Understanding SQL Cursor
SQL cursors are important concepts in database management, allowing you to manage and manipulate data within result sets by iterating through each record, row by row. Learn the basics of SQL cursors and gain an understanding of the different types and their use cases.
SQL cursor explained: A comprehensive guide
An SQL cursor is a database object that facilitates processing of rows from a result set. When executing a query, you often get a set of rows as a result. In certain situations, you may want to process these rows one-by-one, applying some code or logic to each row. This is where using an SQL cursor comes in handy. Essentially, a cursor can be viewed as a pointer that enables you to traverse the records within a result set, fetch each row individually, and perform necessary operations on each row.
Here are the general steps involved in using a SQL cursor:
- Declare the cursor: In this step, you define the cursor name and specify the query that determines the result set.
- Open the cursor: Opening the cursor executes the query and retrieves the result set.
- Fetch the row(s) from the cursor: This step involves fetching the rows from the result set, one by one, so that you can apply your code or logic on each row.
- Close the cursor: After processing all the rows, you need to close the cursor to release the allocated resources.
- Deallocate the cursor: This step removes the cursor from the memory. Omitting this step can lead to memory leaks.
Cursor Attributes: Cursors have certain attributes that help you to determine the status and position of the cursor. Some commonly used cursor attributes are %FOUND, %NOTFOUND, %ROWCOUNT, and %ISOPEN.
Types of SQL Cursor: Static, Dynamic, and Keyset-driven
There are three main types of SQL cursors: Static, Dynamic, and Keyset-driven. Each type has its own characteristics and use cases. Let's explore these types in detail.
- Static Cursor: A static cursor captures a snapshot of the data at the time the cursor is opened. This means that any changes made in the database after opening the cursor are not visible to the cursor. Static cursors are generally used when you want to work with a stable result set that does not change during the cursor operations.
- Dynamic Cursor: A dynamic cursor reflects the changes made to the data while the cursor is still open. This cursor type is recommended when you need to view and process real-time changes made to the database during the execution of cursor operations.
- Keyset-driven Cursor: A keyset-driven cursor is a mix of static and dynamic cursors. It is based on a set of keys that uniquely identify each row in the result set. When data changes after opening the cursor, it updates the keyset accordingly. However, it does not display new records added to the database or removed records like dynamic cursors.
Comparing SQL cursor types for better database management
Understanding the differences between the types of SQL cursors can help you make better decisions when choosing the most suitable cursor type for your database operations. The table below summarises the key differences between static, dynamic, and keyset-driven cursors.
Cursor Type | Key Characteristics | Use Cases |
Static | A snapshot of data at cursor opening. Changes made after the cursor is opened are not visible. | Working with a stable result set without real-time changes during cursor operations. |
Dynamic | Reflects real-time changes made to the data while the cursor is open. | Viewing and processing real-time changes to the database during cursor operations. |
Keyset-driven | Based on a set of keys that uniquely identify each row. Updates the keyset when data changes but does not display new or removed records. | When working with a result set that may have data updates but does not require accessing newly added or removed records. |
Note that while cursors are powerful tools for processing data row-by-row, they can have an impact on performance, especially when working with large result sets. Always evaluate if using a cursor is the best option for your database operations and consider alternatives such as set-based operations if performance is a concern.
SQL Cursor Practical Usage
In practice, SQL cursors are powerful tools for manipulating data within a database. They allow you to perform row-by-row processing of the data in a result set, making it possible to apply complex logic and computations to each individual record. However, it is important to use cursors efficiently to avoid performance issues, particularly when working with large data sets.
SQL cursor example: A step-by-step tutorial
To understand the practical implementation of SQL cursors, let's walk through a step-by-step example. In this tutorial, we will use a cursor to calculate and update the salary of employees in the 'employees' table based on their performance rating.
Here's the structure of the 'employees' table:
id | name | salary | performance_rating |
Follow these steps:
- Declare a cursor to fetch employee data. Ensure that you select only the necessary columns to improve the cursor's efficiency.
DECLARE emp_cursor CURSOR FOR SELECT id, salary, performance_rating FROM employees;
- Declare variables to hold the fetched data and the updated salary.
DECLARE @id INT, @salary FLOAT, @performance_rating INT, @new_salary FLOAT;
- Open the cursor to execute the query and retrieve the result set.
OPEN emp_cursor;
- Fetch the first row from the cursor and store the data in the declared variables.
FETCH NEXT FROM emp_cursor INTO @id, @salary, @performance_rating;
- Create a loop to iterate through each row in the result set.
