SQL Triggers Explained
A trigger is a named database object that performs a specified action automatically when an event occurs, such as an INSERT, UPDATE, DELETE, or TRUNCATE statement on a specified table or view. SQL triggers enable you to perform additional actions in response to changes in data, helping to maintain the integrity of your database.
Basic Concepts of SQL Triggers
In SQL, triggers are important tools for maintaining data consistency, enforcing business rules, and auditing data changes. Some frequently used concepts in the context of SQL triggers include:- Trigger Event: The event that causes a trigger to be executed, such as INSERT, UPDATE, or DELETE statements.
- Trigger Action: The action performed by the trigger, typically involving modifications to one or more tables.
- Triggering Event: The occurrence that initiates the trigger's execution. This includes data manipulation operations like inserting, updating, or deleting records in a table.
- Triggering Table: The table on which the trigger event is defined, such as the table being modified or accessed.
How SQL Triggers Work
When an event occurs that satisfies the conditions defined in a trigger, the action specified in the trigger is automatically executed. Here's an example explaining the process:Suppose you have an online store with a table named orders. Whenever a new order is INSERTED, you want to decrease the available stock of the product by the given quantity. A trigger can be created on the orders table that gets executed whenever a new order is INSERTED, and this trigger would then automatically update the stock table.
SQL Triggers Types and Use Cases
Triggers can be divided into different types based on the triggering event or the level at which they are executed. Understanding these types of triggers allows you to decide which type is suitable for your specific use case.Before and After Triggers
Before and After triggers are determined by the timing of their execution:- Before Triggers (
BEFORE INSERT
,BEFORE UPDATE
,BEFORE DELETE
): These triggers execute before the triggering event. They can be used to modify the data before it is saved in the database, which is useful for data validation or cleanup. - After Triggers (
AFTER INSERT
,AFTER UPDATE
,AFTER DELETE
): These triggers execute after the triggering event. They can be useful for actions that depend on the changes made by the event, such as maintaining a record of changes or updating related tables.
It's important to note that TRUNCATE cannot have a trigger directly associated with it because it's not logged on a per-row basis. However, it is possible to create a trigger on an auxiliary table and then use a DELETE statement on that table to trigger the desired action.
Row-Level and Statement-Level Triggers
Row-Level and Statement-Level triggers are distinguished by the scope of the triggering event:- Row-Level Triggers (
FOR EACH ROW
): These triggers are executed once for each row affected by the triggering event. They are useful for operations that need to be applied to individual records, such as maintaining a history of changes for each row. - Statement-Level Triggers: These triggers are executed once for each triggering event, regardless of the number of rows affected. They are suited for operations that need to be performed once per event, such as maintaining summary or audit tables.
A practical example of a row-level trigger would be keeping track of the last modification date for each row in a table. As a new row is INSERTED or an existing row is UPDATED, the trigger would update a "last_modified" column for the affected row.
Working with SQL Triggers Examples
To create an SQL trigger, you will first need to understand the trigger creation syntax and then apply it to a practical use case, such as creating a trigger for auditing purposes or for maintaining referential integrity between related tables.Syntax for Creating an SQL Trigger
The syntax for creating an SQL trigger varies depending on the database management system you are using, but the general structure remains the same. Here is an outline of the basic syntax:CREATE TRIGGER trigger_name {BEFORE|AFTER} {INSERT|UPDATE|DELETE} ON table_name [FOR EACH ROW] trigger_body;
CREATE TRIGGER
: This is the command used to create a new trigger.trigger_name
: A unique name for the trigger.BEFORE|AFTER
: The trigger timing, determining when the trigger action is executed with respect to the triggering event.INSERT|UPDATE|DELETE
: The triggering event that activates the trigger.ON table_name
: Specifies the table on which to create the trigger.FOR EACH ROW
: Optional modifier for row-level triggers.trigger_body
: The code that will be executed when the trigger is activated.
