Understanding the SQL CHECK Clause in Computer Science
In computer science and database management, the SQL CHECK clause plays a significant role in maintaining data integrity within databases. A type of constraint, the SQL CHECK clause ensures that the data entered into a specific column or set of columns adheres to a specified condition or logical expression. This article will delve into its definition, implementation in databases, and explore its syntax and usage in detail.
Definition and Purpose of SQL CHECK Constraint
The SQL CHECK constraint is used in table definitions to enforce a particular condition being met when data is being inserted or updated within a field or record of a table. It essentially ensures that the data entered aligns with the defined rules, helping to maintain data integrity. When data doesn't meet a CHECK condition, the attempt to insert or update the data in the column returns an error.For instance, consider a table that stores information about the age of employees in an organisation. A CHECK constraint can be implemented to limit the allowed age to a specifically defined range (e.g., the age must be between 18 and 65).
Implementing SQL CHECK Constraint in Databases
The SQL CHECK constraint can be applied to a column or a table as a whole during the creation or modification of a table using the CREATE TABLE and ALTER TABLE statements respectively. Here is an example of incorporating a SQL CHECK constraint during table creation:CREATE TABLE employees ( employee_id INTEGER, name VARCHAR(50), age INTEGER CHECK (age >= 18 AND age <= 65) );
CREATE TABLE employees ( employee_id INTEGER, name VARCHAR(50), age INTEGER, salary DECIMAL(10, 2), CHECK (age >= 18 AND age <= 65 AND salary >= 10000) );
ALTER TABLE employees ADD CHECK (age >= 18 AND age <= 65 AND salary >= 10000);
SQL CHECK Syntax and Usage
The general syntax for implementing the SQL CHECK constraint in a table is as follows: 1. Adding constraint during table creation:CHECK(column_name condition) for column-level constraints
or
CHECK (multiple_conditions) for table-level constraints
ALTER TABLE table_name ADD CHECK (multiple_conditions)
CREATE TABLE employees ( employee_id INTEGER NOT NULL CHECK (employee_id > 0), name VARCHAR(50), age INTEGER CHECK (age >= 18 AND age <= 65), salary DECIMAL(10, 2) CHECK (salary >= 10000) );
Examples of SQL CHECK in Database Management
SQL CHECK is a widely used constraint in database management systems to ensure data consistency and integrity. This section will provide basic examples for beginners, demonstrate common use cases of the SQL CHECK constraint, and explore advanced applications in database operations.Basic SQL CHECK Example for Beginners
As a beginner, it is essential to start with a simple example to understand the basic implementation of the SQL CHECK constraint. The following example demonstrates how to use the SQL CHECK constraint for a "students" table. Imagine having a table that stores information about the students in a school, including their grade and age. You can utilize the SQL CHECK constraint to make sure that the grade falls within a valid range (e.g., between 1 and 12) and that the age is appropriate for each grade (e.g., between 5 and 18).CREATE TABLE students ( student_id INTEGER, name VARCHAR(50), grade INTEGER CHECK (grade >= 1 AND grade <= 12), age INTEGER CHECK (age >= 5 AND age <= 18) );
Common Use Cases of SQL CHECK Constraint
SQL CHECK constraints have various applications, ensuring data consistency and integrity across different scenarios. Some common use cases include: 1. Ensuring a minimum and maximum value: - For example, setting a minimum and maximum allowed age for employees in an organisation. - Ensuring the salary is within an acceptable range. 2. Defining valid date ranges for records: - Verifying that a start date is earlier than an end date. - Ensuring dates fall within a specific period or duration. 3. Preserving referential integrity for relationships: - Addressing scenarios where foreign key constraints are not sufficient to ensure data integrity. - Validating that values in a column or columns have a specific association with values in other columns within the same table or in a different table. 4. Enforcing business rules: - Utilizing SQL CHECK constraints to ensure that certain conditions are satisfied, such as discounts on items or valid working hours. 5. Validating inputs against domain-specific constraints: - Enforcing that text fields contain valid email addresses or phone numbers. - Checking the format of text fields, such as credit card numbers or serial numbers. These examples showcase the versatility of SQL CHECK constraints and demonstrate their utility in maintaining data integrity across a wide range of scenarios.Advanced Applications of SQL CHECK in Database Operations
As you progress in your understanding of SQL CHECK constraints, you may encounter more complex scenarios that require advanced applications. Some of these advanced applications include: 1. Combining multiple constraints:CREATE TABLE employees ( employee_id INTEGER, name VARCHAR(50), age INTEGER, salary DECIMAL(10, 2), CHECK ((age >= 18 AND age <= 65) AND (salary >= 10000 AND salary <= 100000)) );
CREATE TABLE projects ( project_id INTEGER, name VARCHAR(50), start_date DATE, end_date DATE, CHECK ((DATEDIFF(day, start_date, end_date) >= 7) AND (YEAR(end_date) <= 2025)) );
In this example, the CHECK constraint utilises the "DATEDIFF" and "YEAR" functions to determine the duration between the start and end dates and to ensure that the end date does not go beyond a specific year. As you gain more experience working with SQL CHECK constraints, you will also learn the importance of optimizing these constraints for better database performance and dealing with potential trade-offs between enforcing strict data integrity and maintaining efficient databases.
