Understanding Predates in SQL Statements
When working with SQL (Structured Query Language), it is crucial to understand the concept of predicates and how they can be applied to filter and refine your database queries.
Definition and Role of Predicates in SQL
A predicate, generally speaking, is a statement that results in a Boolean value, i.e., true or false. In the context of SQL, predicates are the conditions used in SQL statements to filter data based on certain criteria. Predicates are an essential part of SQL statements such as SELECT, INSERT, UPDATE, and DELETE, which allow you to manipulate data in databases. They are mostly used in the WHERE clause of an SQL query, although you may also come across them in HAVING and JOIN clauses.
For example, the following SQL query retrieves all records from the `employees` table where the `salary` is greater than 50000:
SELECT * FROM employees WHERE salary > 50000;
In this case, the predicate is `salary > 50000`, which filters the results based on the salary criteria.
Different Types of Predicates in SQL
SQL includes various types of predicates, which can be divided into three main categories: comparison, logical, and set membership predicates. We will discuss each category in detail.
Comparison Predicates
Comparison predicates are used to compare values, typically involving columns of a table. They return a true or false outcome. The most common comparison predicates in SQL include:
- Equal to (=)
- Not equal to (<> or !=)
- Greater than (>)
- Less than (
- Greater than or equal to (>=)
- Less than or equal to (<=)
Examples of comparison predicates:
-- Select all records where age is greater than 21 SELECT * FROM people WHERE age > 21; -- Select all records where the start date is not equal to '2022-01-01' SELECT * FROM events WHERE start_date <> '2022-01-01';
Logical Predicates
Logical predicates are used to combine multiple conditions using logical operators such as AND, OR, and NOT. They are frequently employed in the WHERE clause of an SQL statement for filtering data based on multiple criteria. The results will be either true or false.
Examples of logical predicates:
-- Select all records where salary is greater than 50000 and the location is 'London' SELECT * FROM employees WHERE salary > 50000 AND location = 'London'; -- Select all records where the department is not 'IT' SELECT * FROM employees WHERE NOT department = 'IT';
Set Membership Predicates
Set membership predicates are used to evaluate whether a specified value belongs to a particular set or range of values. The primary set membership predicates in SQL are IN, BETWEEN, and LIKE.
- IN: Checks if a value is present in a specified list or result set.
- BETWEEN: Determines if the value is within a specified range.
- LIKE: Searches for a specified pattern in a column.
Examples of set membership predicates:
-- Select all records where department is in the specified list SELECT * FROM employees WHERE department IN ('Sales', 'Marketing', 'HR'); -- Select all records where the salary is between 40000 and 60000 SELECT * FROM employees WHERE salary BETWEEN 40000 AND 60000; -- Select all records where job_title starts with 'Manager' SELECT * FROM employees WHERE job_title LIKE 'Manager%';
Understanding predicates in SQL is essential for filtering and refining your database queries efficiently. By using different types of predicates – comparison, logical, and set membership – you can construct powerful queries to extract meaningful insights from your data.
Implementing Predates in SQL Queries
To work efficiently with SQL queries, you must be proficient at implementing various types of predicates, such as comparison, logical, and set membership predicates, as well as row predicates. These predicates play a vital role in refining and filtering SQL query results, allowing you to extract the precise data needed for your analysis or business requirements.
Utilising Comparison Predicates in SQL
Comparison predicates are fundamental elements of SQL queries that compare values in your database. They return a Boolean value (true or false), depending on whether the condition is satisfied. SQL supports several comparison operators, which we list below along with useful examples of their application.
Equal to (=) | SELECT * FROM orders WHERE status = 'Completed'; |
Not equal to (<> or !=) | SELECT * FROM products WHERE category <> 'Electronics'; |
Greater than (>) | SELECT * FROM customers WHERE age > 25; |
Less than (<) | SELECT * FROM inventory WHERE stock < 10; |
Greater than or equal to (>=) | SELECT * FROM employees WHERE years_of_experience >= 5; |
Less than or equal to (<=) | SELECT * FROM projects WHERE duration_in_days <= 30; |
When writing SQL queries, you'll likely use comparison predicates frequently to filter and refine your database search results according to specific criteria.
