control over your database queries and access to intermediate results.
- Improved Performance: In some cases, using subqueries can offer better performance compared to using joins or temporary tables, especially when dealing with smaller datasets or when properly optimized by the database management system.
- Simplified Query Logic: Subqueries can help simplify the overall structure of your SQL statements by reducing the need for multiple table joins and eliminating the use of intermediary results or complex calculations.
Though SQL Subqueries offer several advantages, it is important to note that their efficient use largely depends on the database management system, the complexity of the query, and the dataset size. In some cases, using subqueries may result in suboptimal performance, so it is necessary to consider the trade-offs between readability, flexibility, performance, and simplification while incorporating SQL Subqueries into your database management strategies.
SQL Subquery Examples and Use Cases
A deeper understanding of SQL Subquery requires practical examples and use cases. In the following sections, you will explore how SQL subqueries can be used in SELECT statements, enhance queries using the WHERE clause, and combine tables with subquery joins for complex results.
SQL Subquery in SELECT Statement: Practical Examples
Using subqueries in the SELECT statement allows you to compute and display calculations or other intermediate results based on data from your database. Consider the following practical examples:
- Using subquery to compute the total order value: If you have a database containing order items with product IDs, quantities, and unit prices, you can compute the total value of each order using a subquery:
SELECT order_id, (SELECT SUM(quantity * unit_price) FROM order_items WHERE order_items.order_id = orders.order_id ) AS total_value FROM orders;
- Calculating sales percentage: For a table containing sales data, you can use a subquery to calculate the percentage of total sales each product represents:
SELECT product_id, sales_amount, sales_amount * 100 / (SELECT SUM(sales_amount) FROM sales) AS sales_percentage FROM sales;
Enhancing Queries with SQL Subquery in WHERE Clause
When working with a large dataset, filtering data using subqueries in the WHERE clause can help you enhance and focus your queries. Consider the following use cases:
- Finding customers with high-value orders: If you have a database containing customer and order information, you could use a subquery to identify customers with orders above a certain threshold:
SELECT customer_id, customer_name FROM customers WHERE customer_id IN ( SELECT customer_id FROM orders WHERE total_value > 1000 );
- Filtering products based on category ratings: If you have a table with product reviews and ratings, a subquery in the WHERE clause can help filter products based on their average rating and category:
SELECT product_id, product_name FROM products WHERE category = 'Electronics' AND product_id IN ( SELECT product_id FROM reviews GROUP BY product_id HAVING AVG(rating) > 4 );
SQL Subquery Joins: Combining Tables for Complex Results
SQL subquery joins make it possible to combine tables and retrieve complex results more efficiently, especially when dealing with multiple table relationships. Examine the following practical examples:
- Calculating employee performance metrics: When you have separate tables containing employee data and their performance scores, you can use subquery joins to calculate performance metrics comparing the average employee performance with each employee's score:
SELECT employees.employee_id, employees.employee_name, scores.performance_score, (SELECT AVG(performance_score) FROM scores) AS avg_performance FROM employees INNER JOIN scores ON employees.employee_id = scores.employee_id WHERE scores.performance_score >= (SELECT AVG(performance_score) FROM scores);
- Finding products with supplier-provided discounts: If you have tables containing product and supplier data, along with supplier-provided discounts, you can use subquery joins to list products eligible for discounts and the supplier information:
SELECT products.product_id, products.product_name, suppliers.supplier_id, suppliers.supplier_name FROM products INNER JOIN ( SELECT supplier_id, product_id FROM discounts WHERE discount_amount > 0 ) AS discounted_products ON products.product_id = discounted_products.product_id INNER JOIN suppliers ON suppliers.supplier_id = discounted_products.supplier_id;
These examples demonstrate how you can leverage SQL Subquery to enhance your database management abilities. By using subqueries in SELECT statements, WHERE clauses, and joining tables with subquery joins, you can achieve powerful results while maintaining readable and flexible code.
Mastering SQL Subquery Techniques and Best Practices
As you continue to develop your SQL Subquery skills, mastering advanced techniques and adopting best practices can help you make your SQL code more efficient, maintainable, and readable. In this section, we will discuss tips for optimising SQL Subquery performance and common pitfalls to avoid for optimal results.
Tips for Optimising SQL Subquery Performance
To ensure peak performance of your SQL Subqueries, consider the following tips and guidelines that can help reduce execution time and resource consumption in your database management systems:
- Limit the number of returned rows: When working with large datasets, limit the number of rows returned by your subquery using the SQL clauses such as LIMIT or TOP.
- Use appropriate indexes: Create appropriate indexes on the columns used in the subquery to speed up data retrieval and improve performance.
- Filter data efficiently: Apply filtering conditions in your subquery whenever possible, rather than in the main query, to reduce the number of records that need to be processed from the start.
- Consider alternatives: In certain situations, joins and derived tables can offer better performance compared to subqueries. Evaluate the specific requirements of your query and decide accordingly.
- Nested subqueries: Although nested subqueries can provide advanced functionality, they may have a negative impact on performance. Avoid excessive nesting, and opt for more simplified solutions if possible.