SQL CHECK vs Other SQL Constraints in Computer Science
Understanding the differences between SQL CHECK and other SQL constraints is crucial to managing databases effectively. In this section, we will compare SQL CHECK with other essential SQL constraints, such as PRIMARY KEY, FOREIGN KEY, and UNIQUE constraints, to grasp their unique functions, applications, and effects on databases.Comparing SQL CHECK with SQL PRIMARY KEY
Both SQL CHECK and SQL PRIMARY KEY enforce specific rules to maintain data integrity within a database. However, they have different purposes and implementations: SQL CHECK: - Defines a condition that must be satisfied for the column(s) data values. - Ensures that data follows certain business rules or limitations. - Can be placed on one or multiple columns. SQL PRIMARY KEY:- Uniquely identifies each record in a table. - A table can have only one PRIMARY KEY constraint. - A PRIMARY KEY constraint cannot contain NULL values. Let's examine their functionalities by comparing their applications in a table:CREATE TABLE employees ( employee_id INTEGER PRIMARY KEY, -- PRIMARY KEY constraint on employee_id name VARCHAR(50), age INTEGER, salary DECIMAL(10, 2), CHECK (age >= 18 AND age <= 65) -- CHECK constraint on age column );
Understanding SQL CHECK and SQL FOREIGN KEY Constraints
While both SQL CHECK and SQL FOREIGN KEY constraints are instrumental in maintaining data integrity, they serve distinct purposes: SQL CHECK: - Ensures that a column or multiple columns satisfy specific conditions. - Validates data values based on the specified rule(s). SQL FOREIGN KEY:- Maintains referential integrity between tables. - Ensures that the value in a column or set of columns matches the values in a PRIMARY KEY or UNIQUE constraint in another table. - Helps to create parent-child relationships between tables. To compare their functionalities, consider their applications in two tables "employees" and "departments":CREATE TABLE departments ( department_id INTEGER PRIMARY KEY, department_name VARCHAR(50) NOT NULL ); CREATE TABLE employees ( employee_id INTEGER PRIMARY KEY, name VARCHAR(50), age INTEGER CHECK (age >= 18 AND age <= 65), -- CHECK constraint on age column department_id INTEGER REFERENCES departments(department_id) -- FOREIGN KEY constraint on department_id );
SQL CHECK vs SQL UNIQUE Constraint: Key Differences
SQL CHECK and SQL UNIQUE constraints both, in their own way, contribute to maintaining data integrity within a database, though they serve different purposes: SQL CHECK: - Enforces a specific condition or rule for the data values of one or multiple columns. SQL UNIQUE:- Ensures that unique data values are maintained within the specified column(s). - Can have NULL values, so it can also be used to enforce uniqueness without preventing NULL entries (unlike a PRIMARY KEY constraint). To differentiate their functionalities, let's observe their application in a table:CREATE TABLE employees ( employee_id INTEGER PRIMARY KEY, name VARCHAR(50), email VARCHAR(100) UNIQUE, -- UNIQUE constraint on the email column age INTEGER CHECK (age >= 18 AND age <= 65) -- CHECK constraint on age column );
In this example, the UNIQUE constraint on the "email" column ensures that each employee has a unique email address, while the CHECK constraint on the "age" column validates that employee ages fall within the defined range (18 to 65). By understanding the similarities and differences between SQL CHECK and other SQL constraints, you can make the best decision on which constraint to use in various scenarios, ensuring data integrity and consistency within databases.