Using Logical Predicates for Advanced Filtering
Logical predicates come in handy when you need to combine or negate multiple conditions in your SQL query. These predicates employ logical operators such as AND, OR, and NOT to form more complex filtering conditions, providing advanced data search capabilities. Below, we provide examples illustrating the use of these three logical operators in SQL queries.
- AND:
SELECT * FROM employees WHERE department = 'Finance' AND job_title = 'Analyst';
- OR:
SELECT * FROM orders WHERE status = 'Pending' OR status = 'Processing';
- NOT:
SELECT * FROM products WHERE NOT (price >= 100 AND category = 'Electronics');
By using logical predicates, you can create more intricate filtering conditions, enabling you to retrieve specific data sets that meet multiple criteria simultaneously.
Working with Set and Row Predicates
In addition to comparison and logical predicates, SQL offers set and row predicates for more advanced data manipulation. These predicates focus on evaluating whether a value belongs to a defined set or range, or if a row satisfies a specific condition. We'll cover key set and row predicates in SQL as follows:
Set Predicates
Set predicates are used to filter data based on whether a value appears in a given set or satisfies certain criteria. Examples include:
- IN:
SELECT * FROM students WHERE course_id IN (101, 102, 103);
- BETWEEN:
SELECT * FROM products WHERE price BETWEEN 50 AND 100;
- LIKE:
SELECT * FROM contacts WHERE email LIKE '%@example.com';
Row Predicates
Row predicates evaluate whether a specific row in a table satisfies a certain condition. A notable example of a row predicate is EXISTS, which determines if a given subquery returns any rows.
- EXISTS:
SELECT * FROM orders AS o WHERE EXISTS ( SELECT * FROM order_items AS oi WHERE oi.order_id = o.order_id AND oi.product_id = 42 );
By mastering the use of set and row predicates, you can augment your SQL query capabilities, allowing you to search for and retrieve data with a higher level of accuracy and control. Understanding these concepts is fundamental to becoming a proficient SQL user.
Examples of Using Predates in SQL
In this section, we will explore various real-life examples of using predicates in SQL queries to filter and manipulate data effectively. We will cover simple predicate usage, as well as advanced techniques for combining predicates to achieve complex data retrieval requirements.
Filtering Data with Simple Predicates
Using simple predicates in SQL queries is essential for basic data filtering and selection. Below, we delve into a series of examples that elucidate the practical use of different types of predicates—comparison, logical, and set membership—in filtering data within a given context:
- Retrieving employees with an 'Active' status:
SELECT * FROM employees WHERE status = 'Active';
The above example demonstrates a simple comparison predicate where the condition equates to 'status = Active'. Only the rows satisfying this criterion will be returned.
- Selecting products with a price less than £50, excluding the 'Toys' category:
SELECT * FROM products WHERE price < 50 AND category <> 'Toys';
Here, both a comparison predicate and a logical predicate are utilised in the query to filter out products with a specific price range and category.
- Obtaining customers from a predefined list of countries:
SELECT * FROM customers WHERE country IN ('United Kingdom', 'France', 'Germany');
In this example, the set membership predicate 'IN' helps screen customers from a list of specified countries, thus refining the search results.
These examples showcase how simple predicates enable users to easily filter and refine data within SQL queries to fulfill common business tasks and data exploration needs.
Combining Predicates for Complex Data Retrieval
To meet more advanced data retrieval requirements, it is often necessary to employ complex combinations of predicates in SQL queries. By skilfully combining comparison, logical, set membership, and row predicates, users can extract specific data points and derive meaningful insights to support decision-making. The following examples demonstrate elaborate predicate combinations that yield complex data retrieval results:
- Fetching orders placed between a given date range, excluding those with a 'Cancelled' status:
SELECT * FROM orders WHERE order_date BETWEEN '2021-01-01' AND '2021-12-31' AND status <> 'Cancelled';
This example utilises a combination of comparison, logical, and set membership predicates to filter orders based on both a date range and order status. The output retrieves only those orders that meet both criteria simultaneously.
