SQL Trigger Update Explained
A SQL Trigger Update is a procedural code that automatically executes in response to specific events, like INSERT, UPDATE, DELETE, or TRUNCATE in a specified table or view within the database.
For example, a trigger could be set up to automatically update the 'last_modified' date in a customer's record whenever specific fields are updated.
Different Types of SQL Triggers
Triggers can be broadly categorized into various types based on different criteria, such as the execution moment and action. Triggers based on execution moment: - BEFORE: Triggered before an INSERT, UPDATE, or DELETE action is carried out on a specified table. - AFTER: Triggered after an INSERT, UPDATE, or DELETE action is done on a specified table. - INSTEAD OF: Run instead of the original action that caused the trigger within a view. Triggers based on action type: - INSERT TRIGGER: Automatically executed when an INSERT command runs on the specified table. - UPDATE TRIGGER: Executed whenever an UPDATE command modifies the specified table. - DELETE TRIGGER:Invoked when a DELETE command removes data from the specified table.Pros and Cons of Implementing SQL Trigger Updates
SQL Trigger Updates come with some advantages and disadvantages. Assessing the pros and cons is crucial when implementing them in your database management system. Pros:- Create and maintain data consistency and integrity across multiple tables.
- Reduce application code, as you can keep specific business logic within the database.
- Enforce constraints and manage specific data access permissions.
- Automate various tasks and streamline data management processes.
- Triggers can be challenging to debug, as the process is largely hidden from the user.
- Performance can suffer due to multiple triggers being executed simultaneously, causing cascading effects as one triggers another.
- Compelx triggers can introduce deadlock situations and lead to application failure.
- Improper implementation of triggers may result in data inconsistencies or failed transactions.
When designing a database system, it is essential to consider both the benefits and potential pitfalls of SQL Trigger Updates. Properly implemented triggers can significantly improve data integrity and management processes, while improperly executed triggers may introduce more problems than they solve.
SQL Trigger Update Example and Use Cases
Creating Your First SQL Trigger Update
To create an SQL Trigger Update, start by identifying the table and event that should trigger the desired action.
For this example, we will create a simple trigger to update the 'last_modified' date in a customers table whenever specific fields are changed. The fields to monitor for updates are 'first_name', 'last_name', and 'email'. Here is a step-by-step guide for creating an SQL Trigger Update:
1. Use the CREATE TRIGGER statement and specify a unique name for the trigger. It's good practice to use a descriptive name for easy identification:
CREATE TRIGGER update_customer_timestamp
2. Specify the event that will trigger the action (AFTER, BEFORE, or INSTEAD OF). In this example, we use AFTER to update the 'last_modified' field after the target fields are updated:
AFTER UPDATE
3. Define the table and columns to monitor for updates. We're monitoring the 'first_name', 'last_name', and 'email' columns in the 'customers' table:
ON customers FOR EACH ROW WHEN (OLD.first_name <> NEW.first_name OR OLD.last_name <> NEW.last_name OR OLD.email <> NEW.email)
4. In the trigger body, provide the action that should take place. In this case, we update the 'last_modified' field with the current date and time:
BEGIN
UPDATE customers SET last_modified = NOW() WHERE customer_id = NEW.customer_id;
END;
5. Combine the code snippets and execute the CREATE TRIGGER statement in your database:
CREATE TRIGGER update_customer_timestamp
AFTER UPDATE
ON customers FOR EACH ROW WHEN (OLD.first_name <> NEW.first_name OR OLD.last_name <> NEW.last_name OR OLD.email <> NEW.email)
BEGIN
UPDATE customers SET last_modified = NOW() WHERE customer_id = NEW.customer_id;
END;
Once created, this SQL Trigger will automatically update the 'last_modified' field whenever the specified fields are updated.