Best Practices for Using SQL CHECK in Computer Science Education
Many students learning computer science, especially those focusing on database management, will benefit from understanding how to use the SQL CHECK constraint effectively. It can be a crucial aspect for data integrity and consistent data storage in databases. This section will discuss best practices related to SQL CHECK usage, including achieving data integrity, avoiding common mistakes, and tips for efficient database management.Ensuring Data Integrity with SQL CHECK Constraint
To maintain a high level of data integrity with the SQL CHECK constraint, consider the following best practices: Use descriptive names for constraints: Give your SQL CHECK constraints names that accurately describe the condition being imposed. This can make understanding their purpose easier for others working on the database. Choose the right constraint level: Define the SQL CHECK constraint at column-level for single column conditions, and at table-level for more complex multi-column conditions. Be concise and clear with conditions: Keep the conditions simple and easy to understand, reducing complexity where possible. Use clear and descriptive logical expressions. Provide adequate error messages: Implement custom error messages that display information about which constraint failed and why. These messages can ease troubleshooting and debugging. Keep performance in mind: Remember that SQL CHECK constraints can affect query performance. Make sure to optimize your constraints to reduce the impact on performance.Common Mistakes to Avoid with SQL CHECK Implementations
When working with SQL CHECK constraints, it's important to be aware of common mistakes, to avoid potential issues:Overlapping conditions: Avoid creating multiple CHECK constraints that unnecessarily overlap. This not only causes confusion but can also impact query performance. Missing edge cases: Ensure that your CHECK constraint covers all relevant scenarios that are critical to your business requirements. Carefully analyze edge cases to avoid introducing security or data integrity issues. Using NOT NULL instead of CHECK: When the purpose of a constraint is to prevent NULL values in a column, use the NOT NULL constraint instead of the CHECK constraint for better performance and clarity. Ignoring transactional control: Be mindful of other operations that might trigger updates or alterations to the data. Implement proper transactional controls to guarantee data consistency during multiple operations. Applying the CHECK constraint on an incorrect data type: Be cautious about constraints that involve data type conversions or might cause conflicts when dealing with different data types or character sets.Tips for Efficient Database Management Using SQL CHECK
Implementing SQL CHECK constraints effectively can lead to more efficient database management. The following tips will help improve your database operations: Regularly review and update CHECK constraints: To maintain the effectiveness of your constraints, review them as your business requirements evolve and update or refine them when necessary. Use database vendor tools: Take advantage of the tools provided by your database vendor to help optimize your constraints and manage query performance more effectively. Balance strictness and flexibility: Strive to create constraints that are strict enough to protect data integrity, but also flexible enough to allow for future updates and changes to the database schema. Perform thorough testing: Test your constraints extensively to ensure they fulfill their intended purpose and do not impose unintended restrictions on data. Document constraints and their purpose: Properly documenting your SQL CHECK constraints, including their conditions and the rationale behind them, can make it easier for other team members to understand and maintain the database. By applying these best practices, common mistakes avoidance, and efficiency tips, you can improve your expertise in using SQL CHECK constraints in computer science-related fields and maximize the quality and consistency of your database operations.SQL CHECK - Key takeaways
- Definition and Purpose of SQL CHECK Constraint: Ensures data entered in a specific column or set of columns adheres to a specified condition or logical expression, maintaining data integrity within databases.
- Implementing SQL CHECK Constraint in Databases: Can be applied to a column or a table using the CREATE TABLE and ALTER TABLE statements.
- Examples of SQL CHECK in Database Management: Utilized for validating input data against business rules, improving data reliability, and ensuring users must enter specific data meeting the constraints.