- Extracting employees with either a specific job title or a minimum number of years of experience:
SELECT * FROM employees WHERE job_title = 'Senior Developer' OR years_of_experience >= 5;
In this query, both comparison and logical predicates are combined to select employees with a particular job title or who possess a given minimum experience level. This approach enables users to locate data points that satisfy at least one of the specified conditions.
- Retrieving products whose stock falls below a threshold, accompanied by their supplier information:
SELECT p.*, s.* FROM products p JOIN suppliers s ON s.supplier_id = p.supplier_id WHERE p.stock < 10;
The above example demonstrates a complex SQL query that employs a combination of predicates in a JOIN clause. This approach aggregates data from multiple tables and filters the result set based on the stock threshold condition.
These examples underscore that by adeptly combining various types of predicates in SQL queries, users can efficiently retrieve and manipulate data to address complex business requirements and support data-driven decision-making processes.
SQL Query Optimization with Predicates
By harnessing the power of predicates in SQL queries, you can significantly enhance query performance and optimize database management. Proper implementation of predicates can lead to more efficient data retrieval and reduced server workload. In this section, we delve into predicate pushdown for efficient database management and share useful tips for creating optimized SQL queries.
Predicate Pushdown for Efficient Database Management
Predicate pushdown is an optimization technique in SQL-based databases that relocates the evaluation of predicates as close to the data source as possible. This approach ensures that unnecessary data are filtered out at an early stage of the query execution process, resulting in improved performance and reduced resource consumption.
Benefits of predicate pushdown include:
- Reduction in the amount of data read from storage, thus lowering I/O costs.
- Decreased memory and CPU usage, as less data is transmitted and processed.
- Improved query performance, especially for large-scale data analysis.
Predicate pushdown is typically employed in large-scale distributed storage systems, such as Hadoop, Apache Hive, and Impala, as well as columnar databases like Apache Parquet. However, it can also be integrated into traditional relational databases by using database-specific optimizations, such as indexes and partitioning.
To utilize predicate pushdown in an SQL query effectively, consider the following guidelines:
- Use the correct data types and ensure that data types match between columns and predicates, as this ensures faster evaluations.
- Avoid unnecessary predicate evaluations by extracting minimally required data: that is, use SELECT clauses specifically rather than SELECT * for selecting all columns.
- Utilise indexes and partitioning on frequently referenced or filtered columns to enable the database to efficiently locate and retrieve data based on predicates.
- Incorporate View or User-Defined Functions (UDFs) with filtering conditions for reusable predicates, thereby streamlining complex SQL queries.
Useful Tips for Creating Optimized SQL Queries
With a better understanding of SQL predicate pushdown, it is essential to apply best practices for optimizing your SQL queries for enhanced performance and efficiency. Below, we outline several useful tips to create optimized SQL queries:
- Select only the necessary columns:
Limit the number of columns retrieved by an SQL query. Instead of using SELECT *, specify the required columns explicitly, which reduces the amount of data retrieved and processed by the query.
- Filter data early using predicates:
Apply filtering conditions as early as possible within your SQL query, preferably in the WHERE or JOIN clauses, in order to minimize the amount of data that must be processed, transmitted, and stored.
- Take advantage of indexes:
Create indexes on frequently filtered or sorted columns, enabling the database to quickly locate and fetch the required data with minimal overhead. Additionally, keep these indexes updated to maintain optimal query performance.
- Use JOINs efficiently:
When joining multiple tables, start with the most restrictive predicates in the WHERE clause or the JOIN condition. This practice reduces the amount of intermediate data that needs to be processed during query execution.
- Leverage query optimization features:
Utilise database-specific query optimization features, such as indexed views, partitioning, or materialized views. These techniques can significantly boost the performance of complex SQL queries.
- Analyze query execution plans:
Examine the SQL query execution plans provided by the database management system to identify bottlenecks and inefficient operations. Use this information to adjust and optimize your queries accordingly.