Examples of Practical SQL Trigger Update Applications
There are numerous practical applications of SQL Trigger Updates to manage data efficiently and enforce business logic. Some common use cases include: Audit Trail: Creating a log of all changes made to specific tables is useful for tracking user activity, ensuring data security, and aiding in troubleshooting errors. Data Validation: SQL Trigger Updates can enforce data validation rules and prevent users from entering invalid or incomplete information into the database. Cascading Updates: A trigger can propagate changes in one table to related tables, ensuring data consistency and integrity throughout the system. Maintaining Referential Integrity: Triggers can automatically update related records when primary keys are updated, preventing orphaned records and maintaining referential integrity. Automating Business Processes:Some business processes require automatic actions in response to specific data changes. SQL Trigger Updates can handle such situations and execute these actions automatically.Common Mistakes and Best Practices in SQL Trigger Updates
When implementing SQL Trigger Updates, keeping in mind best practices and avoiding common mistakes is essential for achieving optimal results:Common Mistakes:
- Overusing triggers, leading to performance issues and challenging debugging scenarios.
- Failing to consider transaction management, resulting in data integrity issues and deadlocks.
- Using triggers to handle tasks that could be more efficiently implemented through stored procedures or application code.
- Missing crucial error handling and logging mechanisms within triggers.
Best Practices:
- Use triggers sparingly and only when necessary for enforcing business logic or maintaining data integrity.
- Optimise triggers for performance, ensuring that they only execute when needed and avoid complex or computationally expensive operations.
- Always implement error handling and logging within triggers to aid in troubleshooting and monitoring.
- Consider transaction management and rollback mechanisms within triggers to maintain data integrity.
- Test triggers extensively to ensure correct behaviour and performance within the overall system.
SQL Trigger Update Inserted Row Scenarios
An inserted row in a database refers to a new record that has been added using an INSERT statement. SQL Trigger Updates can be employed to handle actions associated with inserted rows, such as enforcing data validation rules or propagating the changes to related tables.
To effectively manage inserted rows with SQL Trigger Updates, follow these steps:
- Choose the appropriate event that will trigger the action. In this case, use the AFTER or BEFORE event type to detect when an INSERT operation has been executed.
- Specify the table to monitor for newly inserted rows.
- Create the trigger body that outlines the desired actions in response to the detected INSERT event.
CREATE TRIGGER insert_audit_log AFTER INSERT ON users FOR EACH ROW BEGIN INSERT INTO audit_log (user_id, username, action, timestamp) VALUES (NEW.user_id, NEW.username, 'INSERT', NOW()); END;This trigger automatically records the user_id, username, action type ('INSERT'), and a timestamp in the audit_log table whenever a new row is inserted into the users table.
SQL Trigger Update and the Death of Data Anomalies
SQL Trigger Updates are essential in eliminating data anomalies, ensuring data consistency, integrity, and correctness in a database. When dealing with inserted rows, triggers can help address the following types of data anomalies:- Insert Anomalies: Triggers can enforce required fields and validate data before inserting records, preventing instances where crucial information may be missing.
- Update Anomalies: By automating tasks such as updating related records in response to newly inserted rows, triggers maintain data consistency across multiple tables and prevent discrepancies.
CREATE TRIGGER update_sales_summary AFTER INSERT ON sales FOR EACH ROW BEGIN UPDATE sales_summary SET total_sales = total_sales + NEW.total_amount WHERE sales_summary_id = NEW.sales_summary_id; END;This trigger ensures that the 'sales_summary' table is always up to date and consistent with the 'sales' table, effectively eliminating data anomalies.
Ensuring Data Consistency with SQL Trigger Update Inserted Row
Ensuring data consistency is one of the primary goals of database management systems. A critical aspect of data consistency is maintaining accurate relationships between tables and their associated data. SQL Trigger Updates provide an effective way to manage inserted rows and maintain consistency across related tables. Consider a scenario where you have a database with tables 'products' and 'inventory'. When a new row is inserted into the 'products' table, you need to create a corresponding entry in the 'inventory' table to track available stock. This can be achieved with an AFTER INSERT trigger:CREATE TRIGGER create_inventory_record AFTER INSERT ON products FOR EACH ROW BEGIN INSERT INTO inventory (product_id, stock_available) VALUES (NEW.product_id, 0); END;This trigger ensures that every time a new product is added to the 'products' table, a corresponding entry is created in the 'inventory' table, maintaining data consistency between the two tables. By leveraging SQL Trigger Updates to handle inserted rows, valuable consistency and integrity are maintained throughout the database.