- Aggregate data wisely: Use aggregate functions, such as AVG(), COUNT(), and SUM(), in conjunction with the GROUP BY clause to minimize the amount of data that the database management system has to process.
- Optimise the subquery type: Choose the most suitable subquery type (scalar, row, column, or table subquery) based on your specific use case to increase efficiency.
- Reuse redundant subqueries: If several subqueries return the same result, consider creating a common table expression (CTE) or temporary table to compute the result once and reuse it multiple times.
Common Pitfalls and How to Avoid Them in SQL Subquery
When working with SQL subqueries, be aware of the following common pitfalls and learn how to avoid them to ensure your code remains efficient and readable:
- Using correlated subqueries when not necessary: Correlated subqueries can cause poor performance, as they are executed for each row of the main query. If possible, use non-correlated subqueries to avoid unnecessary performance degradation.
- Ignoring NULL values: When dealing with NULL values, use proper NULL handling functions or techniques to ensure accurate query results. Avoid using '=' or '<>' directly, and instead use the SQL functions: COALESCE(), NULLIF(), IS NULL, or IS NOT NULL.
- Misusing aggregate functions: Aggregate functions such as COUNT(), AVG(), and SUM() should be used carefully within subqueries, especially when combined with GROUP BY, as they can return misleading or incorrect results.
