Introduction to Creating Tables in SQL
Creating tables in SQL (Structured Query Language) is a fundamental task for any computer science student or future database administrator. Tables are the essential building blocks for storing and organizing data, which is why understanding how to create tables within SQL is a vital skill. In this article, we will discuss how to establish table structures with SQL data types, create tables in SQL Server Management Studio, and define tables with Identity columns.
Establishing Table Structures with SQL Data Types
When setting up table structures, understanding SQL data types is crucial. These data types determine the type and format of data that can be stored in a table column. This section will cover the essentials of SQL data types and provide some basic information on common data types in SQL Server and Oracle.
Essentials of SQL Data Types
SQL data types are used to define the type of data that can be stored within a table column. They are crucial when creating and altering tables, ensuring that the right data is stored properly, and various operations can be performed on it. Some key points to consider when working with SQL data types are:
- Each column in an SQL table must have a data type.
- There are different data types available depending on the database management system (DBMS) being used. For example, SQL Server and Oracle have their range of data types.
- Choosing the correct data type ensures efficient storage and prevents potential issues when querying the data.
A data type is a classification that specifies which type of value a column can hold in a SQL table.
Common Data Types in SQL Server and Oracle
SQL Server and Oracle are two widely-used DBMS, and each one comes with its own set of data types. Users must be familiar with the specific data types available in their DBMS. In the tables below, we will explore a few common data types in both SQL Server and Oracle.
SQL Server Data Type | Description |
---|---|
VARCHAR | Variable-length character data |
INT | Integer data |
DECIMAL | Exact numeric data |
DATE | Date values |
Oracle Data Type | Description |
---|---|
VARCHAR2 | Variable-length character data |
NUMBER | Numeric data |
DATE | Date and time values |
BLOB | Binary large object data |
Creating Tables in SQL Server Management Studio
SQL Server Management Studio (SSMS) is a graphical user interface designed to manage SQL Server instances and create tables. We will outline the step-by-step guide for creating tables using SSMS in the next section.
Step-by-Step Guide for SQL Server Table Creation
Following these steps, you can create a table in SQL Server Management Studio:
- Open SQL Server Management Studio and connect to an SQL Server instance.
- Expand the Databases folder, and select the desired database.
- Right-click on the 'Tables' folder, and choose 'New Table'.
- In the new table window, enter column names, data types, and set constraints as needed (e.g., primary key, unique).
- Once the desired structure is set, click the 'Save' button, and provide a table name.
- The new table is now created and can be found under the 'Tables' folder in the database.
Note: You can also create a table using SQL commands within the Query Editor in SSMS. Simply write a CREATE TABLE statement using the T-SQL language and execute it accordingly.
Defining SQL Tables with Identity Columns
Identity columns are a useful feature for automatically generating unique identification numbers (IDs) for table rows. In this section, we will discuss when and why identity columns should be utilized.
Utilising Identity Columns: When and Why?
Using Identity Columns is advantageous in situations where you need a unique and automatically incremented value for each row in your table. These auto-generated values work well as primary keys because they prevent duplicate entries, make it easier to manage relationships between tables, and simplify data entry. Here are some scenarios in which you should consider using Identity Columns:
- Tracking individual records with a unique identifier, such as tracking customer IDs.
- When creating relationships between tables, a unique primary key is necessary.
- Preventing duplicate entries and ensuring data integrity across your tables.
To create an Identity column, you include the IDENTITY keyword in your CREATE TABLE statement, followed by the seed and increment values in parentheses. These values represent the starting value and the increment value, respectively.
CREATE TABLE SampleTable
(
Id INT IDENTITY(1,1),
Column1 VARCHAR(50),
Column2 INT
)
In this example, we have an Identity column named 'Id', with a starting value of 1 and incremented by 1 for each new row added to the SampleTable.
Keep in mind that Identity Columns do have their limitations. For instance, adding or modifying Identity values can be complex and lead to unintended consequences. Additionally, they may not be suitable for situations where you require manual control over the unique identification numbers. Always consider your specific use case and requirements before implementing Identity Columns in your tables.
SQL Server vs Oracle: Table Creation Syntax Differences
Both SQL Server and Oracle are widely-used Database Management Systems (DBMS), each with its own unique syntax for creating tables. Understanding the differences between the SQL Server's CREATE TABLE approach and Oracle's CREATE TABLE procedure will ensure a smoother transition between working in these two environments and providing a more comprehensive understanding of their respective functionalities.
Comparing SQL Server's Create Table Approach
SQL Server's table creation approach involves using Transact-SQL (T-SQL) to define the structure of the table and its columns. The syntax differences compared to Oracle include data types, constraints, and special keywords. Here, we explore these differences in detail.