SQL Trigger Update Another Table Techniques
Synchronising multiple tables efficiently is crucial in many database management scenarios. With SQL Trigger Updates, achieving this becomes feasible by automating specific actions as the data changes in the related tables. SQL Trigger techniques for syncing tables involve understanding and establishing relationships between tables and defining triggers that automatically update or insert data based on specific events. Here are some essential steps in synchronising multiple tables with SQL Trigger Updates: 1. Define relationships between tables: Establish the relationships between the affected tables, such as primary key and foreign key relationships. 2. Determine the type of action needed:Identify the actions required for synchronising the tables, such as:- Inserting
- Updating
- Deleting data
For example, imagine a scenario with two tables 'orders' and 'order_history'. Suppose that you want to update the 'order_history' table whenever a new row is inserted or an existing row is updated in the 'orders' table. Following the steps mentioned above, the relationships between the tables are established (order_id being the primary key and foreign key), and triggers are created for both INSERT and UPDATE events:
-- Trigger for INSERT event
CREATE TRIGGER sync_insert_orders
AFTER INSERT
ON orders FOR EACH ROW
BEGIN
INSERT INTO order_history (order_id, status, timestamp)
VALUES (NEW.order_id, NEW.status, NOW());
END;
-- Trigger for UPDATE event
CREATE TRIGGER sync_update_orders
AFTER UPDATE
ON orders FOR EACH ROW
BEGIN
INSERT INTO order_history (order_id, status, timestamp)
VALUES (NEW.order_id, NEW.status, NOW());
END
These triggers ensure that the 'order_history' table is synchronised with the 'orders' table whenever an INSERT or UPDATE event occurs.Mastering the Art of SQL Trigger Update Another Table
To master the art of creating SQL Triggers for updating another table, it is essential to follow best practices and become proficient in various SQL Trigger concepts. The steps below describe how to achieve this:1. Understand SQL Trigger types: Get familiar with different trigger types such as AFTER, BEFORE, and INSTEAD OF, and learn when to use each type in various scenarios. 2. Choose appropriate actions: Identify the ideal actions to be performed by the triggers and understand their implications on the database.3. Write efficient trigger bodies: Ensure that the trigger body is optimised for performance by using appropriate clauses and reducing computational overhead. 4. Consider chain reactions and cascading effects: Be mindful of the potential cascading effects that can arise from triggers, and avoid creating triggers that cause chain reactions or deadlocks. 5. Be aware of transaction management: When designing triggers, consider the implications they may have on transaction management and rollback mechanisms. 6. Test and monitor triggers: Regular testing and monitoring of the created triggers are essential for achieving optimal performance and ensuring proper functioning. 7. Consistently follow best practices:Adhere to best practices in trigger creation, such as using descriptive trigger names and maintaining error handling and logging mechanisms. By following these steps, mastering the art of SQL Trigger Update techniques for updating another table will become more accessible and achievable.Advanced Strategies in SQL Trigger Update Implementation
Advanced strategies in SQL Trigger Update implementation involve understanding and exploiting powerful features of SQL triggers to achieve greater efficiency and effectiveness in managing database changes. Some advanced techniques and strategies include: 1. Conditional Triggers: Implement triggers that only execute when specific conditions are met. This can help optimise performance and reduce unnecessary trigger execution. 2. Dynamic SQL in Triggers: Use dynamic SQL statements within triggers to create more flexible and granular control over triggered actions.3. Nested Triggers: Leverage the power of nested triggers to perform more complex database operations. 4. Maintaining History and Audit Trails: Create triggers to maintain history tables that track changes over time, or audit trails for monitoring and troubleshooting purposes. 5. Triggers for Referential Integrity: Utilise triggers to maintain referential integrity across related tables by propagating changes and preventing orphaned records. 6. Custom Error Handling: Implement custom error handling within triggers to provide better diagnostic information about trigger-related issues or failures. 7. Integrating with Stored Procedures: Combine triggers with stored procedures to encapsulate complex business logic within the database itself. By adopting these advanced strategies, you will be able to utilise SQL Trigger Updates in more sophisticated and powerful ways, allowing better management of your databases and catering to complex business logic and data integrity requirements.SQL Trigger Update After Update Methods
Optimising SQL Trigger Updates can significantly improve the efficiency and performance of a database system. One popular method for enhancing trigger efficiency is focusing on the timing of executing triggers, such as executing them after an UPDATE event. This approach enables the SQL Triggers to only execute when necessary.