Practical Use Case: Creating a Trigger
Let's see an example of creating a trigger to maintain a history of changes in an employee's salary. Suppose you have two tables, one named 'employees' and another named 'employees_salary_history'. Whenever the salary of an employee is updated in the 'employees' table, we want to add a new row in the 'employees_salary_history' table. Here's the SQL code to create the trigger:CREATE TRIGGER trg_salary_history AFTER UPDATE OF salary ON employees FOR EACH ROW INSERT INTO employees_salary_history (employee_id, old_salary, new_salary, changed_on) VALUES (:old.employee_id, :old.salary, :new.salary, CURRENT_TIMESTAMP);In this example, the trigger is named 'trg_salary_history', it is executed after an update of the 'salary' column in the 'employees' table, and it is a row-level trigger, which is specified by the 'FOR EACH ROW' clause. When the trigger executes, it inserts a new row in the 'employees_salary_history' table with the employee_id, old_salary, new_salary, and the change timestamp.
Modifying and Deleting SQL Triggers
Once you have created a trigger, you may need to modify or delete it for various reasons such as fixing issues or removing redundant functionality. This section will detail altering and dropping SQL triggers.Altering an SQL Trigger Example
To modify a trigger, use the 'ALTER TRIGGER' syntax. Let's assume that you want to modify the trigger 'trg_salary_history' to record the user_id of the person who made the update to the employee's salary. To do this, you need to add a new column 'updated_by' in the 'employees_salary_history' table and change the trigger accordingly. Here's the SQL code to alter the trigger to include the 'updated_by' column:ALTER TRIGGER trg_salary_history AFTER UPDATE OF salary ON employees FOR EACH ROW INSERT INTO employees_salary_history (employee_id, old_salary, new_salary, changed_on, updated_by) VALUES (:old.employee_id, :old.salary, :new.salary, CURRENT_TIMESTAMP, USER);This code modifies the 'trg_salary_history' trigger by adding 'updated_by' to the INSERT statement in the trigger body.
Dropping an SQL Trigger Example
To delete a trigger, use the 'DROP TRIGGER' syntax. If you determine that the trigger 'trg_salary_history' is no longer necessary and you want to delete it from the database, you can use the following SQL code:DROP TRIGGER trg_salary_history;
This code removes the 'trg_salary_history' trigger from the database. Note that dropping a trigger does not affect the table or the data in the table, it only removes the trigger functionality.
SQL Triggers vs Functions
SQL triggers and functions both serve specific purposes and are important components of database management systems. However, they differ in terms of functionality, purpose, and usage:SQL Triggers:
- Execute automatically when a specified event occurs (e.g., INSERT, UPDATE, or DELETE).
- Associated with a specific table or view.
- Primarily used for maintaining data integrity, enforcing business rules, and auditing data changes.
- Execute when called explicitly like a regular function in a programming language.
- Return a single value or a table.
- Used for performing calculations, parsing, or data manipulation tasks on input data and returning the result.
When to Use Triggers and When to Use Functions
It's crucial to determine the appropriate usage scenario to make the most of SQL triggers and functions. Here are some general guidelines on when to use each:- Use Triggers: Choose triggers when you want to automate tasks in response to data manipulation events, perform cross-table consistency checks, or maintain historical records.
- Use Functions: Consider functions when you need to encapsulate reusable logic, perform complex calculations, or manipulate data without triggering an event. Functions should be used when logic requires flexibility and doesn't rely on specific table events.
Performance Considerations
When comparing the performance aspects of SQL triggers and functions, certain factors must be considered:- Triggers: Since triggers execute automatically, they may introduce processing overhead and slow down data manipulation operations. Overuse of triggers or implementing complex logic within triggers can negatively impact database performance.
- Functions: Functions can be optimized for performance as they are explicitly called, and their execution time is generally faster. However, poorly written functions can also impact the system's overall performance if they are resource-intensive or called frequently within data manipulation operations.
Recommendations for Using Triggers and Functions
To make the most of SQL triggers and functions, adhere to these best practices:- Triggers:
- Avoid using excessive triggers, as this may hinder database performance.
- Keep trigger logic simple, clean, and focused on a single purpose.