Syntax Differences in SQL Server
When creating tables in SQL Server, you use the CREATE TABLE statement. The syntax for defining the table structure consists of column names, data types, and constraints. Some key aspects of table creation in SQL Server include:
- Data types: SQL Server has a unique set of data types, such as VARCHAR, NVARCHAR, and SMALLINT.
- Constraints: In SQL Server, you can define primary keys, foreign keys, unique keys, and other constraints using the PRIMARY KEY, FOREIGN KEY, UNIQUE, and CHECK keywords respectively.
- Identity columns: Use the IDENTITY keyword for creating identity columns that automatically generate unique values for each row in the table.
Example of SQL Server's CREATE TABLE approach:
CREATE TABLE Employee
(
EmployeeID INT IDENTITY(1,1) PRIMARY KEY,
FirstName VARCHAR(50) NOT NULL,
LastName VARCHAR(50) NOT NULL,
Age INT CHECK(Age>=18),
Email VARCHAR(100) UNIQUE
)
Exploring Oracle's CreateTable Procedure
Oracle's table creation approach, like SQL Server, also uses SQL to define the structure of the table and its columns. However, there are subtle differences in the syntax compared to SQL Server, particularly regarding data types and constraints. Let's delve deeper into the key aspects of table creation in Oracle.
Syntax Differences in Oracle
Oracle uses the CREATE TABLE statement as well when creating new tables. Key aspects of table creation in Oracle include:
- Data types: Oracle's data types are distinct from those of SQL Server. Examples include VARCHAR2, NVARCHAR2, and NUMBER.
- Constraints: Defining primary keys, foreign keys, unique keys, and other constraints in Oracle can either be specified inline with the column definition or out of line, after the column definitions.
- Sequence objects: Unlike SQL Server, Oracle does not support IDENTITY columns. Instead, you can use sequence objects in conjunction with triggers to generate unique values for each row in the table.
Example of Oracle's CREATE TABLE procedure:
CREATE TABLE Employee
(
EmployeeID NUMBER PRIMARY KEY,
FirstName VARCHAR2(50) NOT NULL,
LastName VARCHAR2(50) NOT NULL,
Age NUMBER CHECK(Age>=18),
Email VARCHAR2(100) UNIQUE
)
In summary, while both SQL Server and Oracle have a similar approach to creating tables with the CREATE TABLE statement, there are key differences in terms of data types, constraints, and unique value generation. Becoming familiar with these differences enables you to work across both environments with a deeper understanding of the respective table creation procedures.
Best Practices for SQL Table Design and Creation
Designing and creating efficient SQL tables is crucial for ensuring the database's performance, sustainability, and scalability. By adhering to best practices, you can develop table structures that facilitate effective data storage, retrieval, and management.
How to Create Sustainable and Scalable Table Structures
Creating sustainable and scalable table structures is essential for ensuring that your database can accommodate growing data volumes and support efficient querying. Here are some best practices to consider when designing table structures:
- Choose appropriate data types: Selecting the right data types for each column is fundamental to efficient data storage and query performance. Make sure to choose the data types that best suit the nature and range of the data you are storing.
- Implement primary keys: Using primary keys ensures that each row in a table has a unique identifier, which is crucial for efficient indexing and data retrieval. Whenever possible, opt for simple, immutable keys that aren't prone to changes in the data.
- Establish relationships through foreign keys: Foreign keys are vital for creating relationships between tables and ensuring data consistency. Make sure to establish foreign key constraints between related tables to maintain referential integrity.
- Apply normalization rules: Normalization is the process of organizing database tables to eliminate redundancy and dependency issues. By applying normalization rules, you can create sustainable table structures that prevent data inconsistencies and promote efficient data storage.
- Consider indexing: Indexes can significantly improve the performance of data retrieval operations. Investigate the columns that are frequently used in WHERE clauses and joins, and create indexes for them to optimize query performance.
- Partition large tables: Partitioning tables can help to improve performance in large databases by breaking them into smaller, more manageable pieces. This can facilitate faster querying and maintenance on specific portions of the data.
Optimising Performance in SQL Table Design
Performance is a critical factor to consider when designing SQL tables. Implementing the necessary improvements and adjustments can significantly enhance your database's efficiency and responsiveness. The following suggestions can help optimise performance in your SQL table design:
- Optimise primary key and index structures: Choose primary key and index types that are simple, compact, and efficient. This can include using fixed-size data types like integers, which can improve performance compared to varying-size data types like strings.
- Minimise NULL values: NULL values can contribute to increased storage requirements and poorer query performance. Consider default values or constraints that can prevent NULL values from being stored wherever possible.