- Test and monitor query performance:
Regularly test and monitor the performance of your SQL queries, using performance monitoring tools or built-in database profiling capabilities. Use the gathered performance metrics to identify areas for optimization and improvement.
By employing these best practices alongside an understanding of predicate pushdown, you can create highly optimized and efficient SQL queries, leading to improved database performance and more effective data-driven decision-making.
Best Practices for Using Predicates in SQL
Using predicates effectively in SQL queries is crucial to improving the performance and efficiency of your database operations. By following best practices, you can not only avoid common pitfalls associated with predicates but also enhance your SQL query performance. In this section, we will discuss strategies for avoiding common pitfalls and optimizing SQL query performance through effective predicate usage.
Avoiding Common Pitfalls with Predicates
When using predicates in SQL, it is essential to be aware of typical pitfalls that may result in poor query performance or incorrect results. Here, we discuss some common pitfalls and suggest solutions to overcome them.
- Implicit data-type conversions: Using different data types in predicates can lead to implicit conversions, which affect both query execution time and accuracy of results. To avoid this, ensure that the data types used in predicates match the underlying column data types.
- Using non-sargable predicates: Non-sargable predicates are predicates that do not take advantage of indexes, usually by employing functions or arithmetic operations on columns. Instead of using these predicates, try to re-write the query in a sargable form that can benefit from index usage, e.g., by moving any functions applied to columns to the right-hand side of the predicate.
- Overusing subqueries: Excessive use of subqueries can slow down query performance. Whenever possible, replace subqueries with joins or derived tables to improve query performance.
- Incorrect order of JOIN operations: The order of JOIN operations matters, especially when using multiple JOINs. When joining tables, begin with the most restrictive predicate to reduce the amount of intermediate data that needs to be processed.
- Not using index-efficient operators: Some operators, such as <> (not equal to), are less efficient when working with indexes. Try to use more index-efficient operators like IN, BETWEEN, or LIKE, and consider re-writing the query to benefit from index usage.
By avoiding these common pitfalls, you can significantly enhance the efficiency and accuracy of your SQL queries when using predicates.
Enhancing SQL Query Performance through Effective Predicate Usage
Effectively using predicates in your SQL queries can lead to substantial improvements in query performance and resource consumption. Here, we explore several tips and best practices for optimizing SQL query performance with predicates:
- Utilise appropriate data types: Make sure the data types in predicates and the associated columns are consistent to avoid implicit type conversions and improve query efficiency.
- Optimise predicate placement: Place predicates as early as possible in the query structure, e.g., in the WHERE or JOIN clause, to filter unnecessary data and reduce the amount of processing required.
- Leverage logical predicates efficiently: Use logical predicates (AND, OR, NOT) effectively to combine or exclude multiple criteria and retrieve more specific results.
- Take advantage of set membership predicates: Use set membership predicates like IN, BETWEEN, and LIKE to filter data based on specific sets or patterns of values.
- Benefit from row predicates: Use row predicates such as EXISTS when querying multiple tables or when filtering data based on a condition that involves multiple columns.
- Apply predicate pushdown: Utilise predicate pushdown techniques to improve query performance by filtering data as early as possible in the query execution process.
- Create and use indexes: Define indexes on columns frequently involved in predicates and ensure that your predicates are index-efficient to maximize the benefits of index usage.
- Monitor and analyze query performance: Keep track of your query performance by using database profiling tools or built-in performance monitoring capabilities. Utilize these insights to optimize the use of predicates in your SQL queries.
By adopting these best practices for using predicates in SQL, you can significantly optimize SQL query performance and resource consumption, ultimately leading to a more efficient and effective data retrieval process.