WHILE @@FETCH_STATUS = 0 BEGIN
- Calculate the new salary based on the employee's performance rating. Assume that employees with a rating of 1 receive a 10% increase, while those with a rating of 2 receive a 5% increase.
IF @performance_rating = 1 SET @new_salary = @salary * 1.10; ELSE IF @performance_rating = 2 SET @new_salary = @salary * 1.05; ELSE SET @new_salary = @salary;
- Update the employee's salary in the 'employees' table.
UPDATE employees SET salary = @new_salary WHERE id = @id;
- Fetch the next row from the cursor and continue the loop.
FETCH NEXT FROM emp_cursor INTO @id, @salary, @performance_rating; END;
- Close the cursor and release the allocated resources.
CLOSE emp_cursor;
- Deallocate the cursor to remove it from the memory.
DEALLOCATE emp_cursor;
Efficient use of SQL cursor in data manipulation
Although SQL cursors can be convenient for row-by-row data manipulation, they can negatively impact performance, especially when working with large data sets. To use SQL cursors efficiently, consider the following best practices:
- Choose the appropriate cursor type based on your requirements. Selecting the wrong cursor type can lead to unnecessary overhead and performance issues.
- Limit the number of columns in the SELECT query to only those necessary for the cursor operation. Fetching unnecessary columns can consume additional resources and slow down the operation.
- Avoid nesting cursors, as this can lead to exponentially increased complexity and resource consumption. Consider using alternative approaches such as temporary tables or set-based operations instead.
- Close and deallocate cursors properly to release all allocated resources and prevent memory leaks.
- In some cases, consider using set-based operations instead of cursors for improved performance. While set-based operations may not be suitable for all scenarios, they can often provide faster results when used appropriately.
By following these best practices and emphasizing efficient use of SQL cursors, you can enhance your database management skills and improve the performance of data manipulation operations.
Analysing SQL Cursor Alternatives and Comparisons
It is essential to explore and compare SQL cursor alternatives when working with databases, as different situations may call for different solutions. Understanding how SQL cursors compare to other techniques such as while loops and exploring alternative approaches can help you make better decisions when it comes to database management and optimisation.
SQL cursor vs while loop: Which one to use?
Both SQL cursors and while loops can be used for row-by-row processing of data in a result set. However, they have differences in performance, flexibility, and application. Selecting the right method for your requirements involves weighing their strengths and weaknesses.
Evaluating differences in performance and application
SQL cursors and while loops each have their own advantages and limitations, which can impact their performance and suitability for different tasks. Let's compare these two methods in terms of their performance and application:
- Performance:
- SQL cursors are often less efficient than while loops because they require additional database resources for maintaining the pointer and fetching rows one by one. This can lead to performance issues, especially when working with large result sets.
- While loops typically offer better performance than SQL cursors, as they involve fewer database interactions and are less resource-intensive.
- Flexibility:
- SQL cursors provide greater flexibility, allowing you to fetch rows from multiple tables and perform complex tasks such as row-by-row calculations and updates. In addition, you can choose from various cursor types (static, dynamic, and keyset-driven) based on your requirements.
- While loops are relatively less flexible, as they are usually limited to row-by-row operations within a single table, and the looping conditions can sometimes be more complex to implement.
- Application:
- SQL cursors are best suited for situations that involve row-by-row processing, complex calculations, or row-by-row updates of records fetched from one or more tables.
- While loops are more appropriate for simpler tasks or when you need a less resource-intensive solution for row-by-row processing within a single table. They may also be a better choice for tasks that can be accomplished using set-based operations.
Considering these differences, it is important to evaluate the specific requirements of your task, including performance, flexibility, and application, before choosing between an SQL cursor and a while loop.
SQL cursor alternative: Exploring other options in database management
In some cases, SQL cursors may not be the most efficient method for row-by-row processing or other database operations. Therefore, it is valuable to learn about alternative approaches that can be used in different situations and offer potential performance benefits over SQL cursors.
Benefits of using SQL cursor alternatives
SQL cursor alternatives can provide several benefits, making them worth exploring for certain data manipulation tasks. Some of these benefits include:
- Improved performance: Alternatives to SQL cursors often involve fewer resources and less overhead, potentially providing better performance, especially when working with large data sets.
- Reduced complexity: SQL cursor alternatives may involve simpler code and logic, making it easier to write, read, and maintain.
- Versatility: Knowing a variety of SQL cursor alternatives can help you choose the most appropriate method for your specific task, ultimately leading to more efficient and effective database management.
Some common SQL cursor alternatives include:
- Set-based operations: Set-based operations, such as SELECT, UPDATE, INSERT, and DELETE, can be used to perform bulk operations on multiple rows simultaneously, leading to faster execution times when compared to row-by-row processing using cursors.