- Ignoring the order of execution: Keep in mind that the subquery is executed before the main query. Make sure that your subquery returns the expected result set before it's used by the outer query.
- Overusing IN and NOT IN operators: While these operators can be helpful in certain contexts, they can also lead to performance issues. Consider alternative approaches such as EXISTS, NOT EXISTS, or JOINS in these situations.
By mastering SQL Subquery techniques and best practices, avoiding common pitfalls, and optimising your SQL code for performance, you can ensure that your database management tasks are efficient, maintainable, and produce accurate results.
SQL Subquery - Key takeaways
SQL Subquery: A query embedded within another query (main or outer query) to produce intermediate results.
Subquery types: Scalar, Row, Column, and Table Subqueries.
Applications: Data filtering, data manipulation, data aggregation, and joining tables.
Advantages: Enhanced readability, increased flexibility, improved performance, and simplified query logic.
Techniques and best practices: Optimising performance, avoiding common pitfalls, and applying proper NULL handling.
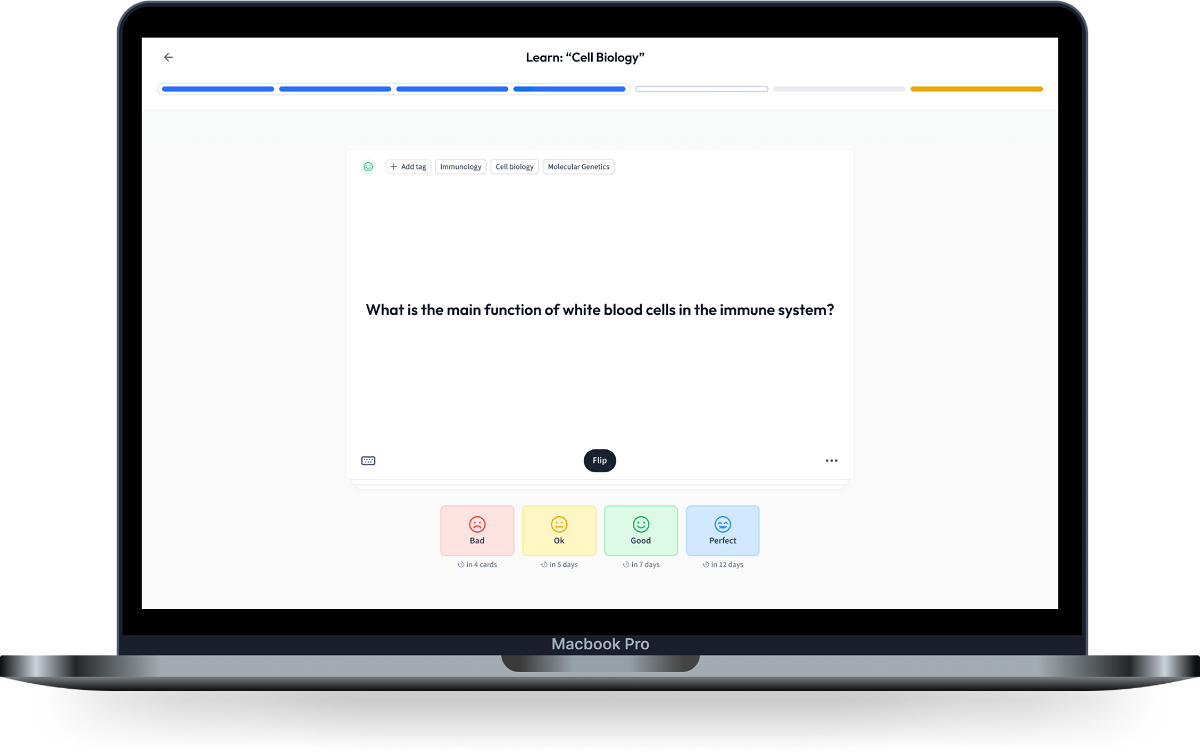
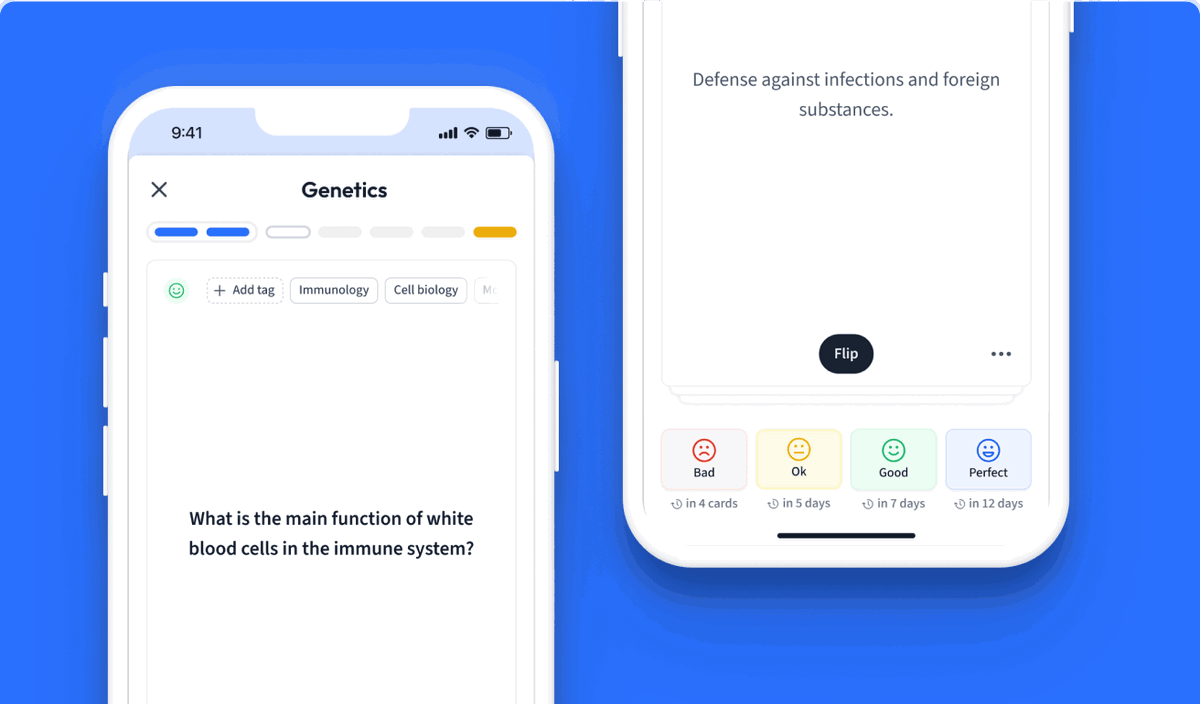
Learn with 15 SQL Subquery flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about SQL Subquery
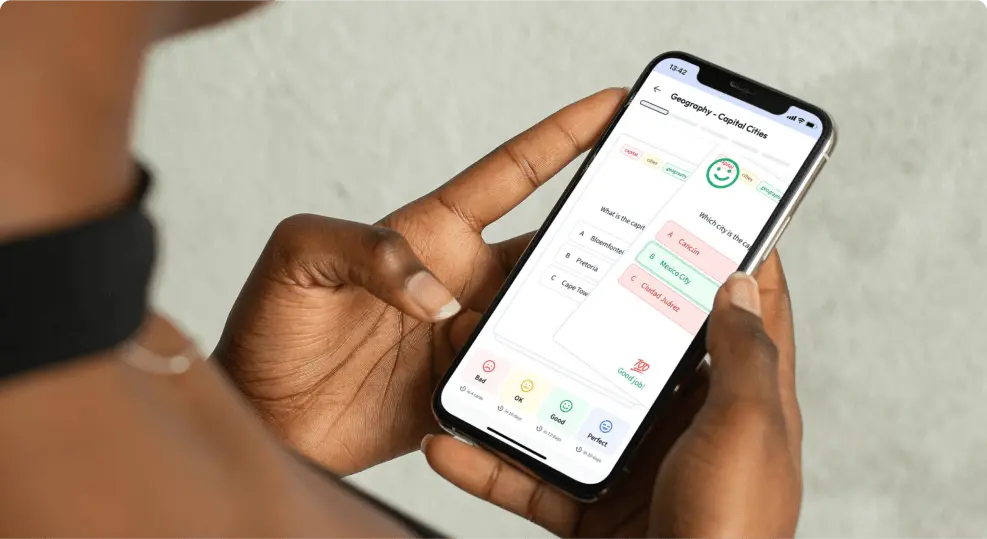
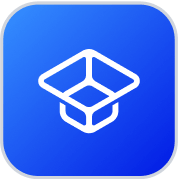
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more