Timing SQL Trigger Updates for Better Efficiency
To optimise the efficiency of SQL Trigger updates, it is essential to time them appropriately. The choice between AFTER or BEFORE update trigger methods can impact the performance of the database system. By choosing to execute triggers after an UPDATE event, their execution can be postponed, allowing for improved efficiency. Consider the following methods for improving trigger timing: 1. Determine the ideal trigger type: Choose between AFTER or BEFORE triggers based on whether the trigger action should occur after or before the database records have been updated. The chosen type will affect the transaction duration and locking times in the database. 2. Analyse trigger dependencies: Evaluate the dependencies between different triggers and avoid creating triggers that have circular dependencies or cause a cascading execution.3. Execute triggers conditionally: Use trigger conditions to ensure that triggers only execute when specific conditions are met. This can help reduce unnecessary trigger executions and improve overall performance. 4. Control transaction scope:Be mindful of the transaction scope when working with triggers, as it can directly impact the trigger's execution and the overall performance of the database system.By implementing these techniques, you can boost the efficiency of your SQL Trigger updates and ensure smooth operation in your database management system.
The Role of SQL Trigger Update After Update in Database Management
SQL Trigger Update AFTER UPDATE plays a significant role in maintaining database integrity, enforcing business rules, and automating data management processes. Here are some critical functions of AFTER UPDATE triggers in database management: Data Consistency and Integrity: Triggers help maintain data consistency and integrity by automatically updating dependent data in related tables, ensuring that data remains accurate and up-to-date. Automating Business Processes: AFTER UPDATE triggers can be employed to automate specific tasks or actions in response to data updates, adhering to various business rules and requirements. Error Handling and Logging: Triggers can be used to handle errors and log information about data changes in audit trails, ensuring that errors can be diagnosed, and suspicious activities can be monitored.Improved Data Validation: SQL Triggers can enforce data validation rules and constraints, preventing users from entering invalid or incomplete data. By incorporating SQL Trigger Update AFTER UPDATE in your database management system, these crucial functions will ensure that your data remains consistent, accurate, and aligned with your business objectives.Case Study: SQL Trigger Update After Update in Real-life Applications
In a real-life application, consider an e-commerce platform that contains tables named 'products' and 'product_prices'. Whenever a product receives a price update, the e-commerce platform must also update other related data and actions, such as re-arranging products based on the new price or sending notifications to potential buyers. AFTER UPDATE triggers can automate these tasks seamlessly. For example, a trigger could be created to update a 'product_recommendations' table, which stores a list of products based on their updated prices and sale performance. This trigger could be designed as:CREATE TRIGGER update_product_recommendations AFTER UPDATE ON product_prices FOR EACH ROW WHEN (NEW.price <> OLD.price) BEGIN -- Update the product_recommendations table with new data UPDATE product_recommendations SET price = NEW.price WHERE product_id = NEW.product_ID; END;This trigger ensures that the 'product_recommendations' table is updated accordingly whenever a product's price changes. Through such innovative use of SQL Trigger Update AFTER UPDATE, businesses can achieve better efficiency, improved data accuracy, and seamless handling of critical data updates.
SQL Trigger Update - Key takeaways
SQL Trigger Update: Procedural code that performs actions in response to specific events, like INSERT, UPDATE, DELETE, or TRUNCATE, on a table or view within the database.
Types of SQL Triggers: Based on execution moment (BEFORE, AFTER, INSTEAD OF) and action type (INSERT, UPDATE, DELETE).