- Be mindful of column widths: Specifying unnecessarily large column widths can result in wasted storage space and inefficient data retrieval. It is important to balance the need for accurate and precise data storage with the capacity and performance implications of oversized column widths.
- Consider clustered indexes: Clustered indexes can greatly improve query performance by physically sorting the data within the table based on the index. However, a table can have only one clustered index, so carefully evaluate its impact on potential queries when opting for this design choice.
- Implement data compression: Data compression can help reduce storage requirements for tables, leading to performance benefits. Take advantage of page or row-level compression when suitable to optimise space usage and query performance.
- Employ materialised views or summarised data structures: In scenarios where frequent, complex, and resource-intensive calculations or aggregations are required, consider using materialised views or other summarised data structures to precompute and store the results, enabling faster query performance.
By adopting these best practices and optimisation strategies, you can create SQL table structures that are efficient, scalable, and performant, ensuring that your database is well-suited to accommodate growth and future demands.
Create Table SQL - Key takeaways
Create Table SQL: Fundamental skill for computer scientists, involves establishing table structures using SQL data types.
SQL Data Types: Define the type and format of data stored in table columns, essential for creating and altering tables.
Create Table SQL Server Management Studio: Graphical interface for managing SQL Server instances and creating tables, with step-by-step guides available.
Identity Columns: Used to automatically generate unique identification numbers for table rows, useful for tracking individual records and creating relationships between tables.
SQL Server vs Oracle: Differences in table creation syntax and data types, important to understand when working in different database management systems.
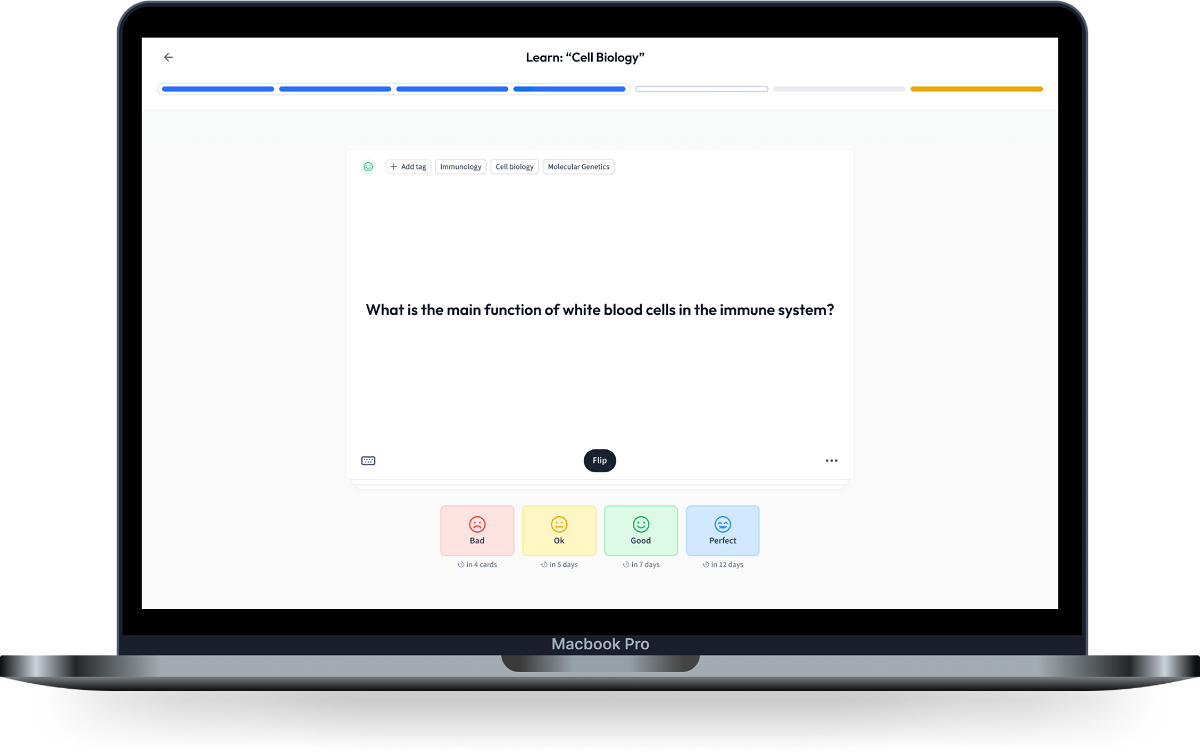
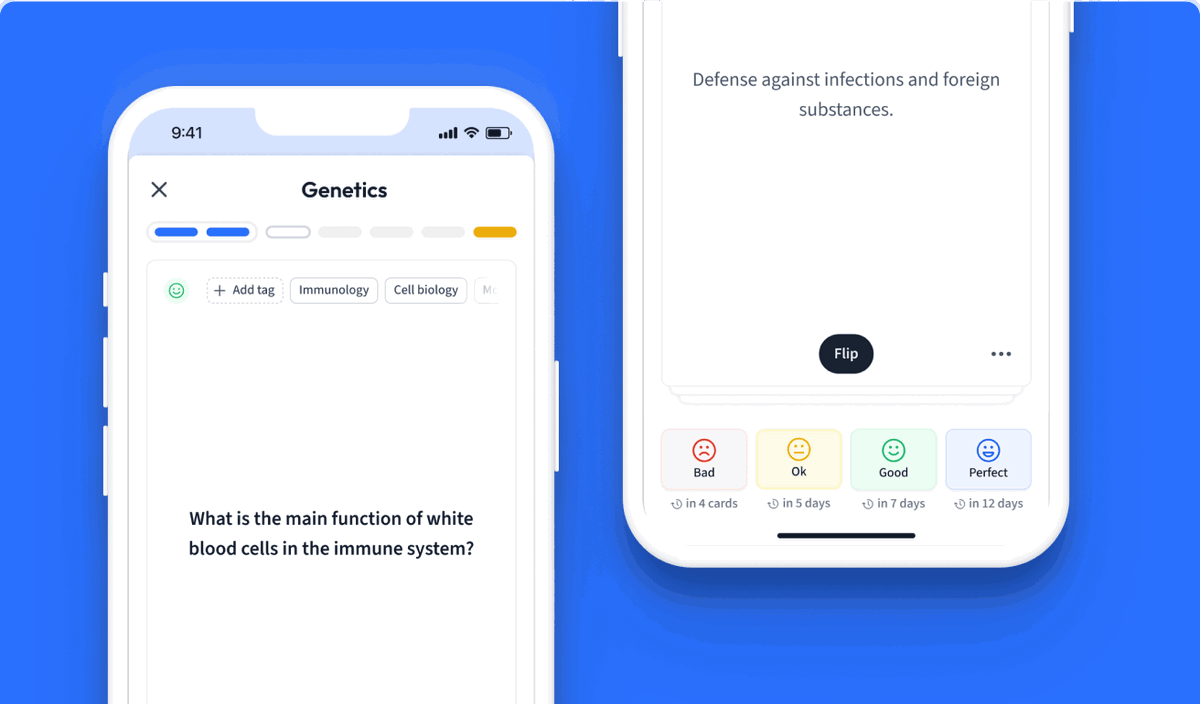
Learn with 15 Create Table SQL flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Create Table SQL
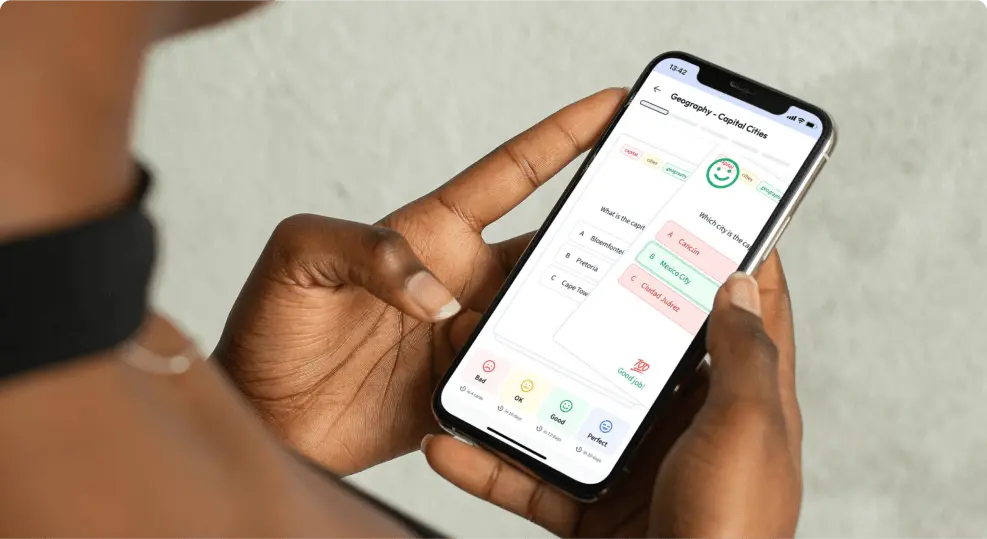
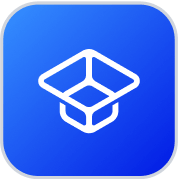
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more