Using Predicates in SQL Statements - Key takeaways
Definition and role of predicates in SQL: conditions used in SQL statements to filter data based on certain criteria, such as in SELECT, INSERT, UPDATE, and DELETE statements
Three main categories of predicates: comparison (e.g., >,
Predicate pushdown: optimization technique that moves predicate evaluation closer to the data source, reducing resource consumption and increasing performance
Best practices for using predicates: use appropriate data types, optimize predicate placement, use logical predicates efficiently, leverage set membership predicates, and apply predicate pushdown
Important tips for optimized SQL queries: select only necessary columns, filter data early, use JOINs efficiently, take advantage of indexes, and analyze query execution plans
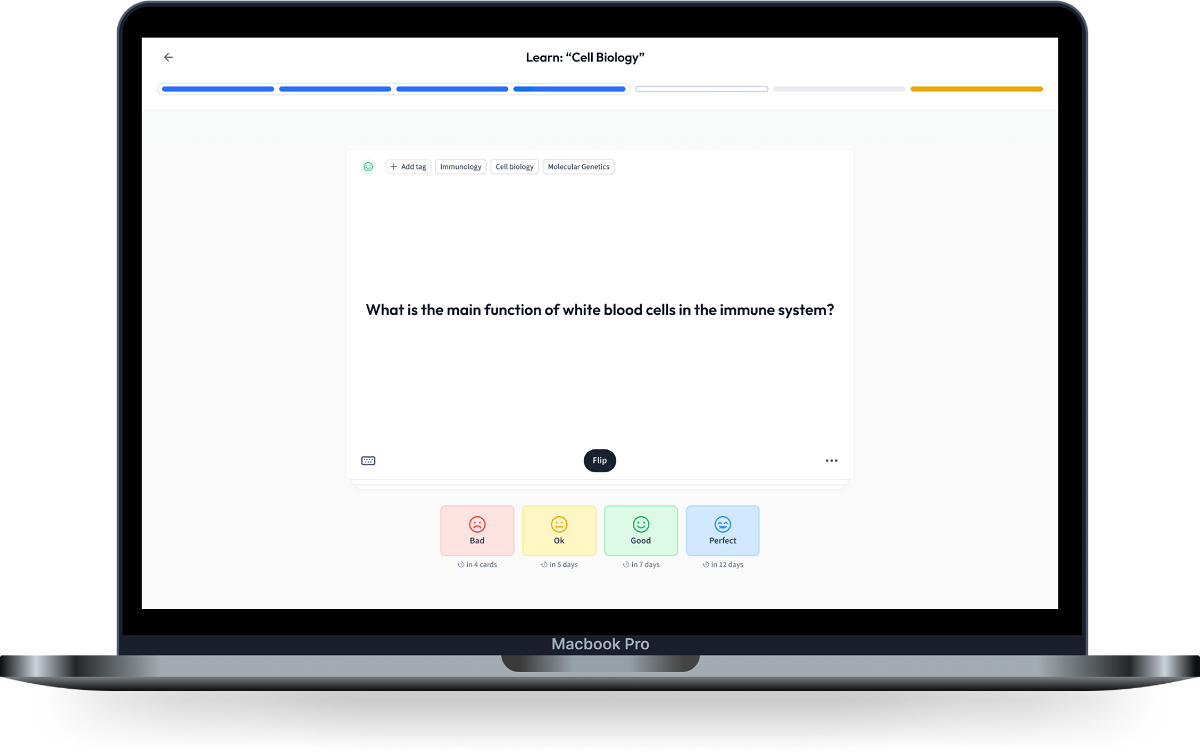
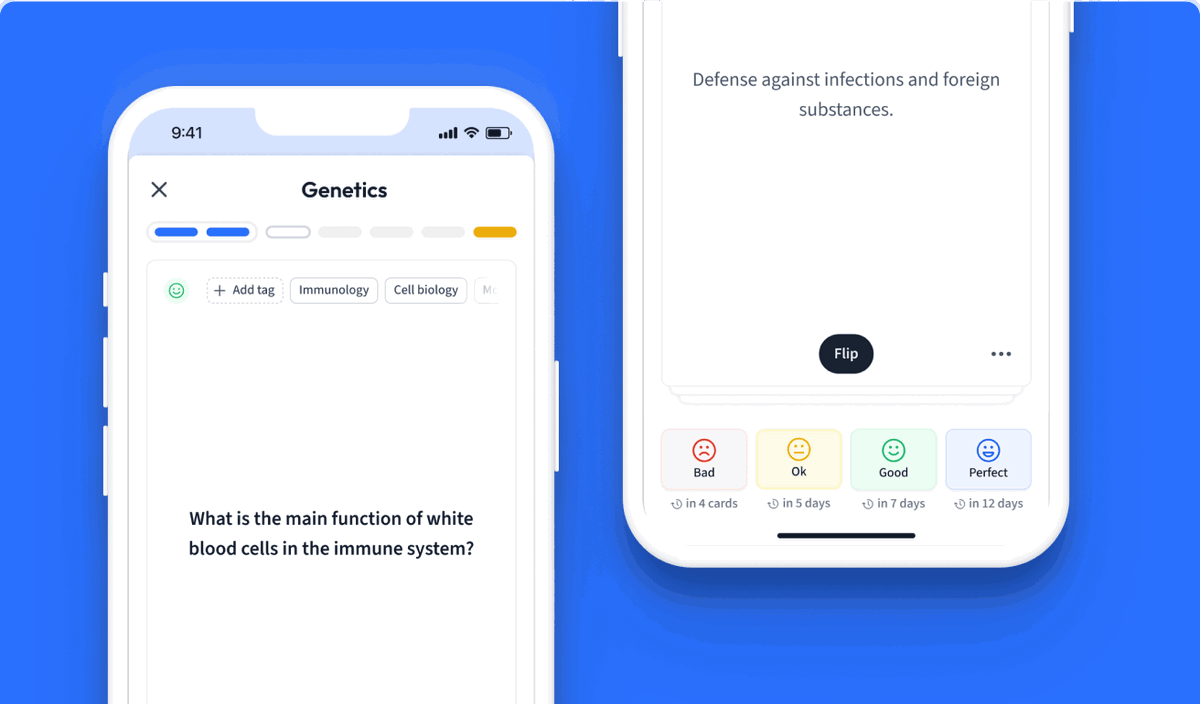
Learn with 11 Using Predicates in SQL Statements flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Using Predicates in SQL Statements
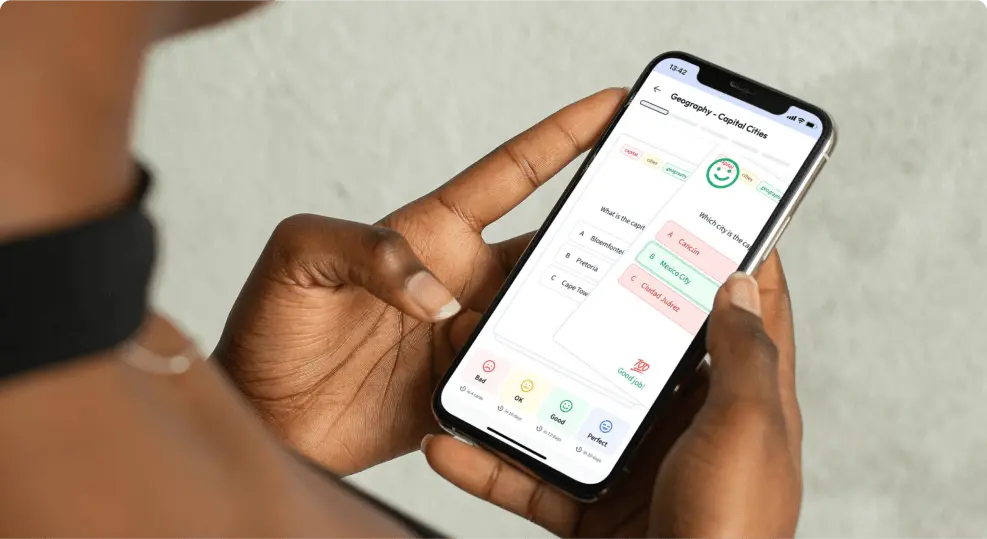
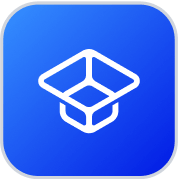
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more