- Use triggers mainly for enforcing data integrity and consistency between related tables.
- Functions:
- Optimize functions for performance by minimizing resource consumption and avoiding nested function calls.
- Encapsulate complex computation and data manipulation logic within functions for better code organization and reusability.
- Make use of built-in functions whenever possible, as they are typically more efficient than user-defined functions.
Working with SQL Triggers Inserted Tables
Inserted Table is a temporary, in-memory table created by the database management system (DBMS) whenever a trigger is executed due to an INSERT, UPDATE, or DELETE event. Inserted tables hold the new updated values for data manipulation operations and can be accessed by the trigger during its execution to reference or compare the data.
Purpose of the Inserted Table
Inserted tables serve various purposes in the context of SQL triggers, including:- Storing new values for inserted or updated rows, allowing the trigger to reference or compare these values.
- Facilitating the implementation of business logic, constraint enforcement, and auditing features by providing a means to examine the changes being made.
- Enhancing data integrity by enabling the trigger to detect discrepancies between the old and new data values and apply appropriate actions before committing the changes.
How SQL Triggers Inserted Table is Used
When a data manipulation event occurs, the DBMS creates an inserted table and fills it with the updated row(s) involved in the triggering event. The inserted table can then be accessed by the trigger through a special "inserted" keyword. This allows the trigger to manipulate or compare the new data with the old data before either committing or rolling back the changes. In the case of:- INSERT operations: The inserted table contains all the newly added rows.
- UPDATE operations: The inserted table contains the updated rows with their new values.
- DELETE operations: The inserted table is not used, as the deleted rows are stored in another temporary table called the "deleted" table.
Practical Examples with SQL Triggers Inserted Tables
Here are some examples of SQL Triggers being used.
Example: Logging Changes to a Table
Let's consider a scenario where you want to log changes made to the employees table, recording the updated salary for each affected employee. To achieve this, create a trigger that uses the inserted table to extract the new salary values and store them in a separate audit table.CREATE TRIGGER trg_salary_log AFTER UPDATE OF salary ON employees FOR EACH ROW BEGIN INSERT INTO salary_audit (employee_id, old_salary, new_salary, modified_at) VALUES (:old.employee_id, :old.salary, :new.salary, CURRENT_TIMESTAMP); END;In this example, the trigger trg_salary_log is activated after the salary column in the employees table is updated. It then inserts a new row into the salary_audit table, capturing the employee_id, old_salary, new_salary, and modification timestamp.
Example: Ensuring Data Integrity with SQL Triggers Inserted
Consider a scenario where you have a table named "orders" and a table named "products", and you want to ensure that an order can only be placed for available products with sufficient stock. To achieve this, a trigger can be created that uses the inserted table to compare the requested order quantities against the available stock in the products table.CREATE TRIGGER trg_validate_order BEFORE INSERT ON orders FOR EACH ROW BEGIN DECLARE @stock INT; SELECT stock INTO @stock FROM products WHERE product_id = :new.product_id; IF @stock < :new.quantity THEN ROLLBACK; -- If the requested quantity is more than available stock, roll back the transaction RETURN; END IF; END;In this example, the trg_validate_order trigger is activated before a new row is inserted into the orders table. It checks the requested order quantity against the available stock using the inserted table values. If the requested quantity exceeds the available stock, the transaction is rolled back, ensuring data integrity in the database.
SQL Triggers - Key takeaways
SQL Triggers: Named database objects automatically executing actions (INSERT, UPDATE, DELETE, TRUNCATE) when an event occurs on a specified table or view, maintaining data integrity within databases.
Basic Concepts of SQL Triggers: Trigger Event (e.g., INSERT, UPDATE, DELETE), Trigger Action (modifications to tables), Triggering Event (data manipulation operations), Triggering Table (table being modified).
SQL Triggers Types: Before Triggers (before the triggering event), After Triggers (after the triggering event), Row-Level Triggers (executed for each row affected), Statement-Level Triggers (executed once per event).