- Common Table Expressions (CTEs): CTEs are temporary, named result sets that can be used to simplify complex queries and improve query performance. They can be a useful alternative for some tasks that would otherwise require cursors.
- Window Functions: Window functions enable you to perform calculations across a set of rows related to the current row, making it possible to avoid row-by-row processing in certain situations and potentially improve performance.
- Subqueries and Correlated Subqueries: Subqueries, including correlated subqueries, can be used to retrieve data from multiple tables or perform calculations based on related data, offering another alternative to using cursors for some applications.
When exploring SQL cursor alternatives, it is crucial to understand their specific use cases and weigh their benefits and limitations based on your individual requirements. By doing so, you can better optimise your database operations and ensure efficient data management.
SQL Cursor - Key takeaways
SQL Cursor: A database object used for row-by-row processing of data in a result set, allowing complex logic and computations to be applied to each individual record.
Cursor Types: Static (captures a snapshot of data), Dynamic (reflects real-time changes), and Keyset-driven (updates keyset when data changes but does not display new or removed records).
SQL Cursor vs While Loop: Cursors provide greater flexibility but may have performance issues compared to While Loops, especially with large data sets.
SQL Cursor Alternatives: Set-based operations, Common Table Expressions (CTEs), Window Functions, and Subqueries and Correlated Subqueries.
Efficient use of SQL Cursors: Choose the correct cursor type, limit the number of columns in SELECT queries, avoid nesting cursors, close and deallocate cursors, and consider set-based operations when possible.
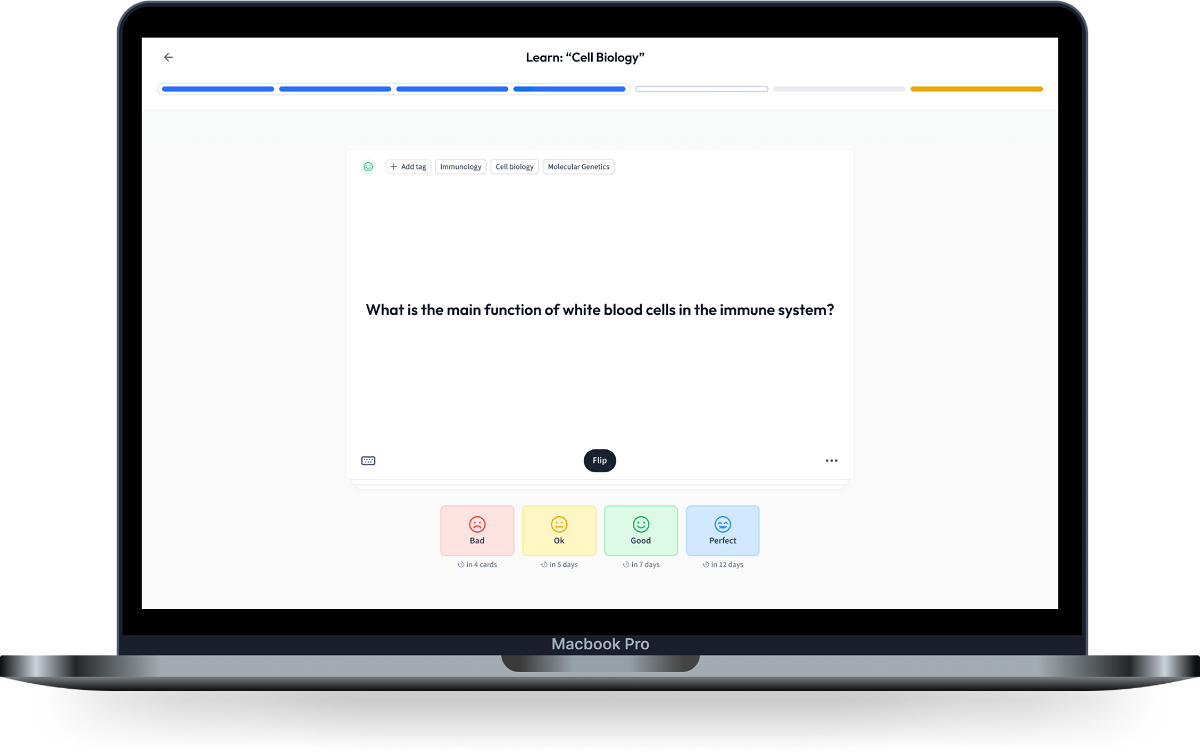
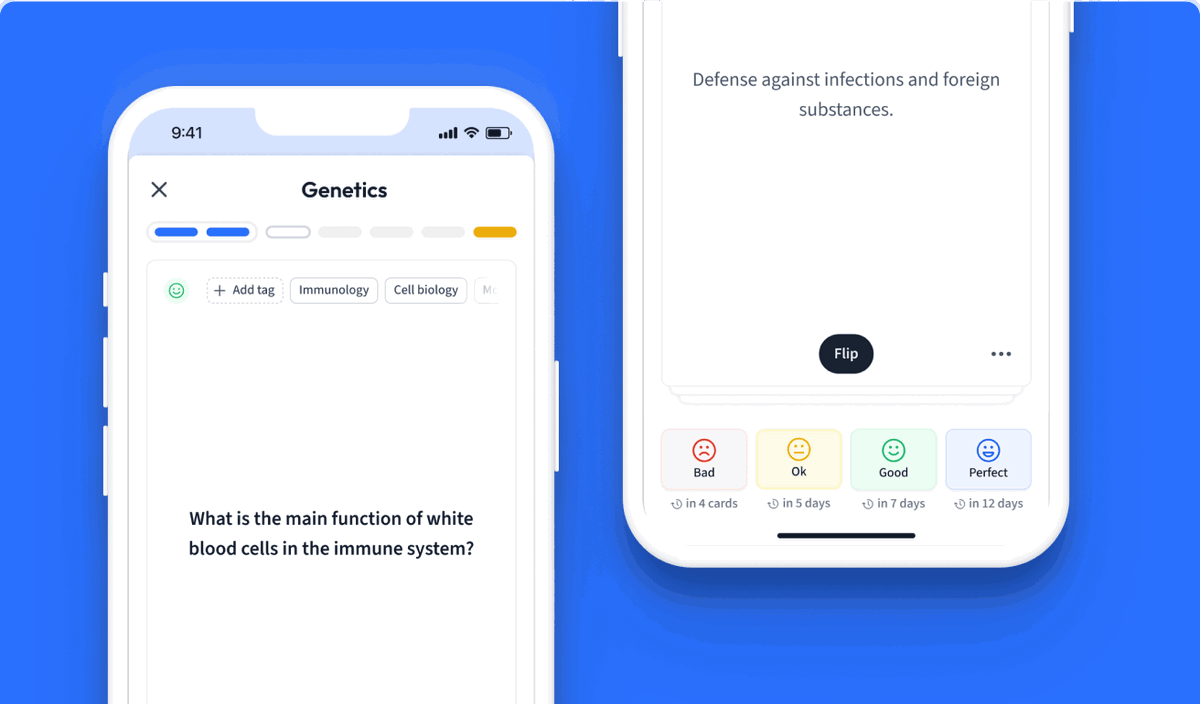
Learn with 34 SQL Cursor flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about SQL Cursor
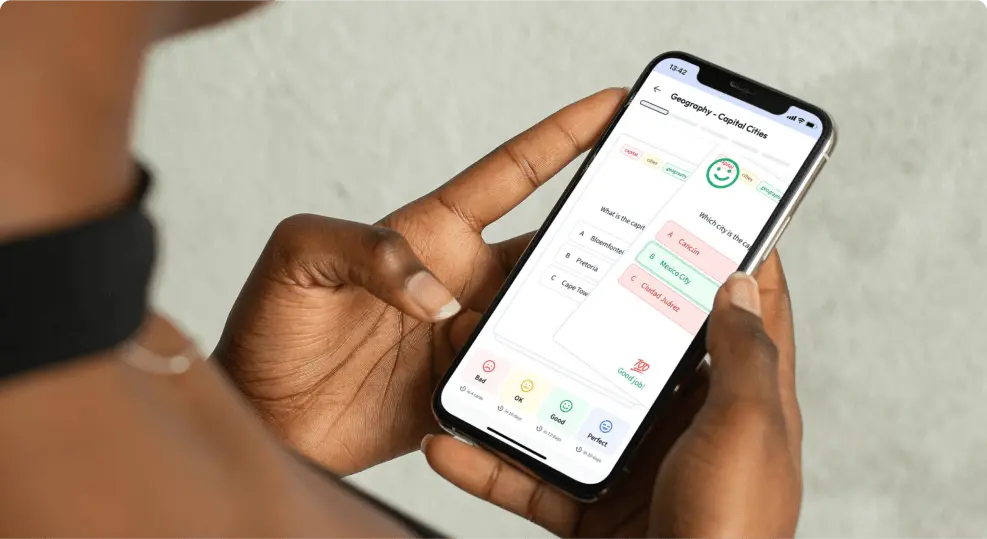
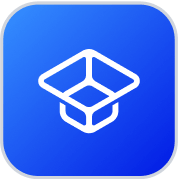
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more