- SQL CHECK vs Other SQL Constraints: SQL CHECK is compared to PRIMARY KEY, FOREIGN KEY, and UNIQUE constraint in terms of their unique functions, applications, and effects on databases.
- Best Practices for Using SQL CHECK: Include ensuring data integrity, avoiding common mistakes, and implementing tips for efficient database management.
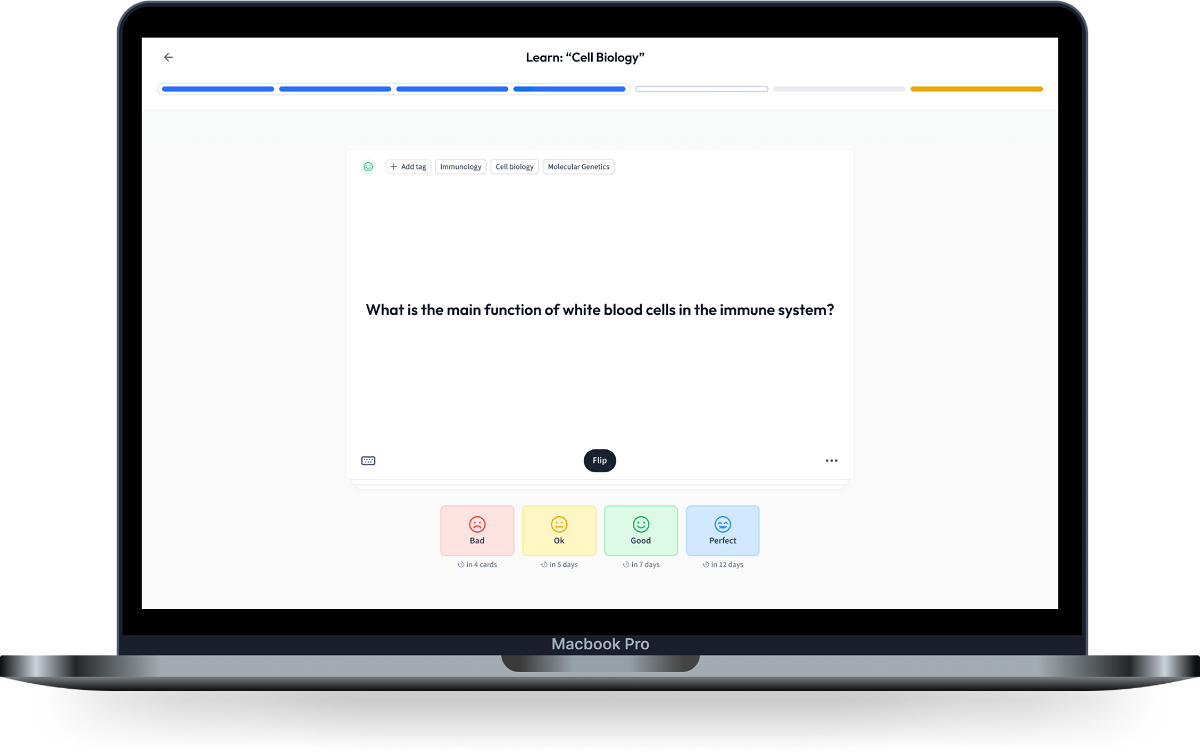
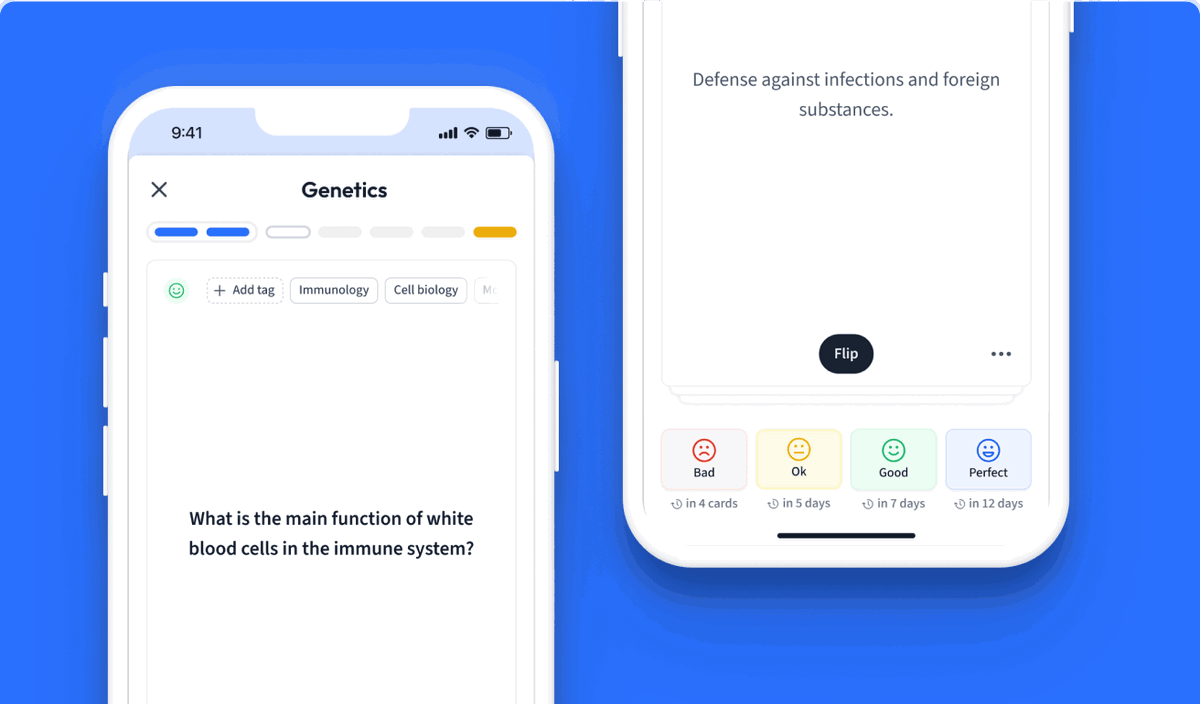
Learn with 16 SQL CHECK flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about SQL CHECK
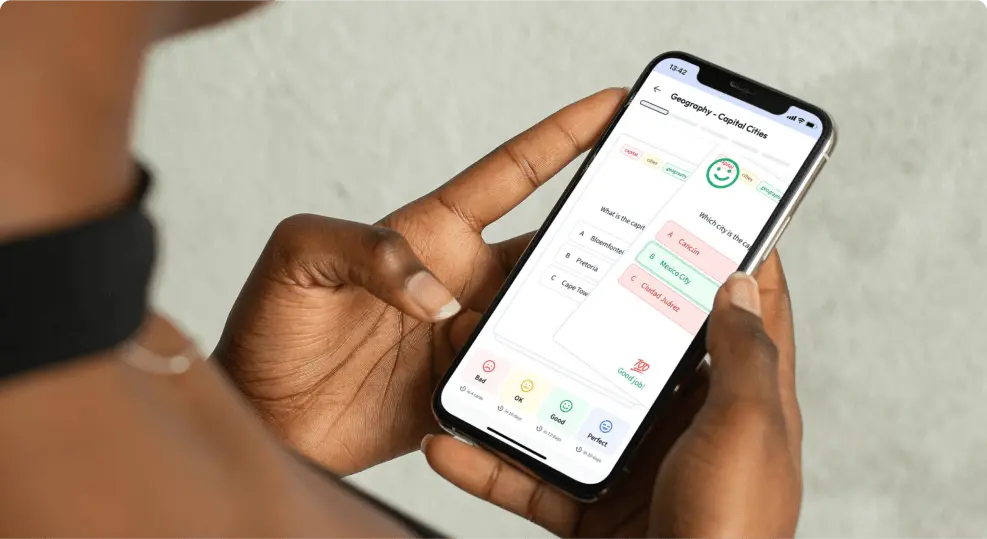
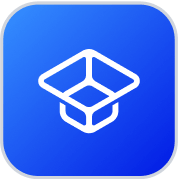
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more