Difference between SQL Triggers and Functions: Triggers are automatically executed in response to data manipulation events, while Functions are explicitly called to return values. Triggers enforce data integrity, whereas Functions perform calculations and data manipulation.
SQL Triggers Inserted: Temporary in-memory table created during trigger execution, enabling the trigger to reference and compare new values for data manipulation operations, ensuring data integrity.
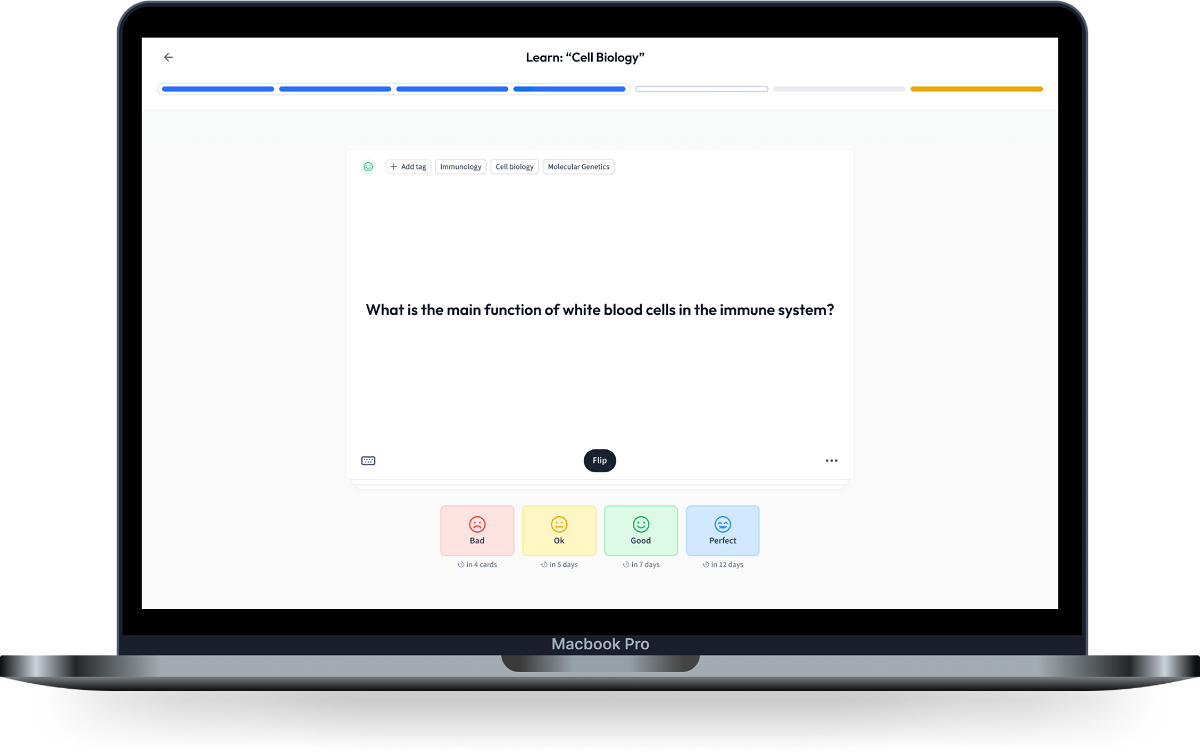
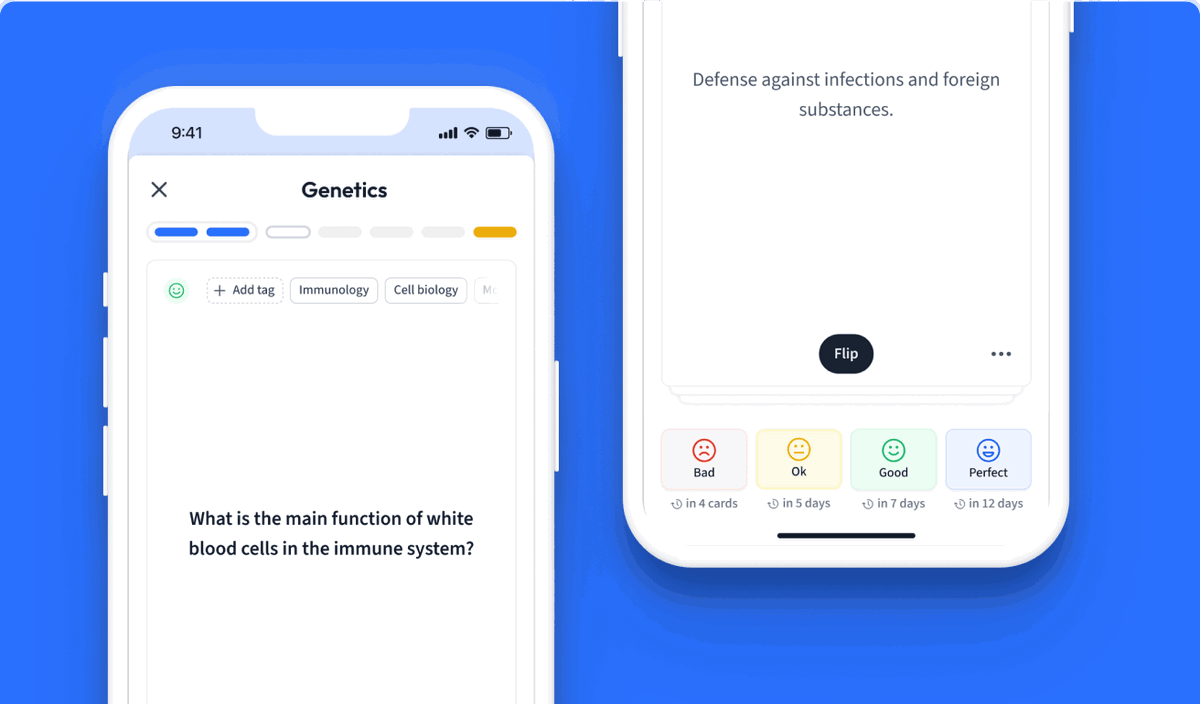
Learn with 59 SQL Triggers flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about SQL Triggers
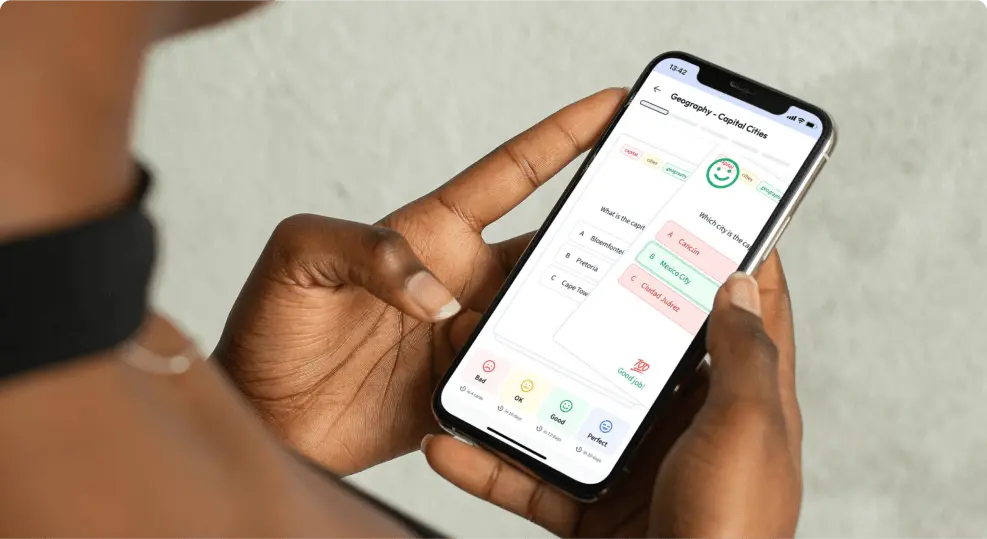
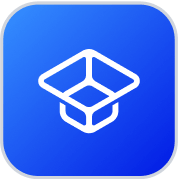
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more