Pros and Cons: Triggers can improve data consistency and integrity, enforce constraints, and automate tasks, but they may also be challenging to debug, affect performance, and introduce deadlocks.
Handling Inserted Rows: SQL Trigger Updates can enforce data validation rules, maintain data consistency, and prevent data anomalies when handling inserted rows.
Synchronising Multiple Tables: SQL Triggers can maintain relationships between tables, automatically update or insert data, and ensure data consistency and integrity across the system.
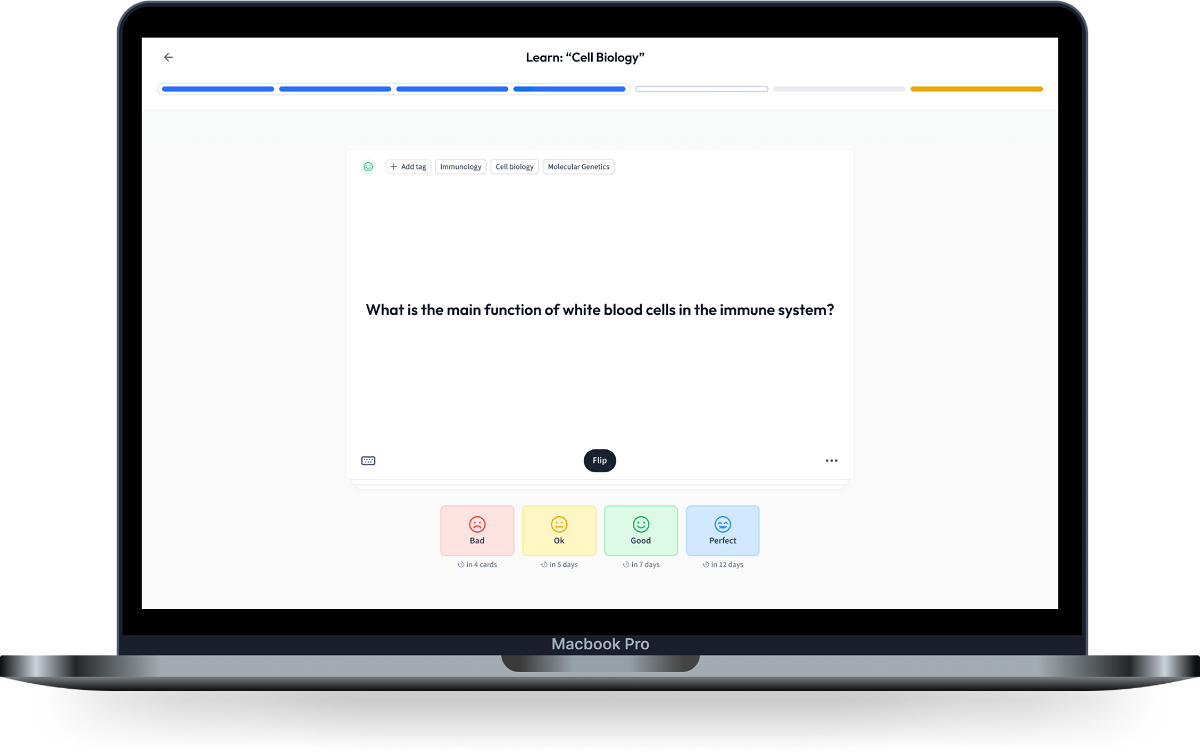
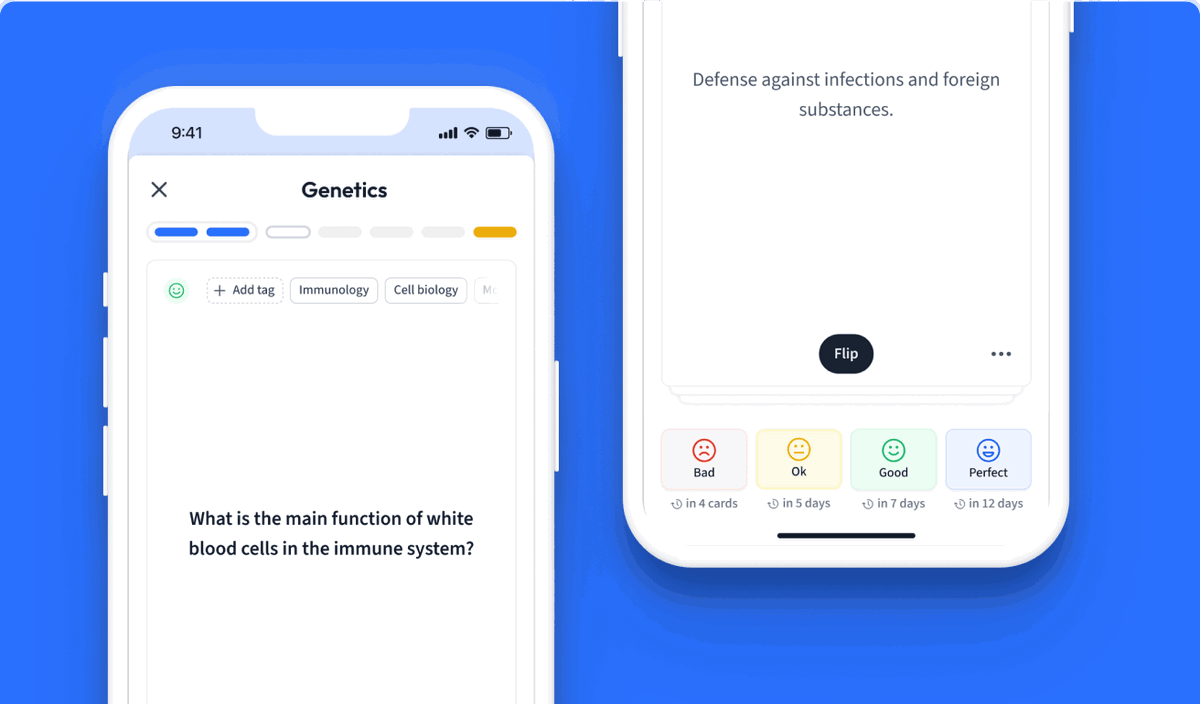
Learn with 15 SQL Trigger Update flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about SQL Trigger Update
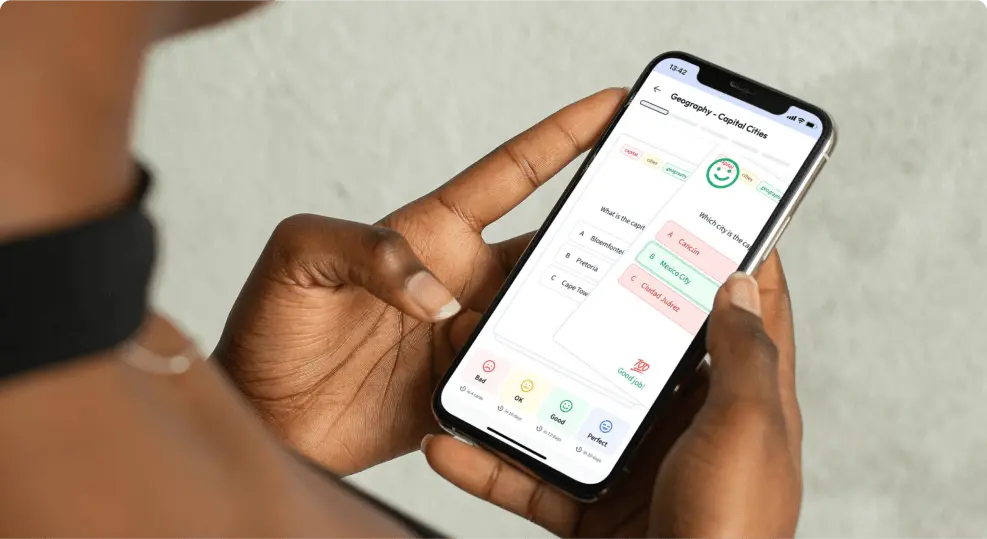
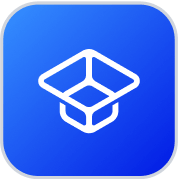
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more